Convert milliseconds to date in Java
In Java, we frequently need to convert milliseconds into Dates with several formats, including dd MM yyyy and dd MM yyyy HH:mm:ss:SSS Z, among others.
The Date class is one of the most crucial Java classes for working with dates. The Date is internally stored in milliseconds. We can construct a Date from milliseconds by utilizing the constructor of the Date class.
It is necessary to familiarise yourself with the SimpleDateFormat class before moving on. One of the classes we use to format and parse data is this one. It aids in the conversion of dates between different formats. It helps convert a string-formatted date into a date object. In simple terms, Date is modified appropriately by using the SimpleDateFormat class.
Java has numerous options for converting milliseconds to dates. Both the java.util.Calendar.setTimeInMillis() method and the java.util.Date(long Millis) method is available. Examples of both approaches to constructing Date from A Millisecond in Java will be shown in this article.
In this case, we are formatting Date in Java using SimpleDateFormat, which is not thread-safe and shouldn't be shared by several threads.
Program 1
Filename: MillisecondsToDate1.java
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.Scanner;
// to translate milliseconds into dates, develop the MillisecondsToDate1 class.
public class MillisecondsToDate1 {
// start the main() method
public static void main(String args[])
{
// declaration for the milliseconds variable
long miliSec;
// Scanner class object creation
Scanner sc = new Scanner(System.in);
System.out.print("Enter milliseconds: ");
// receive user input and initialize the variable
miliSec = sc.nextLong();
// close scanner class obj
sc.close();
// to change the date object, create a new instance of the SimpleDateFormat class.
DateFormat obj = new SimpleDateFormat("dd MMM yyyy HH:mm:ss:SSS Z");
//We create a Date instance and supply the constructor with milliseconds.
Date res = new Date(miliSec);
// The res is now formatted using SimpleDateFormat.
System.out.println(obj.format(res));
}
}
Output
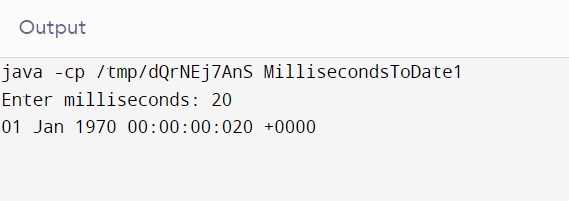
Program 2
Filename: MillisecondsToDate2.java
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
// to transform milliseconds into dates, develop the MillisecondsToDate2 class.
public class MillisecondsToDate2 {
// start the main() method
public static void main(String args[]) {
// To obtain the current time in milliseconds, use the system's currentTimeMillis() method.
long milliSec = System.currentTimeMillis();
// By creating an object of the date class and providing milliseconds to the constructor, you can convert milliseconds into the Date.
Date res = new Date(milliSec) ;
// printing the Date value (default format)
System.out.println("After converting milliseconds into date: " + res);
// Make an instance of the SimpleDateFormat class to convert the date object to dd: MMM yyyy HH:mm:ss:SSS Z and dd:MM:yy:HH:mm:ss format.
DateFormat sdf1 = new SimpleDateFormat("dd:MM:yy:HH:mm:ss") ;
DateFormat sdf2 = new SimpleDateFormat("dd MMM yyyy HH:mm:ss:SSS Z") ;
// after converting the res into dd:MM:yy:HH:mm:ss and dd MMM yyyy HH:mm:ss:SSS Z format
System.out.println("Date in dd:MM:yy:HH:mm:ss: " + sdf1.format(res));
System.out.println("Date in dd MMM yyyy HH:mm:ss:SSS Z: " + sdf2.format(res));
// Using the calendar class, convert milliseconds to dates.
// a Calendar class instance should be created.
Calendar calndr = Calendar.getInstance();
// To set the time, use the calendar class's setTimeInMillis() method. calndr.setTimeInMillis(milliSec);
// after formatting the date into dd:MM:yy:HH:mm:ss
System.out.println("After converting milliseconds into date using Calendar class: "+ sdf1.format(calndr.getTime()));
// transferring data from one date to another
Date currentdate = new Date();
Date tempDate = new Date(currentdate.getTime());
System.out.println("original Date: " + sdf1.format(currentdate));
System.out.println("copied Date: " + sdf1.format(tempDate));
}
}
Output
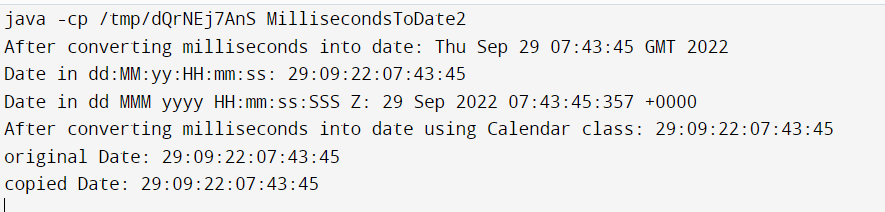
Program 3
Filename: MillisToDate.java
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
public class MillisToDate {
public static void main(String args[]) {
// java.util.Date is used to convert milliseconds to dates.
//current time in milliseconds
long currentDateTime = System.currentTimeMillis();
// making a date from milliseconds
Date currentDate = new Date(currentDateTime) ;
//printing value of Date
System.out.println("current Date: " + currentDate) ;
DateFormat df = new SimpleDateFormat("dd:MM:yy:HH:mm:ss") ;
//formatted value of current Date
System.out.println("Milliseconds to Date: " + df.format(currentDate)) ;
//Converting milliseconds to Date using Calendar
Calendar cal = Calendar.getInstance();
cal.setTimeInMillis(currentDateTime) ;
System.out.println("Milliseconds to Date using Calendar:"
+ df.format(cal.getTime())) ;
//copying one Date's value into another Date in Java
Date now = new Date() ;
Date copiedDate = new Date(now.getTime()) ;
System.out.println("original Date: " + df.format(now)) ;
System.out.println("copied Date: " + df.format(copiedDate)) ;
}
}
Output

That concludes the discussion about milliseconds and dates in Java. Two methods have been demonstrated, one of which uses the Date class and the other of the Calendar class.