MessageDigest in Java
The compression of mathematical numbers is accomplished using hash functions. In almost every data security application, hash functions are employed. The hashing, often called has values, returns a value called MessageDigest. While the user-provided input for hashes can be any length, the result always has a set length.
When transforming a communication of any length to a hash algorithm, Java. The security module has a class called MessageDigest that implements techniques like SHA-1, SHA 256, and MD5, among others.
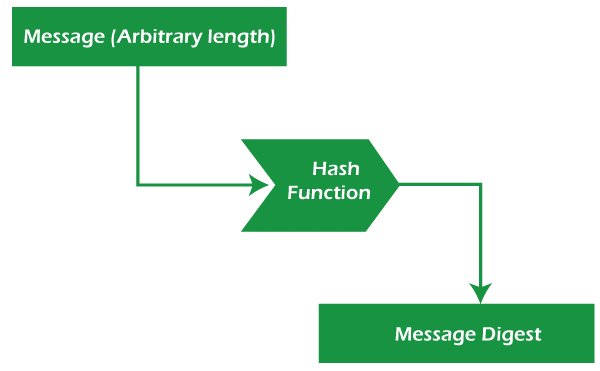
The steps below are used to transform a message together into MessageDigest:
1. The getInstance() function of the MessageDigest class returns an instance performing the provided algorithm. In the initial step, you will use the MessageDigest's getInstance() function to construct an example of MessageDigest. This algo argument, which specifies the method used, is accepted by the getInstance() function.
Syntax:
MessageDigest object = MessageDigest.getInstance("SHA-1");
2. In the subsequent step, we will provide the information to newly generated MessageDigest instances. We employ the MessageDigest subclass' update() function to send the information. Information that takes the form of a bytes array is accepted by that the update() function.
Syntax:
object.update(msg.getBytes);
3. The message digest will be created using the digest() function of a MessageDigest class during the following step. The digest() function returns the decoder as a bytes array. The present object's
hashing function is determined using the digest() procedure.
Syntax:
byte[] digest = object.digest();
The program can understand the methods.
Example: MessageDigestEx.java
// this program is for message digesting in java
//importing the packages required
import java.io.*;
import java.util.*;
import java.security.MessageDigest;
import java.util.Scanner;
// a class is created MessageDigestEx for using the MessageDigest Class
public class MessageDigestEx {
// the main method of the program
public static void main(String args[]) throws Exception{
// an object was created for the scanner class
Scanner sca = new Scanner(System.in);
System.out.println("Please enter the data (message) :");
String msgs = sca.nextLine();
// closing up the scanner class
sca.close();
//utilising the getInstance() function and the SHA-256 method, build a new example of a MessageDigest class.
MessageDigest object = MessageDigest.getInstance("SHA-256");
//To send data to the newly generated MessageDigest Object have used the update() function.
object.update(msgs.getBytes());
//the message digest should be calculated using the digest() technique.
byte[] byteArrays = object.digest();
System.out.println(byteArrays);
// the byte array is converted to hexadecimal format
StringBuffer hexDatas = new StringBuffer();
for (int i = 0; i < byteArrays.length; i++) {
hexDatas.append(Integer.toHexString(0xFF & byteArrays[i]));
}
System.out.println(" The data in the Hexadecimal format : " + hexDatas.toString());
}
}
Output
Please enter the data (message) :
Java Programming Language
[B@6193b845
The data in the Hexadecimal format : adf087da7e732888517370c125734b97fd8a6b39942b982f3ac67e68bb13535
If no provider offers that message digest client applications for the proposed methodology, the getInstance() function of both the MessageDigest classes may raise the NoSuchAlgorithmException.
Example: MessageDigestExceptionExa.java
// this program is for message digesting in java
//importing the packages required
import java.io.*;
import java.util.*;
import java.security.MessageDigest;
import java.util.Scanner;
// a class is created for throwing up the Exception
public class MessageDigestExceptionExa {
// main section of the program
public static void main(String args[]){
// an object is created for the scanner class
Scanner sca = new Scanner(System.in);
System.out.println("Enter the data of any length: ");
String msgs = sca.nextLine();
//end of the scanner class
sca.close();
try {
//utilizing the JTP technique and the getInstance() function, build a new instance of such MessageDigest.
MessageDigest objs = MessageDigest.getInstance("Programming"); // the error is thrown
//To send data to the newly generated MessageDigest instance have used the update() function.
objs.update(msgs.getBytes());
//To determine the message digest, utilize the digest() function.
byte[] byteArrays = objs.digest();
System.out.println(byteArrays);
//the byte array is converted to hexadecimal format
StringBuffer hexDatas = new StringBuffer();
for (int i = 0; i < byteArrays.length; i++) {
hexDatas.append(Integer.toHexString(0xFF & byteArrays[i]));
}
System.out.println("The data in the Hexadecimal format : " + hexDatas.toString());
}catch(Exception e) {
System.out.println(e);
}
}
}
Output
Enter the data of any length:
Programming is good
java.security.NoSuchAlgorithmException: Programming MessageDigest not available