URLConnection class in Java
A communication channel between the URL and the program is represented by the Java URLConnection class. It may be utilized to read from and write to the given resource the URL refers to.
What is the URL?
- The term "URL" stands for "Uniform Resource Locator." An URL is a type of string that helps find a site on the Internet (WWW).
- A URL has two components:
1.The protocol required to access the resource.
2. Where the resource is located.
Features of the URLConnection class
- A class called URLConnection is abstract. The two subclasses, HttpURLConnection and JarURLConnection, are responsible for establishing connections between the client Java programme and URL resources found on the internet.
- The user can read from and write to any resource that a URL object references using the URLConnection class.
- Once a connection has been made and a URLConnection object has been created in the Java application, we may use it to read, write, or obtain further data, such as content length.
Constructors
1. secure URL connection (URL url):- It builds a connection to the requested URL.
URLConnection Class Methods
void addRequestProperty(String key, String value) | A key-value pair is added to the generic request property. |
void connect() | If a connection has not yet been made, it establishes one and creates a communications channel to the resource this URL belongs to. |
boolean getAllowUserInteraction() | It returns the value of the object's allowUserInteraction property. |
int getConnectionTimeout() | The connect timeout setting is returned. |
Object getContent() | It obtains the information from the URL connection. |
Object getContent(Class[] classes) | It gets the data from the URL connection. |
String getContentEncoding() | The value of the header field for content-encoding is returned. |
int getContentLength() | The content-length header field's value is what is returned. |
long getContentLengthLong() | The content-length header field's value, expressed as long, is returned. |
String getContentType() | The date header field's value is what is returned. |
long getDate() | The date header field's value is what is returned. |
static boolean getDefaultAllowUserInteraction() | The allowUserInteraction field's default value is returned. |
boolean getDefaultUseCaches() | It provides the useCaches flag of an URLConnection's default value. |
boolean getDoInput() | The doInput flag of the URLConnection is returned along with its value. |
long getExpiration() | The value of the expired header files is returned. |
static FileNameMap getFilenameMap() | The filename map is loaded from a data file. |
String getHeaderField(int n) | The nth header field's value is returned. |
String getHeaderField(String name) | The name of the header field's value is returned. |
long getHeaderFieldDate(String name, long Default) | It displays the value, encoded as a number, of the provided field. |
int getHeaderFieldInt(String name, int Default) | It provides a numerical representation of the specified field's value. |
String getHeaderFieldKey(int n) | The key for the nth header field is returned. |
long getHeaderFieldLong(String name, long Default) | It gives the specified field's value, decoded as a number. |
Map<String, List<String>> getHeaderFields() | The unmodifiable Map of the header field is returned. |
long getIfModifiedSince() | The ifModifiedSince field of the object's value is what is returned. |
InputStream getInputStream() | An input stream that reads from the open condition is returned. |
long getLastModified() | The last-modified header field's value is that which is returned. |
OutputStream getOutputStream() | An output stream that writes to the connection is returned. |
Permission getPermission() | It returns back a permission object that indicates the authorization required to establish the connection represented by the object |
int getReadTimeout() | It provides the read timeout setting back. |
Map<String, List<String>> getRequestProperties() | It gives back the connection's value for the named general request property. |
URL getURL() | The URLConnection's URL field's value is what it returns. |
boolean getUseCaches() | The value of the useCaches field of the URLConnection is returned. |
Static String guessContentTypeFromName(String fname) | It attempts to determine the content type of an object based on the URL's specified to the file component. |
static String guessContentTypeFromStream(InputStream is) | It makes an effort to determine the kind of stream from the first characters of the input stream. |
void setAllowUserInteraction(boolean allowuserinteraction) | The allowUserInteraction field of this URLConnection is given a new value. |
static void setContentHandlerFactory(ContentHandlerFactory fac) | An application's ContentHandlerFactory is configured by it. |
static void setDefaultAllowUserInteraction(boolean defaultallowuserinteraction) | For all the next URLConnection objects, it changes the allowUserInteraction field's default value to the supplied value. |
void steDafaultUseCaches(boolean defaultusecaches) | It changes the useCaches field's default value to the specified value. |
void setDoInput(boolean doinput) | For this URLConnection, it sets the supplied value for the doInput field's value. |
void setDoOutput(boolean dooutput) | The doOutput field for the URLConnection is set to the desired value. |
How to obtain a URLConnection class object?
The object of the class URLConnection is returned by the openConnection() function of the URL class.
Syntax:
public URLConnection openConnection()throws IOException{}
Displaying a webpage's source code using the URLProgram class
Numerous methods are available from the URLProgram class. Using the getInputStream() function, we can show every information on a website. It gives back all of the supplied URL's contents in a stream that may be read and shown.
URLProgram.java
import java.io.*;
import java.net.*;
public class URLProgram {
public static void main(String[] args){
try{
URL url=new URL("http://www.microsoft.com");
URLConnection urlcon=url.openConnection();
InputStream stream=urlcon.getInputStream();
int i;
while((i=stream.read())!=-1){
System.out.print((char)i);
}
}catch(Exception e){System.out.println(e);}
}
}
Output:
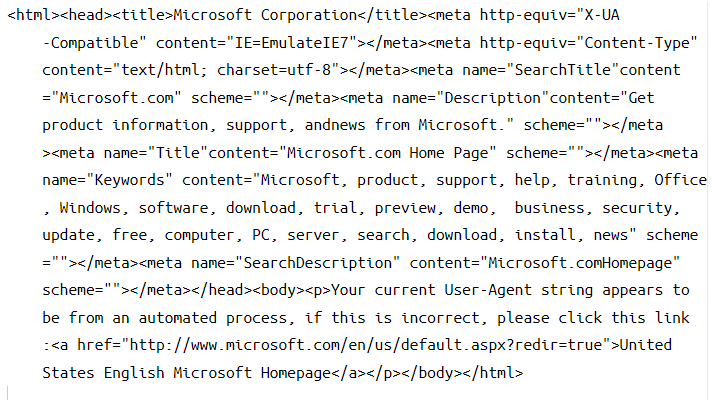