Jagged Array in Java
Prerequisite
We must first understand what Arrays are and Multi-dimensional Arrays are before we can learn about Jagged arrays.
Java Arrays: A collection of data types that are similar is called an array.An array is a container object for values of the same type. Because the size of an array must be defined at the time of its declaration, it is also known as a static data structure.
The array starts at zero and runs up to n-1, where n is the array's length. The array is considered an object in Java and is stored in heap memory. It enables the storage of primitive or reference values. In Java, an array can be single-dimensional or multidimensional.
Array's Features
- It is indexed at all times. The index starts at zero.
- It's a collection of data kinds that are similar.
- It occupies a contiguous memory location.
- It allows to access elements randomly.
Multi-Dimensional Array
A multi-dimensional array resembles a single-dimensional array in appearance. Unlike a single-dimensional array, which can only have a one-row index, it can have several rows and columns. It is a tabular representation of data in which data is kept in rows and columns.
Declaration of a Multi-Dimensional Array
datatype[ ][ ] array_name;
Array initialization
datatype[ ][ ] array_name = new int[rows_no][columns_no];
The array_name is the name of the array, new is a memory allocation keyword, and rows_no andcolumns_noare used to specify the size of the rows and columns.
We can statically initialize multi-dimensional arrays the same way we can single-dimensionalarrays.
int[ ][ ] arr = {{5,6,3,4,2,17},{7,8,10,9,11,18},{12,15,16,14,1,19}};
Example:
class Demo
{
public static void main(String[] args)
{
int arr[ ][ ] = {{5,6,3,4,2,17},{7,8,10,9,11,18},{12,15,16,14,1,19}};
for(int j =0; j <3;j++)
{
for (int k = 0;k<6 ; k++) {
System.out.print(arr[j][k]+" ");
}
System.out.println();
}
// assigning a value
System.out.println("element at first row and second column: " +arr[0][1]);
}
}
In the first row and second column, there are 5,6,3,4,2,17,7,8,10,9,11,18,12,15,16,14,1,19 elements.
What is Jagged Array?
A jagged array is a special type of array that has different numbers of column elements. A ragged array is another name for a jagged array. The term "array of arrays" is sometimes used to describe a jagged array. In Java, a jagged array is a multi-dimensional array with columns of various sizes. But in a jagged array size of all single arrays is different from each other.
In other words, an array in which each row may have a varied number of elements.If we create a different number of columns in a 2-D array, that is known as a jagged array.
For instance: In Java, an array of single-dimensional arrays is called a two-dimensional array. In a two-dimensional array, each one-dimensional array has its column.
A graphical illustration of a Jagged array is shown below.
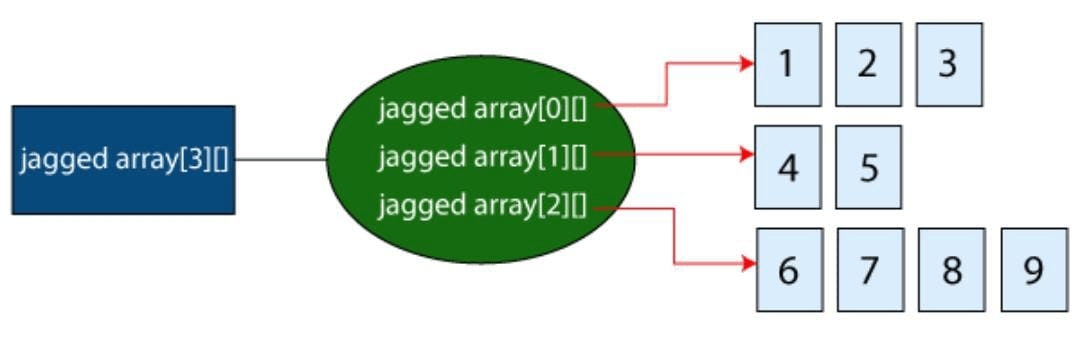
We got an idea of how it looks from the preceding pictorial portrayal. A two-dimensional Jagged array is seen above. As demonstrated above, each element of this array is a one-dimensional array with varying sizes.
The first row of the first 1D array contains three columns, the second row has two columns, and the third row has four columns.
How to create a Jagged Array
Only the first dimension, which specifies the number of rows in the array, is specified when building an array of arrays.
As an example, here's how to make a two-dimensional jagged array:
int arr[][] = new int[3][];
A two-dimensional array with three rows is declared in the previous declaration.
