Java Calculate Average of List
The list is a linear data structure used in Java to store ordered data collections. Additionally, it accepts duplicate values while maintaining insertion order. It is sometimes necessary to find the list's minimum and maximum elements, sum, and average. We will calculate the list's average using Java for loop, Java 8 Stream, and the class IntSummaryStatistics.
Using Loops
The reasoning is fairly basic. First, we'll set the value of a variable called sum, which stores the total of the list's members, to zero. Declare a further element called average, which contains the list's average.
To add the elements to the List, we built an instance of the ArrayList class and used its add () function. Every time the list is iterated over, a for loop retrieves an entry and adds it to the variable sum.
After that, we estimated the list's average by dividing the sum by the list's length.
Java Program to find the average of the list using loops
AverageOfList.java
import java . util . * ;
public class AverageOfList1
{
public static void main ( String args [ ] )
{
int s1 = 0 , average=0 ;
ArrayList <Integer> list1 = new ArrayList < Integer > () ;
// Elements are added using add() method
Scanner sc = new Scanner ( System . in ) ;
//System.out.println(" enter the elements of the list ");
list1 . add ( 42 ) ;
list1 . add ( 31 ) ;
list1 . add ( 50 ) ;
list1 . add ( 418 ) ;
list1 . add ( 265 ) ;
System.out.println(" The elements of the list ");
for(int i=0;i< list1.size();i++)
{
int r=list1.get(i);
System.out.println(r);
}
// Iterating over the loop to get a sum of the elements of the list
for ( int j = 0 ; j < list1 . size ( ) ; j++ )
s1 = s1 + list1 . get ( j ) ;
average = s1 / list1 . size ( ) ;
System . out . println ( " Average of the List: " + average ) ;
}
}
Output
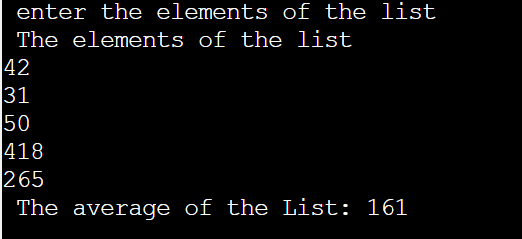
Java Program to find the average of the list using each loop
import java . util . * ;
public class Main
{
public static void main ( String s [ ] )
{
List < Integer > list1 = Arrays . asList ( 10 , 20 , 30 , 40 , 50 , 60 , 70 , 80 , 90 , 100 ) ;
// integer variable declaration to store the sum of elements of the list
int sum1 = 0;
// using each loop to iterate through the list and to get the sum value
for (int j : list)
{
sum1 += j;
}
// checking if the list is empty
if( list1 . isEmpty ( ) )
{
System . out . println ( " Empty List " ) ;
}
else
{
System.out.println("The average of the List is: " + sum1/(float)list1.size());
}
}
}
Output:

Implementing the IntSummaryStatistics Class
The class IntSummaryStatistics is part of java.util package. It uses IntStream class implementation. The focus of the class is using streams. An object of the state is used to gather statistics like count, min, max, total, and average.
stream()
This collection serves as the source of the sequential Stream returned by the function
mapToInt()
It is a lazy intermediate operation. Once the operations have completed their processing, they return a Stream instance as their output. The operations are called on a Stream instance.
summaryStatistics()
This function produces an IntSummaryStatistics containing different summary information about the stream's elements, such as the total number of elements, the stream's average value, the minimum and maximum elements, and more. This is the last action. It implies that it might cross the stream to arrive at a conclusion.
getAverage()
This method provides the arithmetic mean of the values that have been recorded, or 0 if no values have been recorded.
Main.java
import java . util . * ;
public class Main
{
public static void main ( String s [] )
{
List<Integer> list1 = Arrays . asList ( 23 , 516 , 278 , 32 , 6 , 89 , 121 , 534 , 234 , 1 , 5 ) ;
IntSummaryStatistics r = list1 . stream ( ) . mapToInt( ( a ) -> a ) . summaryStatistics ( ) ;
System . out . println ( " The average of the List is: "+ r . getAverage ( ) ) ;
}
}
Output
