Difference Between BufferedReader and FileReader
BufferedReader:
To read data from a specified character stream, two classes are used: buffered readers and file readers. Both of them have advantages and disadvantages. Although how they operate is the main distinction, we will also talk about the other specifics.
The BufferedReader is used to read the data from the text of a character input. The readline() method is used to read the data by the line.
In the BufferedReader, the buffer refers to the small portion of the memory of the device to store the data temporarily. The Buffer uses the RAM(Random access Memory) to store the temporarily, so it fast to store and retrieve the data from the BufferReader.
Constructors:
- BufferedReader(Reader in):
The BufferedReader(Reader in) constructor is used to create a input buffer to read the data from the stream.
- BufferedReader(Reader in, int size):
In BufferedReader(Reader in, int size) constructor, two parameters are required:
First: A Reader for reading data from the input stream
The input buffer's size comes in second. With the specified input buffer size, it generates a new BufferedReader.
Methods:
- close():
The close() method closes the stream and release the resource.
- mark():
The mark() method is used to mark current position.
- markSupported():
The markSupported() return true if the stream support the mark() method.
- read():
The read() method reads single character.
- readLine():
The readLine() method read the line from the text.
- ready():
The ready() method return true if the stream is ready to read.
- reset():
The reset() method is used to reset the stream to a recent point or mark.
- skip():
The skip() method is used to skip the characters.
Example program:
// example program for the Buffer reader
/* importing the packages */
import java. io.*;
class Buffer
{
// main method
public static void main(String args[])
{
// creating the object for File reader and Buffered reader
FileReader f = new FileReader(“F1.txt”);
BufferedReader b = new BufferedReader(f);
char c[] = new char[50];
// checking whether the stream is mark supported or not.
if(b.markSupported())
{
System.out.println(“The Mark() method is Supported”);
b.mark(100);
}
/* let us assume that the File F1 contains data like:
Hi there, This is Raman
Hey this is Bheem*/
// skipping the characters
b.skip(8);
// checking if the stream is ready or not.
if(b.ready())
{
// using the readLine method
System.out.println(b.readLine());
// using the read method
b.read(c);
for(int i=0;i<50;i++)
{
System.out.println(c[i]);
}
System.out.println();
// using the reset method.
b.reset();
for(int i=0;i<8;i++)
{
System.out.print((char)b.read());
}
}
}
}
Output:
Mark method is supported
This is Raman
Hey this is Bheem
Hi there,
In the above program, the file “F1.text” is used to read the data. To successful execution of the program the system should have the file named F1.
FileReader():
The java.io package contains a class called FileReader that can be used to read a stream of characters from files. For decoding from bytes to characters, this class employs either the provided charset or the platform's default charset.
Charset: Methods for creating encoders and decoders as well as for regaining multiple names combined with the charset are defined by the Charset class.
Default Charset: During implicit computer start up, the default charset is determined. It is based on the operating system's underlying locale and charset.
The Hierarchical Flow of the FileReader class is depicted in the image below
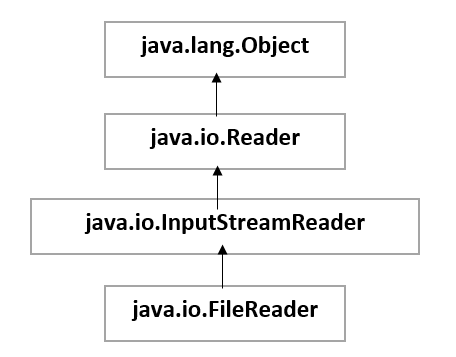
Constructors:
- FileReader(File f):
The FileReader(File f) constructor is used to generate a new FileReader to read a file.
- FileReader(FileDescriptor fd):
The FileReader(FileDescriptor fd) constructor is used to generate a new FileReader with FileDescriptor to read a file.
- FileReader(File f, Charset cs):
The FileReader(File f, Charset cs) constructor is used to create a FileReader to read a file with a given Charset
- FileReader(String file name):
The FileReader(String file name) constructor is used to create a new FileReader to read a file with a File name.
- FileReader(String file name, Charset cs):
The FileReader(String file name, Charset cs) constructor is used to create a new FileReader to read a file with given charset.
