Java 9 Try With Resources
Java 9 provides the improvement in the try statement. It allows us to declare a try statement with duly declared resources. Whenever the user does not require the functionality with a try with the resource it must be closed. In Java 9 we have the Try-with Resources functionality which ensures the user that the resource object will be closed whenever the requirement finishes. It increases the efficiency of the program. It reduces the overhead of closing the file connection, network connection explicitly.
Java 9 Try With Single Resources Example:
import java.io.*;
public class Main {
public static void main(String[] args)
{
try (FileOutputStream fosout= new FileOutputStream("file.txt")) {
String text = " Hello, Welcome to Try with resource feature of Java 9 ";
byte array[] = text.getBytes();
fosout.write( array );
}
catch (Exception e) {
System.out.println(e);
}
System.out.println(" Message has been written in the file.txt and resources are closed successfully ");
}
}
Output:
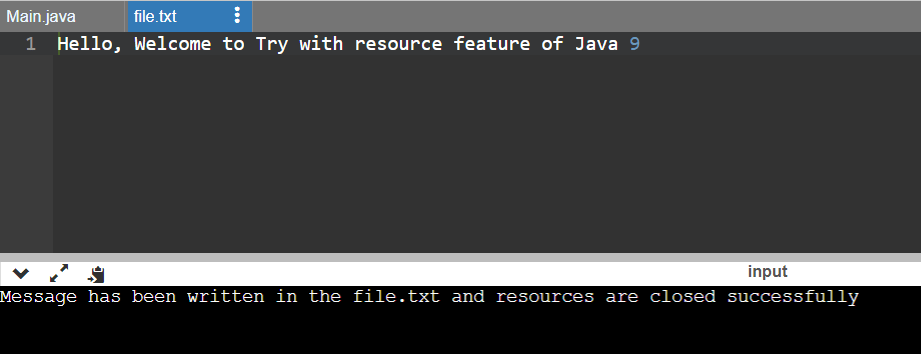
Explanation:
In java 9, we have the facility to use try with resource functionality. We have declared one class main inside which the file outstream object created inside the try. The byte array is obtained from text using the getBytes() method. Then this content is written into the file outstream object and then try with resource functionality in java 9 closes all the connections without forcing the user to manually do it. And then the message is printed " Message has been written in the file.txt and resources are closed successfully " with file written with the message " Hello, Welcome to Try with resource feature of Java 9 ".
Java 9 Try With Multiple Resources Example:
import java.io.*;
public class Main {
public static void main(String[] args) {
try ( FileOutputStream fosout = new FileOutputStream ( "output.txt" );
BufferedReader br = new BufferedReader( new FileReader( "file.txt" )))
{
String text;
while (( text = br.readLine()) != null ) {
byte array[] = text.getBytes();
fosout.write(array);
}
System.out.println("File content copied from one file another file.");
}
catch (Exception e) {
System.out.println(e);
}
System.out.println(" Message has been written in the output.txt and resources are closed successfully ");
}
}
Output:
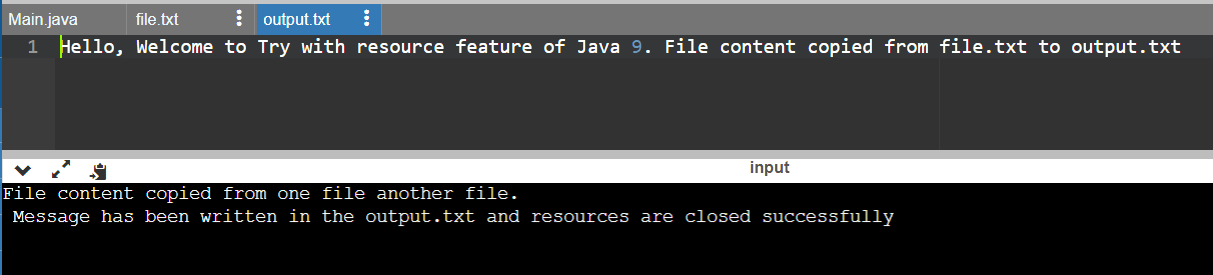
Explanation:
In java 9, we have the facility to use multiple try with resource functionality. We have declared one class main inside which the file outstream object created inside the try. Also, the BufferedReader object is created having the file.txt passed inside it. Then using this object we are reading the content from file.txt and writing it to the another file and then try with resource functionality in java 9 closes all the connection without forcing the user to manually do it. And then the message is printed "File content copied from one file another file." Also prints, " Message has been written in the output.txt and resources are closed successfully " with file written with the message " Hello, Welcome to Try with resource feature of Java 9. File content copied from file.txt to output.txt ".
Java 9 Try With Resource Improvement:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.Reader;
import java.io.StringReader;
public class Main {
public static void main(String[] args) throws IOException {
System.out.println( check1(" Java Earlier version require extra br object "));
System.out.println( check2(" Java 9 dont require extra br object for reading "));
}
static String check1(String message) throws IOException {
Reader input = new StringReader( message );
BufferedReader br = new BufferedReader( input );
try ( BufferedReader br1 = br ) {
return br1.readLine();
}
}
static String check2(String message) throws IOException {
Reader input = new StringReader( message );
BufferedReader br = new BufferedReader( input );
try (br) {
return br.readLine();
}
}
}
Output:

Explanation:
In java 9, we have the facility to use try with resource functionality. It improves the efficiency of the code. In this, we have declared one class main inside which we have created two method check1() and check2(). The check1() method shows the functionality using the java earlier versions in which the BufferedReader object is created the first time to store the Reader input and again we have to create the second BufferedReader object br1 which stores br content and then return it. Rather in the check2() method which uses the java 9 functionality single BufferedReader br is created which stores the input message and also returns content with the same br object. In this way, Java 9 provides user convenience and saves time using try with resource functionality.