Swastika Pattern in Java
This part teaches us how to create the Swastika Pattern in Java utilizing user-defined columns and rows as well as stars or other special characters.A Java pattern application that is very difficult to code.We need to make certain that the user enters odd numbers for the rows and columns to obtain the ideal Swastika since it will be simple to locate the middle of the rows and columns.
The rows and columns must be the same size and have an odd number.The result will be a flawless swastika pattern.
The following is how the Swastika will be printed using the "for" loop
Program for the Swastika Pattern in Java
import java.io.*;
import java.util.Scanner;
class SwastikaPattern
{
public static void main (String[] args)
{
int rows, cols;
Scanner sc = new Scanner(System.in);
System.out.println("Please enter odd numbers for rows and columns to get perfect Swastika.");
System.out.println("Enter total rows");
rows = sc.nextInt();
System.out.println("Enter total colums");
cols = sc.nextInt();
sc.close();
designSwastika(rows, cols);
}
static void designSwastika(int rows, int cols)
{
for (int u = 0; u < rows; u++)
{
for (int v = 0; v < cols; v++)
{
//check to see if u is less than half of the rows or not.
if (u < rows / 2)
{
// check to see if v is less than half of the cols or not
if (v < cols / 2)
{
// if v == 0, print "*"
if (v == 0)
{
System.out.print("*");
}
// if not, we print space
else
{
System.out.print(" "+ " ");
}
}
// check to see if v is equal to half of the columns.
else if (v == cols / 2)
{
System.out.print(" *");
}
else
{
// The first row will have a "*" if u = 0.
if (u == 0)
System.out.print(" *");
}
}
// check to see if u equal half of the rows or not.
else if (u == rows / 2) {
System.out.print("* ");
}
else
{
// The middle and final columns will have the symbol "*" when u > rows/2.
if (v == cols / 2 || v == cols - 1)
{
System.out.print("* ");
}
else if (u == rows - 1)
{
// The very last row will have a '*' if v = cols/2 or if it is the last column.
if (v <= cols / 2 || v == cols - 1)
{
System.out.print("* ");
}
else {
System.out.print(" "+ " ");
}
}
else
System.out.print(" "+" ");
}
}
System.out.print("\n");
}
}
}
Output of the above program
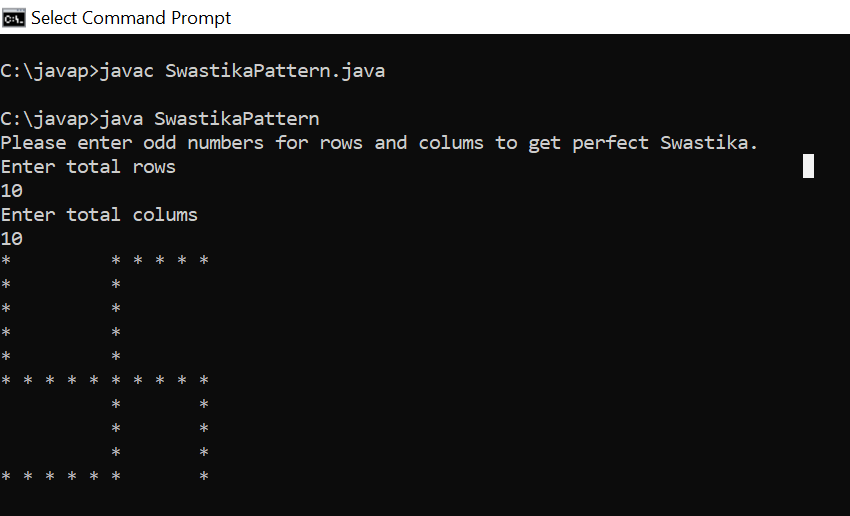