Java Program to generate binary numbers
A binary tree can create binary numbers ranging from 1 to n. Every node in a tree, the right, and left nodes, has two children, as is common knowledge. The root node in this binary tree will be 1. We will append 0 and 1 to the end of the root node, respectively, to acquire the left and right nodes of the root node, and so on.
Steps in generating binary numbers
- Make a String-type Queue.
- Set the value of a variable, say n, to 0.
- As the root node, push number one into the queue.
- Repeat steps 6 and 7 until the sum is less than n.
- Print the root element by selecting it from the queue.
- Send the element's right child, as well as its left child, to the queue.
- Add one more to the variable total.
Program to generate binary numbers using queues
BinaryUsingQueues.java
import java.util.*;
public class BinaryUsingQueues
{
private static void BinaryNumbersgenerator ( int n )
{
// it returns 0 if number is equal to zero
if ( n == 0 )
{
return;
}
// Creating queue of string datatype
Queue < String > q = new LinkedList < > ( ) ;
// Adding a base number to the string
q . add ( " 1 " ) ;
// t variable to store the value
int t = 0;
System.out.println("Binary number for "+n);
while (t < n)
{
String curr = q . poll ( ) ;
System . out. print ( curr + " " ) ;
q . add ( curr + "0" ) ;
q . add ( curr + "1" ) ;
t++;
}
System.out.println();
}
// Main section of the program where execution starts
public static void main(String args[])
{
int n1;
Scanner sc=new Scanner ( System . in ) ;
System.out.println("enter number");
n1 = sc . nextInt ( ) ;
BinaryNumbersgenerator ( n1 ) ;
int n2 ;
System.out.println("enter number");
n2 = sc . nextInt ( ) ;
BinaryNumbersgenerator ( n2 ) ;
}
}
Output
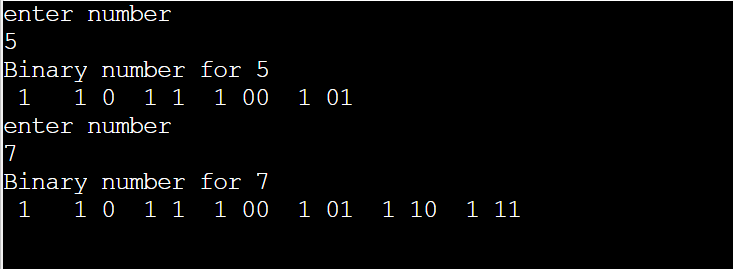
Let’s see another approach for the same.
BinaryNumbers2.java
import java. util . * ;
public class BinaryNumbers2
{
public static void main ( String args [ ] )
{
int n ;
Scanner sc=new Scanner ( System . in ) ;
System . out . println ( " Enter number " ) ;
n = sc . nextInt ( ) ;
Queue < String > que = new LinkedList <> ( ) ;
que . add ( " 1 " ) ;
while (n > 0)
{
String t = que . poll ( ) ;
System . out . print ( t + " " ) ;
que . add ( t + "0" ) ;
que . add ( t + " 1 ") ;
n-- ;
}
que . clear ( ) ;
}
}
Output

Binary Numbers using the itoa() method
The function changes an integer into a string with a null termination. Even negative numbers can be converted.
Procedure using the itoa() method
1. Initialize the value up to which binary numbers should be generated.
2. Divide the amount by 2 after calculating the modulo and storing the residual in an array.
3. Till the amount is equal to or less than 0, repeat the previous step.
4. To obtain the equivalent binary numbers, print the array starting at the end.
import java . io . * ;
import java . util . * ;
public class BinaryNumberUsingitoa
{
static String itoa ( int x , int base )
{
boolean neg = false ;
String s1 = "";
if (x == 0)
return "0";
neg = (x < 0);
if (neg)
x = -1 * x;
while (x != 0)
{
s1 = (x % base) + s1 ;
x = x / base ;
}
if ( neg )
s1 = "-" + s1 ;
return s1 ;
}
static void Generator ( int n )
{
System . out . print ( " 0 " ) ;
for (int j = 1; j <= n; j++)
{
String a1 = new String ( itoa ( j , 2 ) ) ;
System . out . print ( a1 . substring ( 0 , a1 . length ( ) ) + " " ) ;
}
}
public static void main ( String args [ ] )
{
Scanner sc = new Scanner ( System. in ) ;
int n1 = sc . nextInt ( ) ;
Generator ( n1 ) ;
}
}
Output
