User Defined Exception in Java
An exception is an error (run time error) that happened while a program was being executed. The program stops abruptly whenever the Exception occurs, and the code after the line that caused the Exception has never been executed.
In Java, users can make custom exceptions that are derived classes from the super Exception class. Custom exceptions or user-defined exceptions are those we create on our own. Java custom exceptions are generally utilized to customize the Exception to user needs.
The example sample 1 is a user-defined exception which contains invaliAge, which extends the Exception class.
You will provide us with your exception description by using the custom exception. The getMessage() function on the object we generated may be used to retrieve a message, which we have supplied to the function Object() of the inheritance hierarchy, the Exception class, in this case.
Need to use the user-defined exceptions
Most of the common types of computing exceptions are covered by Java exceptions. But often, we have to make exceptions.
A few arguments for using the user-defined exceptions are as follows:
- To detect a subset of current Java exceptions and give them specific conditions.
- Exceptions to business logic These include business logic or process exceptions. Understanding the exact issue is helpful for both application users and programmers.
We must modify the Java Exception class to create customised exceptions in Java.lang package.
Example for creation of a user-defined exception in Java:
public class NameoftheFile extends Exception {
public NameoftheFile(String exception) {
super(exception);
}
}
Example 1:
Now let us look at a basic Java user-defined exception example. The function Object() of the Exception in the sample code accepts a string as an input. The super() function is used to give this string to the function Object() of the superclass Exceptions. Similarly, there is no requirement to invoke the super() method or use an argument when calling the function Object() of the Exception class.
UserDefinedException1.java
// class UserDefinedException1 denotes the creation of user-defined exception
class AgeException extends Exception
{
public AgeException(String st)
{
// A constructor of the Parent class Exception is called
super(st);
}
}
// class using the user-defined AgeException
public class UserDefinedException1
{
//this block contains the method for checking the age condition
static void check(int age) throws AgeException
{
if(age < 18)
{
// an object is used for throwing the Exception
throw new AgeException("Invalid age");
}
else
{
System.out.println("Person can eligible");
}
}
// main section of the program
public static void main(String args[])
{
try
{
// calling the check method
check(15);
}
catch (AgeException e)
{
System.out.println("Found an exception");
//the message is printing from the AgeException class
System.out.println("The Exception occurred is: " + e);
}
System.out.println("Other lines of the code....");
}
}
Output:

In the above example, when the personage(page) is passed as 15, then it displayed that there occurred an exception Invalid age.
Example 2:
UserDefinedException2.java
// class UserDefinedException2 denotes the user-defined exception
class MyException extends Exception
{
}
// MyException class using the user-defined Exception
public class UserDefinedException2
{
// main section
public static void main(String args[])
{
try
{
// an object is used for throwing the Exception
throw new MyException();
}
catch (MyException ex)
{
System.out.println("found an exception");
System.out.println(ex.getMessage());
}
System.out.println("other lines of code");
}
}
Output:
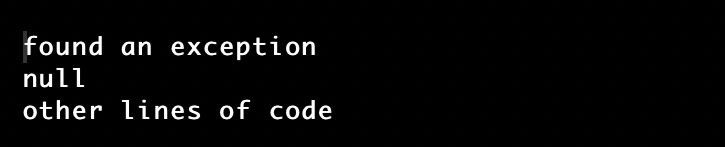