Spliterator in Java 8
In this tutorial, we will understand the meaning of spliterator in java 8. It is just like any other iterator available in java used to traverse the elements of either collection or generator function. It is of great importance in java. It offers some methods to use parallel programming features. However, it directly supports the sequential processing of data. It is an interface present in the Java collection.
There are following eight methods offered by the spliterator interface.
- Characteristics()
- estimateSize()
- getExactSizeIfKnown()
- hasCharacteristics()
- getComparator()
- tryAdvance(Consumer action)
- trySplit()
- forEachRemaining(Consumer action)
Now, let us understand each of the above methods one by one.
Characteristics():
Syntax - int characteristics()
This method does not accept any argument and returns the nature and elements of the calling spliterator.
estimateSize():
Syntax –estimateSize()
This method is to get the estimate of the count of elements remaining for iteration. This method does not accept any argument and returns the count of elements.
getExactSizeIfKnown():
Syntax – default long getExactSizeIfKnown()
This method also does not accept any parameter and returns the count ofelements that are remained to be iterated.
hasCharacteristics():
Syntax –default booleanhasCharacteristics(int characteristics)
This method is takeninto account to detect if the characteristics of the spliterator include all the given characteristics or not.
This method takes the parameter as input and returns true if the characteristics are existing in the spliterator and false if it is not present there.
getComparator():
Syntax - booleantryAdvance()
This method is taken into account to sort. It does not accept any argument but returns some values.
- The method gives out null in the case when the source is sorted in the usualdirection.
- When the source is unsorted, then this method throws an exception called IllegalStateException.
tryadvance():
Syntax - boolean tryAdvance(Consumer<?super T> action)
It accepts action as an argument and returns This method is taken into account toexecute the given action on the remaining elements and returns true. it returns false if the elements are absent.
trysplit():
Syntax - Spliterator trySplit()
This method does not accept any parameter and returns a spliterator that covers nearly some percentage of the elements or is null if the spliterator can’t get split.
forEachRemaining():
Syntax - default void forEachRemaining(Consumer action)
This method is taken into account to execute the specified is used to perform an action on each portion consecutively. It executesan action when the processing of each element is completed or unless the action throws NullPointerException.
Implementation:
Let us try to understand the spliterator methods in a java program.
// packages required for classes
import java.util.ArrayList;
import java.util.Scanner;
import java.util.Spliterator;
import java.util.stream.Stream;
// class named SpliteratorEg1 is created to comprehend all the methods of the Spliterator class
public class SpliteratorEg1 {
// main() method
public static void main(String[] args) {
int size;
// creation of a Scanner class object to get inputs from the user
Scanner sc = new Scanner(System.in);
// Creation of an array list.
ArrayList<Integer>age_list;
System.out.println("Enter the size of the ArrayList:");
size = sc.nextInt();
age_list = new ArrayList<Integer>(size);
for(int i = 0; i< size; i++) {
System.out.println("Enter "+(i+1)+" Age:");
int value = sc.nextInt();
age_list.add(value);
}
// closing the Scanner class object
sc.close();
// getting a Stream to the array list by using the stream() method.
Stream<Integer> stream1 = age_list.stream();
// getting the Spliterator object on ages by using the spliterator() method
Spliterator<Integer> spliterator1 = stream1.spliterator();
// getting the estimate size by using estimateSize()method
System.out.println("The estimated size of the ArrayListis : " + spliterator1.estimateSize());
// getting the exact size of ArrayList if known by using getExactSizeIfKnown() method
System.out.println("The exact size of the ArrayList is: " + spliterator1.getExactSizeIfKnown());
// using hasCharacteristics() and characteristics() methods
System.out.println(spliterator1.hasCharacteristics(spliterator1.characteristics()));
System.out.println("Elements of the ArrayList:");
// using forEachRemaining() method to display each element of the ArrayList
spliterator1.forEachRemaining((n) ->System.out.println(n));
// using the stream() to get another Stream to the ArrayList.
