Split String into String Array in Java
The String split() technique returns a variety of divided strings after the strategy parts the given String around matches of a given normal articulation containing the delimiters. The ordinary articulation should be a legitimate example, and make sure to get away from unique characters if fundamental.
Java Arrays
A cluster is often an aggregation of comparable types of components with coterminous memory space. A Java array is a data structure made up of identical data type components. Furthermore, array components are stored in neighbouring memory space. It is a data structure that stores comparable components. A Java array can only hold a reasonable number of components. Arrays in Java are list-based; the array's primary component is stored in the 0th file, the second component in the first record, and so on. Unlike in C/C++, we can retrieve the length of the array using the length component. We want to use the sizeof administrator in C/C++.
An array is a class object that is progressively constructed in Java. The Java cluster receives the Object class and implements the connection points Serializable and Cloneable. In Java, we may store rudimentary characteristics or articles in an array. In Java, we can create single-dimensional or multidimensional arrays in the same way that we do in C/C++. Furthermore, Java has the element of weird arrays, which C/C++ does not have.
Syntax to Declare an Array in Java:
• dataType[] arr;
• dataType []arr;
• dataType arr[];
Java String
Generally, a String is a grouping of characters. Yet, in Java, the String is an item that addresses a succession of characters. The Java.lang.String class is utilized to make a string object.
Syntax
<String_Type> <string_variable> = "<sequence_of_string>";
Example:
String str = "Hello!";
There are two methods for making String objects:
- By String literal
- By new keyword
1. String Literal: Java String literal is made by utilizing twofold statements.
For Example: String s = "Victory";
2. New Keyword: Here, the new keyword is used for creating the String.
String s=new String("Victory");
The String split() technique returns a variety of divided strings after the strategy parts the given String around matches of a given normal articulation containing the delimiters. The ordinary articulation should be a legitimate example, and make sure to get away from unique characters if fundamental.
Example:
String s= "A-B-C-D"
String[] strArray = s.split("-");
String split() API: The split() strategy is over-burden.
Regex - the ordinary delimiting articulation.
Limit - controls the times the example is applied and consequently influences the length of the subsequent array.
In the event that the breaking point is positive, the example will be applied to all things considered limit - multiple times. The result bunch's length will not be any more conspicuous than the limit, and the cluster's last section will contain all commitments past the last matched delimiter. If the cutoff value is zero, the resulting array can have any size. The following void strings will be eliminated. The return array can be any size if the cutoff is negative.
Syntax:
- public String[] split(String regex);
- public String[] split(String regex, int limit);
Throws PatternSyntaxException:
Split () tosses PatternSyntaxException on the off chance that the normal articulation's linguistic structure is invalid. In the given model, "[" is invalid customary articulation.
Example
Invalid regex example
public class StringEx
{
public static void main(String[] args)
{
String[] str = "hello world…!".split("[");
}
}
Output
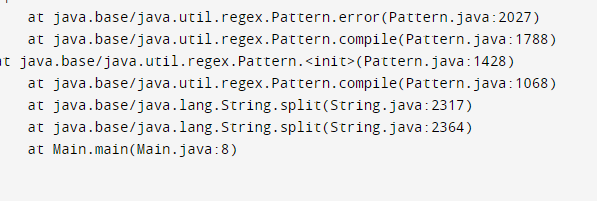
'null' is Not Allowed
The technique doesn't acknowledge 'invalid' contention. It will toss NullPointerException on the off chance that the technique contention is invalid. At the point when invalid articulation is passed to technique.
- Exemption in string "primary" java.lang.NullPointerException
- When Java.lang.String.split(String.java:2324)
- When com.StringExample.main(StringExample.java:11)
Split a String into an Array with the Given Delimiter
Java program to part a string in light of a given token. In the given model, we are parting String for delimiter dash "- ".
Example
public class StringEx
{
public static void main(String[] args)
{
String s= "how to do-in-java-provides-java-tutorials";
String[] strArray = s.split("-");
System.out.println(Arrays.toString(strArray));
}
}
Output:

Split String by Whitespace
Java program to part a string by space utilizing the delimiter "\\s". To part by all blank area characters (spaces, tabs and so forth), utilize the delimiter "\\s+".
Example
public class Strix
{
public static void main(String[] args)
{
String s = " how to do in java provides java tutorials";
String[] strArray = s.split("\\s");
System.out.println(Arrays.toString(strArray));
}
}
Output

Split String by Comma
import java.util.Arrays;
class Main {
public static void main(String[] args) {
String s = "A,B,C,D";
String[] result = s.split(",");
// converting array to string and printing it
System.out.println("result = " + Arrays.toString(result));
}
}
Output
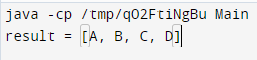
Split String by Multiple Delimiters
Java program to part a string with numerous delimiters. Use regex OR administrator '|' image between different delimiters. In the given model, I am parting the String with two delimiters, dash and speck.
Example
class Main {
public static void main(String[] args) {
String s = " how-to-do-in-java. provides-java-tutorials.”;
String[] result = s.split("-|\\");
// converting array to string and printing it
System.out.println("result = " + Arrays.toString(result));
}
}
Output:

String split(regex, limit)
This variant of the technique additionally parts the String. However, the greatest number of tokens cannot surpass limit contention. After the strategy has been tracked down, given the number of tokens, the remainder of the unspotted String is returned as the last token, regardless of whether it might contain the delimiters. Beneath given is a Java program to part a string by space in, for example, the manner in which the greatest number of tokens cannot surpass 5.
Example
class Main {
public static void main(String[] args) {
String s = " how to do in java provides java tutorials.”;
String[] result = s.split("\\s",5);
System.out.println(result.length);
System.out.println("\n");
// converting array to string and printing it
System.out.println("result = " + Arrays.toString(result));
}
}
Output
