Java For Keyword
For as a keyword in java:
When we need to run a set of statements repeatedly in Java, we use loops. The Java for loop offers a clear way to express the loop structure. For statement condenses the initialization, condition, and increment/decrement into a single line, making the looping structure shorter and simpler to troubleshoot.
Flowchart of For Loop
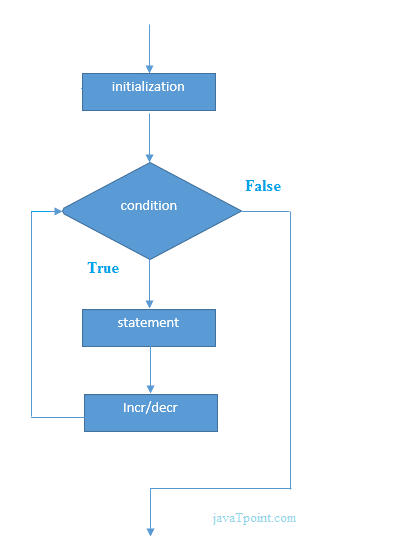
A section of the programming is repeatedly iterated using the Java for loop. Use of a for loop is advised if the number of iterations is fixed.
In Java, there are 3 different forms of for loops.
- Simple for Loop
- For-eachor Enhanced for Loop
- Labeled for Loop
Simple for loop
A straightforward for loop is equivalent to C/C++. We can set the variable to a value.
check the increment/decrement value and the condition. There are four sections to it:
- Initializing in for loop:
Step 1: When the loop begins, the first condition is the one that is run once. Here, we have the option of implementing the variable or using one that has already been initialised. It's an optional requirement.
- Condition in for loop:
Step 2: Every time the loop's state is tested, the second condition is what is carried out. Until the condition is false, execution keeps going. Either true or false must be returned as a boolean value. It's an optional requirement.
- Increment or decrement step in for loop:
Step 3: The variable value is increased or decreased as a result. It's an optional requirement.
- Printing Statement:
Every time the second condition is met, the loop's statement is carried out.
Syntax of for loop:
for (initialization; condition; increment/decrement) {
//output or the statements that should be repeated to print or execute
}
Example for a simple for loop Keyword:
ForExample.java
//Program in java to illustrate the example of for loop keyword
//mathamatic tables of 1 which is executed or printed
public class for_Ex {
public static void main (String [] s) {
//Java for loop
for (int i=1; i<=10; i++) {
System.out.println(i);
}
}
}
Output:
1
2
3
4
5
6
7
8
9
10
Nested For Loop in Java
Nested for loops are for loops that are contained within other loops. Every time the outer loop runs, the inner loop also does.
Example:
Nested For Example in java programming language
public class Nested_For_Ex {
public static void main (String [] s) {
//loop of a
for (int a=1; a<=3; a++) {
//loop of b
for (int b=1; b<=3; b++) {
System.out.println(a+" "+b);
}//end of a
}//end of b
}
}
Output:
1 1
1 2
1 3
2 1
2 2
2 3
3 1
3 2
3 3
Pyramid Examples of java programming language using for loop:
Exmaple 1:
public class PyramidEx1{
public static void main (String [] s) {
for (int a=1; a<=5; a++) {
for (int b=1; b<=a; b++) {
System.out.print("* ");
}
System.out.println() ;//new line
}
}
}
Output:
*
* *
* * *
* * * *
* * * * *
Example 2:
public class PyramidEx2 {
public static void main (String [] s) {
int num=6;
for (int x=1; x<=num; x++) {
for (int y=num; y>=x; y--) {
System.out.print("* ");
}
System.out.println() ;//printing or executing a new line
}
}
}
Output:
* * * * * *
* * * * *
* * * *
* * *
* *
*
For each Loop in Java
Java's for-each loop is used to iterate across an array or collection. Because we don't have to use subscript notation or increment value, it is simpler to use than a standard for loop.
Instead, then using an index, it operates based on elements. In the specified variable, each element is returned one by one.
Syntax:
for (data_type variable: array_name) {
//output printing statements
}
Example:
//array elements of the program
public class ForEachEx {
public static void main (String[] s) {
//initializing an array statically
int ele[] = {12,23,44,56,78};
//executing the array by implementing the for-each loop
for (int a: ele) {
System.out.println(a);
}
}
}
Output of the program:
12
23
44
56
78
Java Labeled for Loop
Each Java for loop can have a name. We utilize label before the for loop to do this. We may break/continue a particular for loop, which is helpful when utilizing nested for loops.
Syntax:
labelname:
for (initialization; condition; increment/decrement) {
//executing statements
}
Example:
public class LabeledforEx {
public static void main (String[] s) {
gg:
for (int a=1; a<=3; a++) {
hh:
for (int b=1; b<=3; b++) {
if(a==2&&b==2) {
break gg;
}
System.out.println(a+" "+b);
}
}
}
}
Output:
1 1
1 2
1 3
2 1
Infinity For Loop in Java
The for loop becomes infinite if two semicolons are used, or ;;
Syntax:
for (;;)
{
// statements
}
Example:
public class ForEx {
public static void main (String [] s) {
//no implemetation of condition in for loop
for (; ;) {
System.out.println("loop is continuous");
}
}
}
Output of the program:
loop is continuous
loop is continuous
loop is continuous
loop is continuous
loop is continuous