How to initialize string array in Java
In this tutorial, we will learn how the string array is initialized in java.
The initialization of string array in the java by using the NEW (it creates the object for the class)operator with an array initialize.
The string array in java is used to store the fixed number of strings.
The java main method also contains the String argument as:
Public static void main (String args[])
The initialization of string array to empty?
We know that the array size can't be changed.
The syntax for empty array:
private static final String[] EMPTY_ARRAY = new String[0];
The java string array is the collection of objects in the array.
In java, arrays are written dynamically. For creating an array, we use two methods. The first one is creating without the size, and the second one is creating with a size.
The syntax for creating the sized array:
String [] s1 = new String [‘Apple’, ‘Banana’, ‘Mango’];
We may perform different operations on String array, i.e., insertion, deletion, updating, sorting, searching etc...
Let's see the syntax for creating a string array without size.
String [] S1;
We can also create any string with another method.
String s1[];
But above one is the standard way. In the above syntax, strings are null values, and the s1 value, which we declared String [3], places three null values.
The compiler will take the default value stored as the null value.
As we all know that the array size is fixed. But we can change the size of the array after being created dynamically. The ArrayList method changes the size by increasing or decreasing it.
- Let's see the syntax for ArrayList:
List<String> ArrayList = new ArrayList<>(Arrays.asList(“apple”, “banana”, “mango”)); System.out.println(ArrayList);
- We can create a string array using an instance variable. The following syntax will create a new string with the default value.
String [] array = (String []) Array.newInstance(String.class,2); System.out.println(Arrays.toString(arr));
Examples
Let's see a simple program for initializing the String Array. Declaring a variable of String [] and initializing the set of strings by using the assignment operator, we can assign the strings to the String [] class.
Example1:
import java.io.*;
public class Demo2
{
public static void main(String args[])
{
String letters[] = {"j", "a", "v", "a", "t" , "p" ,"o" , "i" , "n", "t"};
for(String letter: letters)
{
System.out.println(letter);
}
}
}
Output:
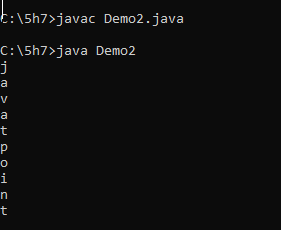
Hence this is a simple program for initializing the string array.
Example 2: Let's see another easiest method to declare the string array:
import java.io.*;
public class Demo2
{
public static void main(String args[])
{
String animals[] = new String[5];
animals[0] = "cat";
animals[1] = "dog";
animals[2] = "rabbit";
animals[3] = "lion";
animals[4] = "tiger";
for(String animal: animals)
{
System.out.println(animal);
}
}
}
Output:
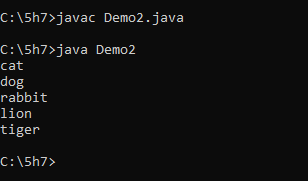
The above two programs will have the same values. But if you use the equals method, it will exit with false. Because equals methods don't see the content, it shows the reference based on the program.
Let's see how the string array will be converted into the String
The Arrays.toString() method will convert the String array to the string function. We can't directly implement it. We can use the string conversion to represent the string function.
Majorly there are many ways to initialize the string array. We will discuss a few of them.
Example 3:
Import java.io.*;
public class Demo2
{
public static void main(String args[])
{
String[] myArr;
myArr = new String[5];
myArr[0] = "First";
for (int i = 0; i < myArr.length; i++)
{
System.out.println(myArr[i]);
}
}
}
Output:
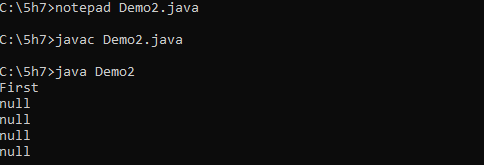
Overview of String Array in Java
- The java string array is the collection of objects in the array.
- In java, arrays are written dynamically. For creating an array, we use two methods. The first one is creating without the size, and the second one is creating with a size.
- The default string value is sored as null values.
- The string array in java is used to store the fixed number of strings.