Difference Between Data Hiding and Abstraction in Java
Abstraction:
Data Abstraction and Data Hiding ideas are utilised to show the expected data to the end client and conceal the superfluous subtleties, however, for specific purposes like decreasing the framework's intricacy to make it easier to understand.
Abstraction is utilised to show the significant arrangement of administrations or functionalities to the clients and conceal the inward execution subtleties of the code or programming. Information Stowing away, then again, is the cycle that covers the inner information and confines it from straightforwardly getting to buy the program for unapproved access. It is accomplished by utilising access specifiers - private and safeguarded modifiers.
Introduction to Data Hiding and Data Abstraction:
Java is an article-situated programming language. Object-Situated Programming (OOP) is a worldview that assists with making answers for real situations without any problem. Information Stowing away and Information Abstraction is two significant ideas of item situated programming. Be that as it may, both appear to be the same but are entirely unexpected regarding idea and execution, which we will cover exhaustively in this article.
Deliberation conceals the inward execution subtleties and shows just the significant administrations or functionalities to the rest of the world, while Information Stowing away is utilised to cover interior information from the rest of the world so it can not be straightforwardly gotten to by the rest of the world/classes.
What is Data Abstraction:
Abstraction is a course of concealing all pointless subtleties and uncovering just the necessary subtleties. Like we as a whole utilise a PC for making reports, web perusing, messing around, and so on, in our everyday existence. In any case, we don't have the foggiest idea of what is happening inside the PC when we turn it on to play out any activity. Additionally, we sort and send a message when we want to send SMS from our portable. We don't have the foggiest idea about the interior course of the message conveyance. That is the way Reflection works in day-to-day existence, as every one of the superfluous subtleties is covered up.
In Java, Data Abstraction is the most common way of lessening the item to its embodiment to present the vital attributes to the clients. Deliberation depicts a thing as far as its properties which are the qualities, their way of behaving, which is described as strategies, and points of interaction which goes about as a method for speaking with different articles. It is accomplished by involving the theoretical classes and connection points in Java programming.
Let us also figure out dynamic classes and connection points to get it better.
A theoretical class is a limited class that can't be utilised to make objects as it can't be started up. To get to it, it should be acquired from another type. A class is proclaimed conceptual. It might contain unique techniques.
An abstract method is a method that is declared without an implementation (without braces and followed by a semicolon), as given below:
abstract void sum( double a, double b, double c); //we are passing 3 variables
On the off chance that a class incorporates unique techniques, the actual class should be pronounced conceptual, as in:
public abstract class A {
// here, we can also declare fields
// here, we can also declare nonabstract methods
abstract void eat();
}
At the point when a theoretical class is acquired, the acquired type generally gives executions to the academic strategies in its all-parent class. In any case, if it doesn't execute every one of them, then the acquired type should likewise be pronounced conceptual.
Presently we should discuss interfaces. A connection point is a reference type, like a class, containing constants, strategy marks, default techniques, static techniques, and settled styles. Strategy bodies exist just for default techniques and static techniques. Interfaces can't be launched — they must be executed by classes or stretched out by different connection points.
Techniques in a connection point that are not pronounced as default or static are verifiably unique, implying the theoretical modifier isn't utilised with the strategies proclaimed in interfaces.
How to Declare an Interface:
public interface OMB {
//The constant declarations, if any
//here is the method signatures
int turn(Direction d,
double r,
double ss,
double es);
int ST(Direction d,
boolean SO);
......
//The above is the signatures for more methods
}
If we want to use or implement an interface, then we must create or write a class:
public class OKD implements OMB {
// the OMB method signatures, with implementation --
// for example:
public int ST(Direction d, boolean SO) {
//here, we can write the code to turn D LEFT lights turn on
//here, we can write the code to turn D LEFT lights turn off
//here, we can write the code to turn D RIGHT lights turn on
//here, we can write the code to turn D RIGHT lights turn off
}
// other members, as needed -- for example, helper classes are not
// visible to clients of the interface
}
As we have found in the above models, the theoretical technique ST is uncovered in the connection point. In any case, its technique execution is concealed in other classes.
