How to add 24 Hours to Date in Java?
In this tutorial, we will learn how to add 24 hours to the local or current date in Java language. We will begin our topic with basic concepts and would reach a java code to finally understand the proper way to accomplish this task.
To add hours to the current date, there exists a method known as the plus hours()method, which belongs to the java class called LocalDate class.
This method is encompassed in Java version 8throughDataTime API.
Users can easily increase the months as per their requirements.
To be able to use this class, the needs need to import a package known as java.time package.
Syntax:
LocalTimet2 = t1.plusHours(hours_to_be_increased);
Understanding the above syntax
- LocalTime- It is a variable to store the local or the current time.
- .plusHours () – it is a method that adds the required no. of hours to the current time.
- LocalTime.now– It fetches the local or the current time.
- hours_to_be_increased– It refers to the number of hours that is to be added to the current time.
Let us see some of the examples to understand the working of the above method.
Examples:
- Input - 15:41:39.843631 Output - 15:41:39.843631 [ After adding 24 hours]
- Input - 14:41:39.843631 Output - 14:41:39.843631 [ After adding 24 hours]
- Input - 12:41:39.843631 Output - 12:41:39.843631 [ After adding 24 hours]
- Input - 10:41:39.843631 Output - 10:41:39.843631 [ After adding 24 hours]
- Input - 09:41:39.843631 Output - 09:41:39.843631 [ After adding 24 hours]
Now, let us see a java code to understand the topic.
Import the following package for the Calendar class in Java.
import java.util. time;
Implementation 1:
import java.time.*;
public class addingHours {
public static void main(String[] args)
{
LocalTime time1 = LocalTime.now();
System.out.println("Current Time : " + time1);
// adding 24 hours to the current time
LocalTime newTime1 = time1.plusHours(24);
System.out.println();
System.out.println("Time after 24 hours : " + newTime1);
System.out.println();
}
}
Output:
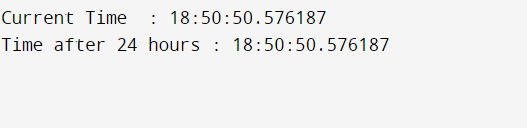
Explanation: In the above code, we have added 24 hours to the current time. Here, the output is the same as the input since the time will be the same after 24 hours.
The method LocalTime.now() is used to fetch the current time.
The method time1.plusHours() is used to increase the time by 24 hours.
Summary:
We learned how to add 24 hours to the current date in Java language. It was interesting to understand we can accomplish this task simply by taking a method called plus hours () into account and providing 24 as the parameter.
However, we can increase any number of hours by giving that number as a parameter in the method. We can also decrease the no. of hours by giving a negative integer as a parameter to the plus hours () method.