You can define the array as a Jagged array once it is declared, as illustrated below:
arr[0] = new int[4];
arr[1] = new int[1];
arr[2] = new int[2];
arr[3] = new int[3];
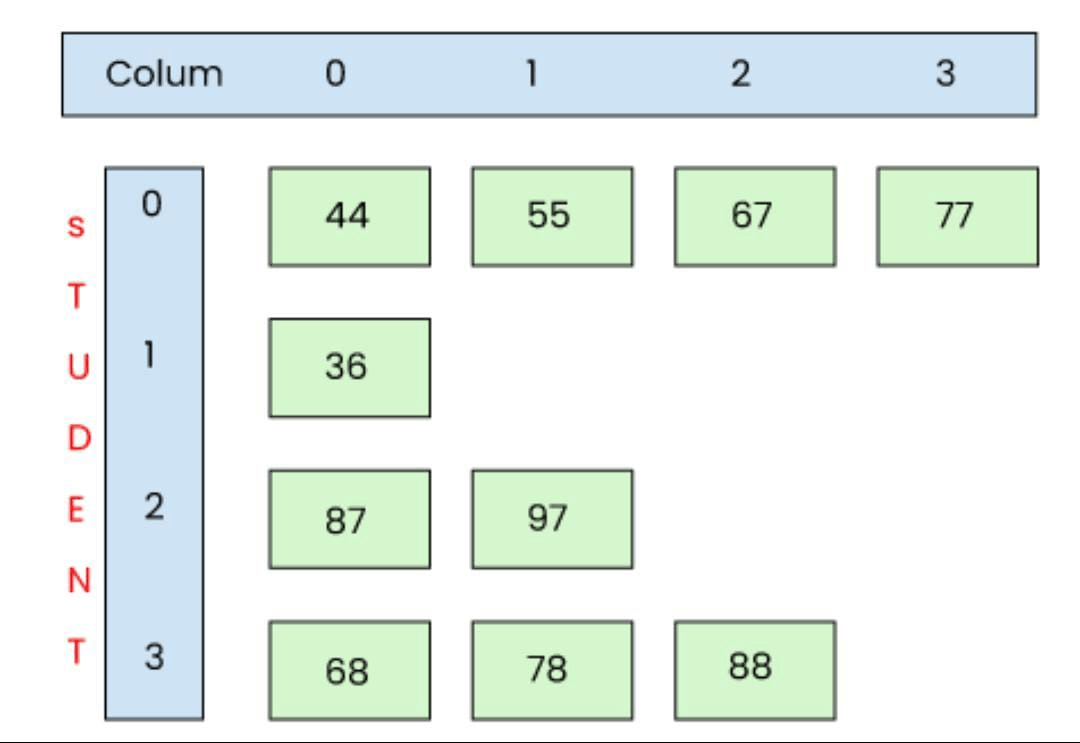
The first sentence indicates that the 2D array's first row will have two columns. The second row will have three columns, and the third row will have four, resulting in a Jagged array.
After the array has been constructed, you can fill it with values. If you don't explicitly initialize this array (as in the example above), the default values will be used as initial values, depending on the array's data type.
You may also use the following syntax to create an array:
intarr[][] = newint[][]
{
newint[] { 3 , 4 , 5 , 6};
newint[] { 7 , 8 , 9} ;
newint[] { 10 , 11} ;
} ;
Another technique to create a Jagged array is to leave off the first new operator, as seen below:
int[][] arr = {
newint[] { 3 , 4 , 5 , 6 };
newint[] { 7 , 8 , 9 };
newint[] { 10 , 11};
};
The new operator is omitted, and the array is both initialized and defined in the same statement, as shown above.
You can alternatively use a declaration and initialization statement instead of all the new operators, as illustrated below.
int[][] arr = {
{ 3 , 4 , 5 , 6} ,
{ 7 , 8 , 9} ,
{ 10 , 11} } ;
By assigning initial values to each row, the software below creates a ragged array. The column values are initialized in each row of the array.
classMain
{
publicstaticvoidmain(String[] args)
{
// Declare a three-row, two-dimensional array.
intarr[][] = newint[5][];
// jagged array definition and initialization
arr[0] = newint[]{ 1 , 2};
arr[1] = newint[]{ 3 , 4 , 5};
arr[2] = newint[]{6,7};
arr[3] = newint[]{ 8 , 9 , 10 , 11 };
arr[4] = newint[]{ 12 , 13 , 14 };
// show the jagged array
System.out.println("Jagged Array in Two Dimensions:");
for(inti=0; i<arr.length; i++)
{
for(intj=0; j<arr[i].length; j++)
System.out.print(arr[i][j] + " ");
System.out.println();
}
}
}
Output:
Jagged Array in Two Dimensions:
1 2
3 4 5
6 7
8 9 10 11
12 13 14
The first row of the Jagged array has three columns, the second row has two columns, and the third row has five columns, as seen in the output.
An example of Jagged Array
class Demo
{
public static void main(String[] args)
{
int arr[ ][ ] ={{ 3 , 4 , 5 , 6} , { 7 , 8 , 9} , { 10 , 11} } ;
for(int i=0;i<3;i++)
{
for (int j = 0; j <arr[i].length; j++) {
System.out.print(arr[i][j]+" ");
}
System.out.println();
}
}
}
Output:
3, 4, 5, 6, 7, 8, 9, 10, 11
Here, the number of rows is three, and the columns for each row are different. The term "jagged array" refers to this form of an array.
Most Commonly Asked Questions:
Q #1) In Java, what exactly is a Jagged array?
Answer: It's a multi-dimensional array with varying sizes for each element of this array, which is another array. As a result, we can make a two-dimensional Jagged array with columns of various sizes.
Q #2) What purpose does a Jagged array serve?
Answer: Multiple-dimensional jagged arrays can significantly increase performance.
Q #3) What is called an array of arrays?
Answer: The multidimensional array, often known as an array of arrays, is commonly represented as a matrix.
Q #4) Where are used of Multidimensional arrays?
Answer: Information that requires a matrix form, such as timetables, timetables, and floor layouts, can be stored in multidimensional arrays, including jagged arrays.