Stream<Integer> stream2 = age_list.stream();
spliterator1 = stream2.spliterator();
// using trySplit() method
Spliterator<Integer> spliterator2 = spliterator1.trySplit();
// Use spliterator2 first in the case spliterator1 could be split,
if(spliterator2 != null) {
System.out.println("Elements of the ArrayList obtained through spliterator2: ");
spliterator2.forEachRemaining((n) ->System.out.println(n));
}
// Now, using the spliterator1
System.out.println("\nElements of the ArrayList obtained from spliterator1");
spliterator1.forEachRemaining((n) ->System.out.println(n));
}
}
Output:
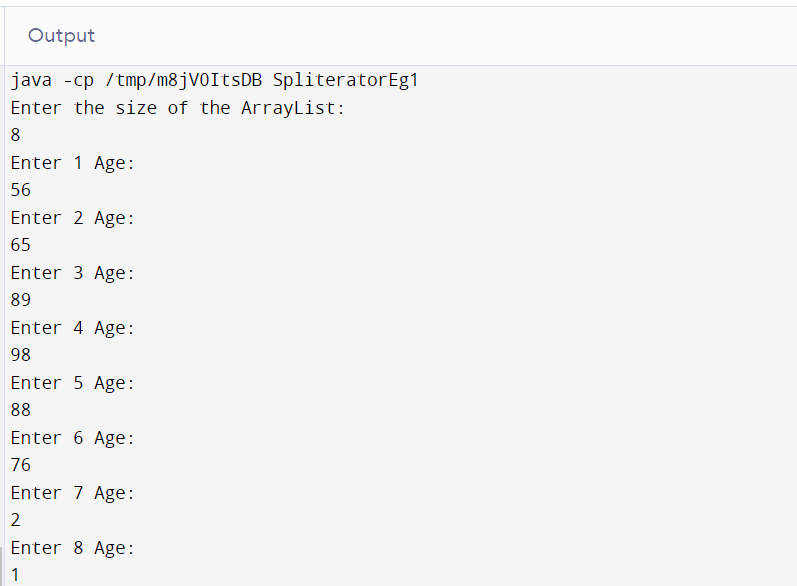
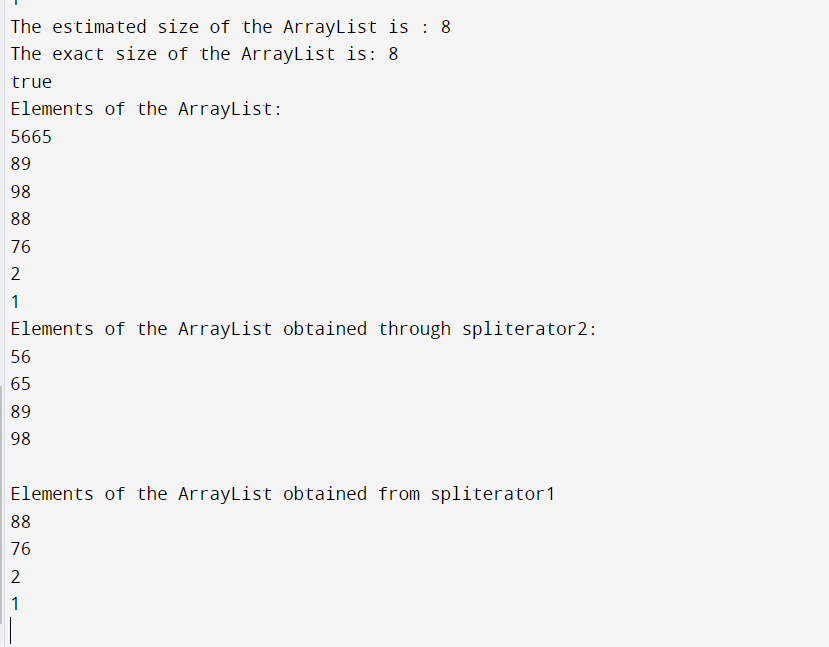
Explanation: In this code, the user is asked to enter the size of the array and the values. Those values are displayed using different spliterator methods.