Static vs Non-Static in Java
A static method can access and update the value of a static data member. The static keyword is mostly used in Java for memory management. The static keyword can be used with variables, methods, blocks, and nested classes. The static keyword refers to a class rather than a particular instance of the class. A static method belongs to a class but not to an instance of that class and so may be called without the class's instance or object. A static method always starts with a Static Keyword.
The following code is an example of a static method.
StaticExample.java
public class StaticExample {
//Static variable
static String name = "Sahil is the Writer of this Article";
static void display() //Static method
{
System.out.println("Hello Everyone");
}
public static void main(String[] args) {
display();
//calling the method without creating its object
System.out.println(name);
// calling the static variable
}
}
Output:
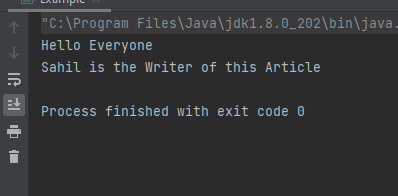
Every method in Java defaults to a non-static method if it doesn't have the static keyword before it. Non-static methods can access every static method and static variable without creating an instance of the object. Dynamic or runtime binding is utilized with non-static methods. We may override the non-static method, unlike the static method. Following is an example of a non-static method.
public class Example {
public void display() // non static method
{
System.out.println("This method is a non-static method with public as its access speciifer");
}
private void display2() // non static method
{
System.out.println("I hope you can now differentiate between Static and Non-Static Method");
}
String name = "Sahil Deshmukh"; // non static variable
static String Autor = "Name of the Author"; // static method
static void display1() // static method
{
System.out.println("Have a good day");
}
public static void main(String[] args) {
Example e = new Example();
System.out.println(Autor); // printing a static variable
System.out.println(e.name); // printing a non-static variable
System.out.println();
e.display();
e.display2();
display1();
}
}
Output:
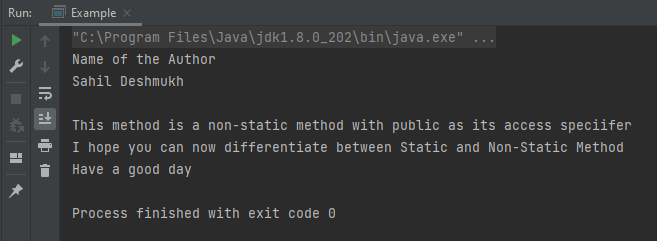
As you can observe, no instance was created to call a static method, whereas to call a non-static method first, we need to create a class instance and then call the particular method.
The following table summarizes the differences between the above methods
Static Method | Non-Static Method |
A static method belongs to a class but not to an instance of that class and may therefore be called without needing the class's instance or object. | Every method in Java defaults to a non-static method if it doesn't have the static keyword before it. Non-static methods may access every static method and static variable without using the class object. |
Only static data members and methods of other classes or the same class may be accessed by the static method; non-static methods or variables are not accessible. | The non-static method can access both static data members and static methods and non-static members and methods from the same or another class. |
Compile-time or early binding is used in the static technique. | Runtime or dynamic binding is used in the non-static technique. |
Because of early binding, the static method is not overridable. | Because of runtime binding, the non-static method can be altered. |
The static approach uses less memory for execution because memory allocation occurs just once because the static keyword provided a specific memory address in ram for that operation. | The non-static method consumes a lot of memory during execution since memory is allocated when the method is called, and memory is allocated every time the method is called. |
The static and non-static approaches were described in the preceding article, as it discussed the methods and their implementation. Let's take a closer look at static and non-static variables now.
A variable is a container that retains the value while a program, such as a Java application, is running. A variable is allocated with a data type. The term "variable" refers to a memory region's name. In Java, there are three types of variables:
- Static Variables
- Local Variables
- Instance Variables
Non-Static variables are a combination of local variables and instance variables. As a result, it's possible to divide Java variables into two categories:
Static Variables
When a variable is declared static, a single copy of it is made and shared among all objects in the class. Static variables, on the other hand, are effectively global variables. It's a class-specific variable, not an object-specific variable. All instances of the class share the static variable. A static variable may be accessed simply by the class name and does not need the use of an object. It improves the memory efficiency of your software (i.e., it saves memory).
// Example of static variable
public class Example1 {
static String name ="Sahil"; //Static Variable
public static void main(String[] args) {
System.out.println(name);
}
}
Output:
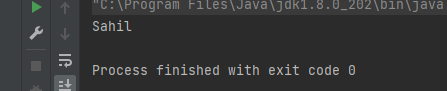
Local Variables
- A local variable is a variable declared within a block, method, or function.
- These variables are generated when the block is entered, or the function is called, and they are removed when the block or function call returns.
- These variables have a scope that is limited to the block in which they are defined, i.e., these variables can only be accessed within that block.
- The initialization of a local variable is required.
public class Example1 {
void author()
{
String name ="Sahil Deshmukh"; // local variable
System.out.println(name);
}
public static void main(String[] args) {
Example1 e = new Example1();
System.out.println("Name of the Author");
e.author();
}
}
Output:
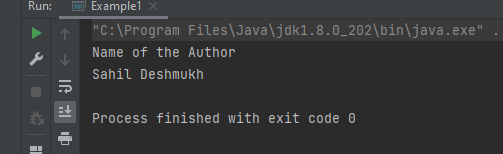
Instance Variables
- These are non-static variables defined outside of any method, constructor, or block of a class.
- When a class declares instance variables, these variables are produced when the class's object is formed and deleted when the object is destroyed. For instance, unlike local variables, variables can utilize access specifiers. The default access specifier will be utilized if no access specifier is provided. It is not necessary to initialize the instance variable. It has a value of 0 by default. Only by constructing objects can you access the Instance Variable.
- In contrast to local variables, we can utilize access specifiers on instance variables. The default access specifier will be utilized if no alternative access specifier is supplied.
- An instance variable does not need to be initialized. By default, the value is set to 0.
- Instance Variable can only be accessed by creating objects.
// Example of Instance variable
public class Example1 {
String name ="Sahil Deshmukh"; //instance variable
public static void main(String[] args) {
Example1 e = new Example1();
System.out.println("Name of the Author");
System.out.println(e.name);
}
}
Output:
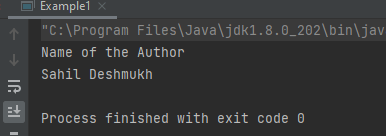
Local Vs. Instance Vs. Static Variable
The following table summarizes the difference between all these three variables
Local Variable | Instance Variable | Static Variable |
Within a procedure or a code block, a variable is defined. | At the class level, defined outside of a method. | At the class level, defined outside of a method. |
It can only be accessed in the method/code block where it was declared. | Is available at all times throughout the class. | Is available at all times throughout the class |
As long as the procedure is running, it stays in memory. | As long as the thing is remembered, it will remain in memory. | As long as the application is running, it stays in memory. |
There is no need for a unique keyword. | No particular keyword is required; however, any access specifier (private, protected, or public) can be supplied. Typically, the terms private and protected are employed. | It is necessary to use the static keyword. You can also specify any access specifier (private, protected, or public). The term "public" is frequently used. |
It must be initialized before it can be utilized. | Because it has a default value depending on its data type, it doesn't need to be initialized before use. | Because it has a default value depending on its data type, it doesn't need to be initialized before use. |