Creation of Multi Thread in java
What are threads in Java?
We can use threads to facilitate parallel processing. Threads are helpful when you wish to execute several pieces of code concurrently.
A thread is a small process that may run numerous pieces of code concurrently. The thread, however, differs from an operation. Each process will have its own memory space allocated in the OS. The same holds true for threads, as they have distinct memory. The same RAM that the process has been allotted will be used to run all the threads.
Thread creation in Java:
Generally, the creation of a thread can be done in two ways in Java.
- Implementing Runnable interface
- Extending Thread class
Multi-thread in Java:
Java's multithreading feature allows for the concurrent execution of several threads.
Because threads employ a shared memory space, multithreading is preferred to multiprocessing. They conserve memory by not allocating separate memory areas, and context switching between the threads is quicker than the process.
Animated films and video games are the main uses of Java multithreading.
Multi-thread execution in Java:
MyThreadExample. java
class MyThread implements Runnable
// implementing MyThread class with Runnable interface
// you can define with any class name
{
int n;
MyThread (int n)
{
this.n = n;
}
public void run ()
{
for (int i = 1; i <= n; i++)
{
// creating different thread procedures
System.out.println (" Thread One " +i);
// printing thread one
if (i%2 == 0)
{
System.out.println ("Even No from Thread Two" +(i*i));
// printing thread two i.e., if “i” is even
// then it prints the square of “i”
}
else
{
// printing thread three, i.e., the cube of the odd digit
System.out.println ("Odd No. from Thread Three " +(i*i*i));
}
try
{
Thread.sleep (500);
// thread in sleep cycle of 500ms
// threads execute after 500ms after first thread execution
}
catch (InterruptedException ie)
{
ie.printStackTrace ();
}
}
}
}
class MyThreadExample
{
public static void main (String args [])
{
MyThread m1 = new MyThread (5);
MyThread m2 = new MyThread (5);
// invoking thread class
Thread t1 = new Thread (m1);
Thread t2 = new Thread (m2);
// creation of thread methods
t1.start ();
t2.start ();
// implementation of threads
}
}
Output:
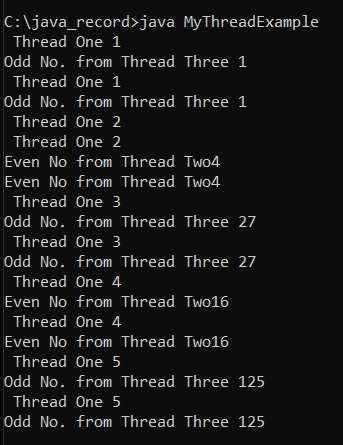
Creating multi -thread by extending thread class:
MyThreadExample.java
class MyThread extends Thread
// implementing MyThread class with extending thread class
// you can define with any class name
{
public void run (int n)
{
for (int i = 1; i <= n; i++)
{
// creating different thread procedures
System.out.println (" Thread One " +i);
// printing thread one
if (i%2 == 0)
{
System.out.println ("Even No from Thread Two" +(i*i));
// printing thread two i.e., if “i” is even
// then it prints the square of “i”
}
else
{
// printing thread three, i.e., the cube of the odd digit
System.out.println ("Odd No. from Thread Three " +(i*i*i));
}
try
{
Thread.sleep (500);
// thread in sleep cycle of 500ms
// threads execute after 500ms after first thread execution
}
catch (InterruptedException ie)
{
ie.printStackTrace ();
}
}
}
}
class MyThreadExample
{
public static void main (String args [])
{
// invoking thread class
MyThread t1 = new MyThread ();
t1.run (5);
MyThread t2 = new MyThread ();
t2.run (5);
// creation of thread methods
t1.start ();
t2.start ();
// implementation of threads
}
}
Output:
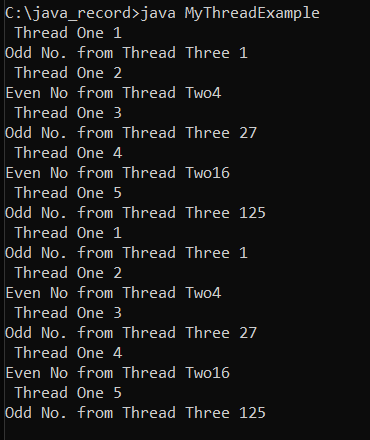
Some uses of multithreading:
- Threads are autonomous, and you can do numerous operations simultaneously; it doesn't block the user.
- It saves time since numerous operations can be completed simultaneously.
- Because each thread is autonomous, if an exception arises in one thread, it has no effect on the other threads.
Multitasking:
Executing numerous things at once is known as multitasking. To make use of the CPU, multitasking is used. You can multitask in two different ways:
- Process-based Task Switching (Multiprocessing)
- Multiple tasks based on threads (Multithreading)
Process-based Task Switching:
- Each process has a memory address. In other words, distinct memory space is assigned to each process.
- A procedure is substantial.
- Communication between processes has a significant cost.
- For the purposes of storing and loading registers, memory mappings, updating lists, etc., switching from one process to another takes some time.
Multiple tasks based on threads:
- The same address space is used by all threads.
- The threads are lightweight.
- The thread cost of communication is minimal.