Adapter class in Java
By using the adapter classes, we can implement Listener interfaces. With the help of adapter classes, we can save code as it provides all implementation methods of listener interfaces
Advantages of Adapter classes
- Adapter class offers a means of incorporating similar patterns into the class.
- It offers a pluggable development kit for applications.
- By using the adapter class, it makes the class more versatile.
- Adapter classes makes easier for unrelated classes to collaborate.
- It offers diverse approaches to using classes.
- It makes lessons more transparent.
To use the adapter class,mustd to import the following packages
- java.awt.event
- java.awt.dnd
- javax.swing.event
java.awt.event adapter class
Adapter class | Listener Interface |
Mouse Adapter | Mouse Listener |
Key Adapter | Key Listener |
Window Adapter | Window Listener |
MouseMotionAdapter | MouseMotionListener |
ContainerAdapter | ContainerListener |
ComponentAdapter | ComponentListener |
java.awt.dnd adapter class
Adapter class | Listener Interface |
DragSourceAdapter | DragSource Listener |
DragTargetAdapter | DragTarget Listener |
java.swing.event adapter class
Adapter class | Listener Interface |
Mouse Input Adapter | Mouse Input Listener |
Internal Frame Adapter | Internal Frame Listener |
Program for Java Mouse Adapter
MouseAdapter1.java
import java.awt.*;
import java.awt.event.*;
public class MouseAdapter1 extends MouseAdapter{
Frame f1; // Creating a frame
// Default constructor
MouseAdapter1(){
f1=new Frame("Mouse Adapter"); // Setting the name of the frame as MouseAdapter
f1.addMouseListener(this);
f1.setSize(400,400); // Setting the size of frame
f1.setLayout(null);
f1.setVisible(true); // Giving visibility to the frame
// This inner class is used to close the window
f1.addWindowListener (new WindowAdapter()
{
public void windowClosing (WindowEvent e1) {
f1.dispose();
}
});
}
public void mouseClicked(MouseEvent e) {
Graphics g1=f1.getGraphics();
g1.setColor(Color.BLACK);
g1.fillOval (e.getX(), e.getY(), 20, 15);
}
public static void main(String[] args) {
new MouseAdapter1();
}
}
Output

Java Window Adapter Program
This program is used to create windows using awt packages
Adapter.java
// Necessary packages are imported
import java.awt.*;
import java.awt.event.*;
public class Adapter {
// Creating a Frame f1
Frame f1;
// default class constructor
Adapter() {
// creating a frame with the title
f1 = new Frame ("Adapter Windows");
// overriding the window closing() method
f1.addWindowListener (new WindowAdapter() {
public void windowClosing (WindowEvent e1) {
f1.dispose();
}
});
// setting the size of the frame
f1.setSize (350, 450);
// setting the size of the frame
f1.setLayout (null);
f1.setVisible (true);
}
// main method
public static void main(String[] args) {
new Adapter();
// Default constructor
// Name of the constructor should be same as class name
} // main method for the program
} //Adapter
Output
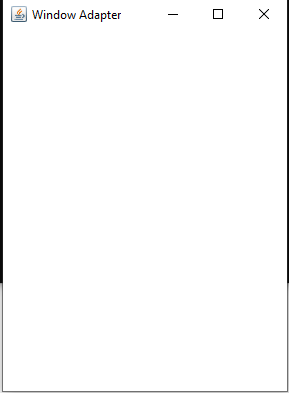
Java MouseMotionAdapter Program
MouseMotionAdapter1.java
import java.awt.*;
import java.awt.event.*;
public class MouseMotionAdapter1 extends MouseMotionAdapter {
// Creating a frame f1
Frame f1;
MouseMotionAdapter1() {
f1 = new Frame ("Adapter Program for mouse motion");
f1.addMouseMotionListener (this);
// Setting the size to 300 X 300 for the frame
f1.setSize (300, 300);
f1.setLayout (null);
f1.setVisible (true);
f1.addWindowListener (new WindowAdapter() {
public void windowClosing (WindowEvent e1) {
f1.dispose();
}
});
}
public void mouseDragged (MouseEvent e1) {
// Creating object for Graphics class to draw in the frame
Graphics g1 = f1.getGraphics();
// Setting the color to red
g1.setColor (Color.RED);
// Drawing oval shaped to draw the described message
g1.fillOval (e1.getX(), e1.getY(), 10, 10);
}
// Main method for the program
public static void main(String[] args) {
new MouseMotionAdapter1();
// Default constructor
//Constructor name should be same as class name i.e., MouseMotionAdapter1
}
}
Output

Java KeyAdapter Program
KeyAdapter1.java
//Importing the required packages
import java.awt.*;
import java.awt.event.*;
public class KeyAdapter1 extends KeyAdapter {
// Creating label and textarea component variables
Label l1;
TextArea area1;
Frame f1;
// class constructor
KeyAdapter1() {
// creating the Frame with the title
f1 = new Frame ("Adapter for Key ");
// creating the Label
l1 = new Label();
// setting the location of the label
l1.setBounds (20, 50, 200, 20);
// creating the text area
area1 = new TextArea();
area1.setBounds (20, 80, 300, 300);
area1.addKeyListener(this);
// Adding the label and text area to the frame by using the add method
f1.add(l1);
f1.add(area1);
// setting the size
f1.setSize (400, 400);
//layout of the frame
f1.setLayout (null);
//visibility of the frame
f1.setVisible (true);
f1.addWindowListener (new WindowAdapter() {
public void windowClosing (WindowEvent e) {
f1.dispose();
}
});
}
public void keyReleased (KeyEvent e) {
String text1 = area1.getText();
// splitting the given String
String words1[] = text1.split ("\\s");
l1.setText ("Words: " + words1.length + " Characters:" + text1.length());
}
// main method for the program
public static void main(String[] args) {
new KeyAdapter1();
}
}
Output
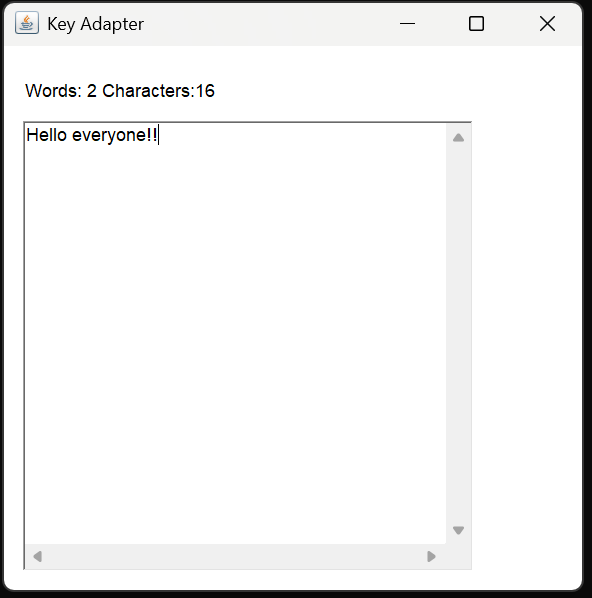