Methods:
- read():
The read() method reads only a single character or returns -1 if the stream is ended.
- read(char[] cb, int offset, int len) :
The read(char[] cb, int offset, int len) method reads and stores a stream of characters in the designated Character Buffer. Length is the total number of characters to be read, and Offset is the position from which it begins reading. It either passes many read characters or returns -1 if the stream is stopped.
- ready():
The ready() method return true if the stream is ready to read .(The stream is ready when the file is not blank.)
- getEncoding():
The name of the character encoding that the stream is using is returned by the getEncoding() method.
- close():
The close() method closes the stream and release the resource.
Example Program:
// importing the packages
import java. io.*;
class FileReader
{
public static void main(String args[])
{
try
{
// creating a object for FileReader with file
FileReader f = new FileReader(“F1.txt”);
System.out.println(“Reading char by char:”);
int i;
// reading the file by char by char using read() method
while((i=f.read())!=-1)
{
System.out.println((char)i);
}
System.out.println(“Reading with using array”);
// reading the file using char array
char[] ca = new char[10];
f.read(ca);
System.out.println(ca);
f.close();
System.out.println(“Succesfully Closed FileReader”);
}
catch(Exception e)
{
System.out.println(e);
}
}
}
Output:
Reading char by char:
Hello there
Reading using array:
Hello there
Successfully closed FileReader.
In the above program, the file “F1.text” is used to read the data. To successful execution of the program the system should have the file named F1.
Difference Between BufferedReader and FileReader.
The following important parameters are used to illustrate and discuss the differences between BufferedReader and FileReader:
- Usage
- Efficiency
- Speed
- Reading Lines.
Based on | BufferedReader | FileReader |
Usage | The BufferedReader can able to read any type of character input stream. | The FileReader can only for reading files. |
Efficiency | The BufferReader uses buffer to read the character. | The FileReader directly reads a file from hard drive. |
Speed | The BufferReader is Fast. | The FileReader is Slow. |
Reading Lines | The BufferReader can read a line and a character at a time. | The FileReader can only read character at a time. |
Usage:
While BufferedReader is not restricted to merely reading files, FileReader is used to read a file from a disc drive. Any character stream can be used to read data from it. BufferedReader can able to read any character from file, because it accepts any type of Reader(StringReader, FileReader, etc.).
FileReader, on the other hand, can only read characters from files. Typically, while reading characters from files, we wrap a FileReader in a BufferedReader.
Efficiency:
Performance-wise, BufferedReader outperforms FileReader by a wide margin. The data is immediately read from a character stream that comes from a file via FileReader. It accesses the disc drive directly each time a character is read, which makes it incredibly inefficient because it takes some time for the disc drive to position the read head properly.
BufferedReader improves the efficiency of the stream by creating the input buffer from the hard drive to read the file.Although it can be changed, the default buffer size is 8Kb(which is sufficient in most situations). BufferedReader reads large amounts of data at once and stores it in the buffer memory that is produced. When there is no data in the buffer, it sends a corresponding read request to the underlying character stream and fills the newly generated buffer with a tonne of data.
Speed:
BufferedReader is substantially faster than FileReader since it internally uses a buffer. BufferReader is quicker because it doesn't constantly access the hard disc like FileReader does.
Reading Lines:
Only the BufferedReader offers a readLine() method that reads an entire line at once. In most circumstances, the reading the line at a time is better than reading a character at a time. The supplied Reader(in this instance, FileReader) simply reads the characters and saves them in the buffer. It is much faster than the FileReader#read() technique since it saves so much time. Be aware that BufferedReader can only read an entire line at once since it uses a buffer memory. This allows it to store a line's characters in the buffer and read the entire line at once from the buffer.
Summary:
The BufferedReader and FileReader are which are used to read the data from a specified input stream. The BufferedReader is used to read the data from the text of a character input. The FileReader is used to read a stream of characters from files and for decoding from bytes to characters, the FileReader class employs either the provided charset or the platform's default charset.
The Difference between BufferedReader and FileReader is based upon the factors like Usage ,Efficiency, Speed and Reading lines.