Abstraction Types:
There are fundamentally three sorts of reflection:
Procedural Reflection:
It is a model of what we maintain that a subprogram should do (yet not how to make it happen). It gives instruments to calling obvious techniques or activities elements.
It is the point at which we compose code segments (static techniques) that are summed up by having variable boundaries. The thought is that we have code that can adapt to different circumstances, contingent upon how its limits are set when it is called. It makes a program more limited and more apparent and assists with troubleshooting since when we right a technique, we write the program for every one of the situations where the system is called.
So we accomplish reflection through classes by progressing methodology in the type of capabilities followed consistently in succession.
Data Abstraction:
It is the reflection where we make complex information types and conceal their execution subtleties from the clients. The advantage of this approach is that it settles the presentation issues and works on the execution after some time. Assuming that we roll out any improvements to work on the exhibition, it doesn't ponder the code present on the client side.
In information designs, for example, HashMap or HashSet, we conceal their execution subtleties from the clients and show the significant tasks to collaborate with the information. So deliberation is accomplished from a bunch of information that depicts an item.
Control Abstraction:
It is the product part of deliberation where the program is rearranged, and superfluous execution subtleties are taken out.
Control reflection is the most common way of deciding all comparative and rehashed explanations ordinarily and uncovering them as a solitary unit of work. It keeps the essential guideline of DRY code, which implies Don't Rehash the same thing, and involving capabilities in a program is the best illustration of control reflection.
Data Hiding:
Assume you have a record in the bank. If your equilibrium variable is announced as open in the bank programming, your record equilibrium will be publicly known. In this situation, anybody can realise your record balance you don't need.
This way, the equilibrium variable is proclaimed private to make your record safe so anybody can't see your record balance. The individual who needs to see his record equilibrium should get to private individuals through techniques characterised inside that class. These strategies will request the record holder's name, client id, and secret key for validation. Consequently, we can accomplish security by using the idea of information stowing away.
Thus, Information Stowing away is the interaction that conceals the information from the parts of the program that needn't be bothered to recover. It guarantees restrictive information admittance to class individuals and gives object uprightness by forestalling accidental or expected changes.
We approach modifiers in the Java programming language like public, private, and secured. The other class articles can get to the public techniques and information individuals. The safeguarded individuals are available by the object of a similar class and its subclasses. In any case, the confidential individuals are open inside the class. Subsequently, the individuals are gotten with the assistance of access modifiers.
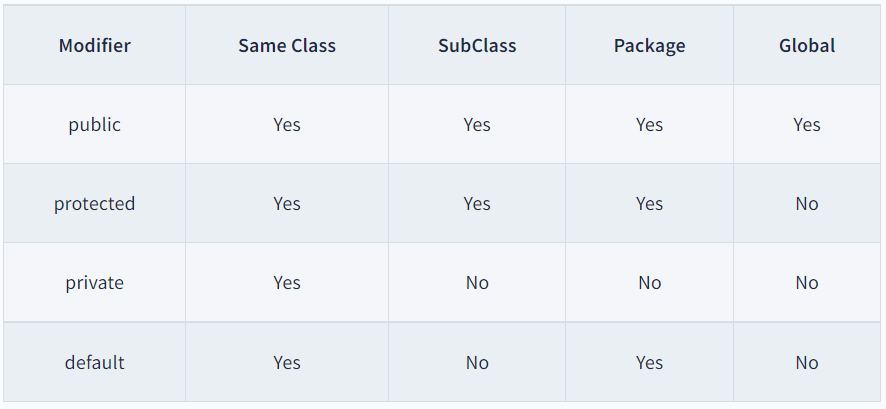
`At the point when it is essential to limit objects of different classes from getting to the individuals from another type, we can utilise the privileged access modifier. Then, the information individuals are just available inside the class. We use getters and setters to make the information private and open external through different courses.
For carrying out information stowing away, exemplification (an OOP idea) is utilised where information and elements of a class are shielded from unapproved access. Conversely, when the knowledge and capabilities are encased into a solitary unit is known as embodiment. Subsequently, the information concealing aids in accomplishing the epitome. The utilitarian subtleties of an item can be dealt with through access specifiers. So the information can not be obtained dishonestly by the capabilities outside the class and is safeguarded from unintentional adjustment.
