Convert list to array Java
One of the popular collection interfaces for storing an ordered collection is the List. The List interface may contain repeating groups and preserves the insertion order of entries.
This article will describe the conversion techniques. The three basic techniques to turn a List into an array in Java are as follows:
- using List.get() function
- applying the toArray() function
- Streaming in Java 8
Using the get() Method
Streaming is one of the easiest approaches for converting a list into an array. This allows us to access each list element individually and add them to the array.
The get() method in the List interface has the following syntax:
public Ele get(int position)
The element that has been situated at the given position within the list is returned by the get() function.
To comprehend how to utilize the get() function of the list, let's look at an example of turning a list into such an array.
Example: ListToArrayEx1.java
//this program is for converting the list into an array
//import section
import java.io.*;
import java.util.LinkedList;
import java.util.List;
// a class ListToArrayEx1 can be created for converting the given list into an array
public class ListToArrayEx1 {
// main() method of the program
public static void main(String[] args)
{
// a linked list was created by declaring the objects to list
List<String> name = new LinkedList<String>();
// the method add() is used for adding the elements to the liked list
name.add("Ramu");
name.add("Sita");
name.add("Raghu");
name.add("Latha");
name.add("Mamatha");
// the size of the linked list is then stored in the length variable
int length = name.size();
// the array elements were stored like the string as the datatype
String[] nameArray = new String[ length ];
//the loop is then iterated over each element over the list completes
//and then con converted it to the list array
for (int i = 0; i < length; i++)
nameArray[i] = name.get(i);
// the values which are in the array are printed
System.out.println("Coverted the list to an array");
for (int j = 0; j < nameArray.length; j++) {
System.out.println((j+1)+" element in the array = "+nameArray[j]);
}
}
}
Output
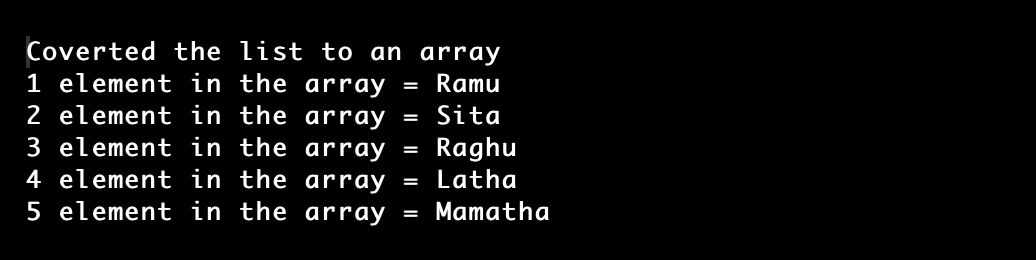
Applying the toArray() Method
The toArray() function makes it simple to change a list into an array. It is an additional approach to array creation from a list. All the entries in the list are returned in an array using the toArray() function. The elements in the resulting array are organized in the exact order as the list.
The List interface's toArray() method has had the corresponding format:
public <T> T[] toArray(T[] array)
The toArray() function returns an array that contains every element in the list and either takes an array as a parameter or does not accept one.
To further comprehend how we may employ the list's toArray() method, let's see another example of transforming a list into such an array.
ListToArrayEx2.java
//this program is for converting the list into an array
//importing the required packages
import java.io.*;
import java.util.LinkedList;
import java.util.List;
//a class ListToArrayEx2 can be created for converting the given list into an array
public class ListToArrayEx2 {
// main() section of the program
public static void main(String[] args)
{
// a linked list was created by declaring the objects to list
List<String> name = new LinkedList<String>();
// the add() method was used for pushing the elements to list
// use add() method of the list to add elements in the linked list
name.add("Panini");
name.add("Daniel");
name.add("Johny");
name.add("Ramesh");
name.add("Mahesh");
//the method toArray() method can be used for converting the list to an array
String[] nameArray = name.toArray(new String[0]);
// the total elements in the list are printed
System.out.println("The list after converted to array");
for (int j = 0; j < nameArray.length; j++) {
System.out.println((j+1)+" element in array = "+nameArray[j]);
}
}
}
Output:
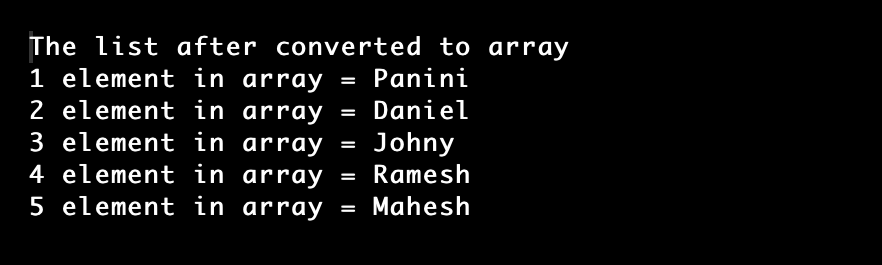
Utilizing Stream
ma may also convert a List into an array utilizing the Stream feature included in Java 8.
The toArray() function of the List interface has the following syntax:
public <T> T[] toArray(T[] array)
The toArray() function only takes one of two parameters: an array or nothing. All of the list's items are contained in the returned array.
ListToArrayExample3.java
//this program is for converting the list into an array
//importing the required packages
//import section
import java.io.*;
import java.util.LinkedList;
import java.util.List;
//create ListToArrayExample3 class
public class ListToArrayExample3 {
// main() section of the program
public static void main(String[] args)
{
// a linked list was created by declaring the objects to list
List<String> name = new LinkedList<String>();
// the add() method is used for adding elements to
name.add("Niranjan");
name.add("Somesh");
name.add("Johny");
name.add("Ramu");
name.add("Mamatha");
//the stream() method, which was used for converting the list to an array
String[] nameArray = name.stream().toArray(String[] ::new);
// the result of all the elements in the array
System.out.println("The converted list into an Array");
for (int j = 0; j < nameArray.length; j++) {
System.out.println((j+1)+" element in the array= "+nameArray[j]);
}
}
}
Output:
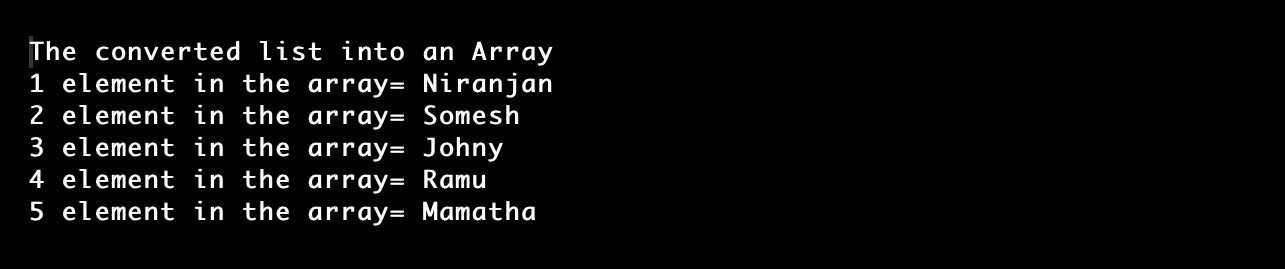