How to Implement or Achieve Data Hiding:
As we have examined, Information Stowing away is accomplished by utilising the ideas of getters and setters strategies. So individuals are private, and getters and setters are public capabilities to access and refresh. Here, we will exhaustively examine the execution.
At the point when the class fields are announced private, then, at that point, getters and setters are utilised to get to and control their upsides of them. Community specifiers are used with the getter and setter techniques for openness.
Example:
class Student
{
// class member variable
private int SRN;
private String SN;
private int SA;
private String SC;
public int getSRN()
{
return SRN;
}
public void setSRN(final int SRN)
{
this.SRN = SRN;
}
public String getSN()
{
return SN;
}
public void setSN(final String SN)
{
// Validating the student's name if it's null and
// throwing an exception if the name is found to be null or its length is 0.
if(SN == null || SN.length() <= 0)
{
throw new IllegalArgumentException();
}
this.SN = SN;
}
public int getSA()
{
return SA;
}
public void setSA(final int SA)
{
this.SA = SA;
}
public String getSC()
{
return SC;
}
public void setSC(final String SC)
{
this.SC = SC;
}
// for printing the values using getter methods
@Override
public String toString()
{
String st = "Student: [Roll No = " + getSRN() + ", name = " + getSN() + ", age = " + getSA() + ", class = " + getSC() + "]";
return st;
}
}
// Main class.
public class GS
{
// main method
public static void main(String args[])
{
// Creating an object for the Student class
final Student st = new Student();
// the student details are getting set using the setter methods.
st.setSRN(10);
st.setSN("John");
st.setSA(16);
st.setSC("Tenth Class");
// Displaying the student details using the
// 'toString()' method, which uses the getter methods
System.out.println(st.toString());
}
}
Output:
Student: [Roll No = 10, name = John, age = 16, class = Tenth Class]
Explanation:
In the above model, we have made an Understudy class in which all the confidential part factors are proclaimed and might not be straightforwardly outside the Understudy at any point in class. For getting to and changing the qualities, getters and setters are made with community modifiers. By this, we are concealing the information and just utilising the getter-setter strategies, which improves the security as confidential factors won’t get too straightforwardly.
We can likewise put a few circumstances for setting the qualities as required. In this model, we are checking to assume the name is invalid or its length is 0. If both events are valid, it will toss a particular case.
Difference Between Data Hiding and Abstraction in Java:
Abstraction:
- It is the most common way of concealing the inward execution and keeping the confounded strategies away from the client. Just the expected administrations or parts are shown.
- Centers around concealing the intricacy of the framework.
- This is generally accomplished by utilising 'dynamic' class ideas and carrying out interfaces.
- It assists with getting the product as it conceals the interior execution and uncovers the required capabilities.
- It doesn't influence end clients since the designers can perform changes inside in execution classes without changing the theoretical technique in the connection points.
- It very well may be executed by making a class that addresses important qualities without including foundation detail.
- Abstraction shows just the fundamental data and conceals the little subtleties.
- The abstraction's motivation is to conceal the intricate execution subtleties of the program or programming.
- Abstraction is utilised in class to create another client-characterised information type or a new user-defined data type.
- In abstraction, dynamic classes and connection points are utilised.
- Abstraction centres around the recognisable way of behaving the information.
Data Hiding:
- The interaction guarantees selective information admittance to class individuals and concealing the inner information from outcasts.
- The interaction guarantees selective information admittance to class individuals and concealing the inner information from outcasts.
- This can be accomplished utilising access specifiers, for example, 'private' and 'secured'.
- This goes about as a security layer. It protects the information and code from outer legacy as we utilise setter and getter techniques to set and get the information in it.
- This guarantees that clients can't get to inward information without confirmation.
- Getters and setters can be utilised to get to the information or to alter it.
- Data Hiding is utilised to conceal the information from the parts of the program by guaranteeing select information admittance to class individuals.
- Data Hiding is carried out to accomplish embodiment.
- Data Hiding is utilised to make the information private.
- Data Hiding, getters and setters are utilised for the execution.
- Data Hiding limits or permits the utilisation of information inside a container.