How annotations work in Java
Annotations were introduced by sun microsystem and are available from the java 1.5 version.
In general, configurations can be done either by XML or by annotations.
Hibernate and spring framework users prefer annotations rather than XML and Java marker interface.
The powerful annotations made the configuration easier.
The special character @ is used to represent an annotation.
Annotations will guide the compiler on how to treat a method/class and in associating the data, and has nothing to do at runtime.
Annotations are available in Java.lang.annotation.Annotations which composed of both standard and inbuilt annotations.
@Autowired, @Override, @Deprecated, and @SuppressWarnings are some of the general-purpose annotations.
Types of Annotations:
Annotations are broadly classified into five types.
Marker Annotations-These is used just for declaration, and these annotations do not contain any data. Example Includes @Override
Single value Annotations- This has a value specified along with the Annotation.
Example-- @Annotation(“value”)
Full Annotations- They contain numerous data members.
Example- @Annotation(key=”name”, value=”test”)
Type Annotations- This can be used anywhere. These are declared with @Target Annotation.
Example- @Target(Test. name)
Repeating Annotations- These can be annotated to apply more than once. For example, to repeat, @Repeatable can be used. Mostly the value fields will be an array.
Example-@Repeatable(test.class)
Below described are Some annotations which are predefined and often used.
@Deprecated- A marker annotation indicates a declaration is replaced by the latest version.
@ Deprecated tag is for the runtime reflection, which has a high preference than @Deprecated Annotation.
Test.java:
public class Test{
@Deprecated
public void Display()
{
System.out.println("test display()");
}
public static void main(String args[]) {
test d1 = new test();
d1.Display();
}
}
Output:
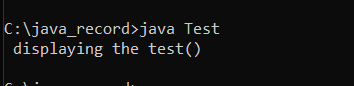
@SuppressWarning This Annotation is used to tell the compiler to suppress a specified compiler warning. @Suppresswarning can be used with a name in string format to suppress. Any unchecked or deprecated warnings are generated.
Test.java:
class Test
{
@Deprecated
public void Display()
{
System.out.println("test display()");
}
}
public class SuppressWarning
{
// If we comment below Annotation, the program generates
// warning
@SuppressWarnings({"test", "check"})
public static void main(String args[])
{
Test d1 = new Test();
d1.Display();
}
}
Output:

@Documented
It tells that Annotation should be documented. The @Document will help tools such as JavaDoc to process the Annotation and inform in the generated document.
@Target It can be used to use one Annotation in another annotation. The @Target has an argument dedicated to constant elementType enumeration. This specifies the declaration to be applied for the Annotation.
Examples of some of the target annotations
Constant Annotation where it is applicable to
ANNOTATION_TYPE for other annotation
FIELD for fields
METHOD for referring to a method
….
@Inheritted is considered as a marker annotation which can be applied to annotation declaration.
@Inherited Annotation will help a subclass to inherit a super class. This helps when an annotation is not present in the subclass, then it tells to check the superclass.
@Override is used to specify that we are overriding a method.
How does @Override work?
Let's have a look at an example...
consider a class with some methods, extends another class, and the extended class should override a method when it is called the @override method is used to ensure that the correct method is over-ridden and else it will return a compilation error in case no method is found to override.
Sample.java
class A{
public void printMeIfCalled(){
System.out.println("I am in A class");
}
}
class B extends A{
public void printMeifcalled(){
System.out.println("I am in B class");
}
}
public class Sample{
public static void main (String[] args){
B call= new B();
call.printMeIfCalled();
}
}
Output:
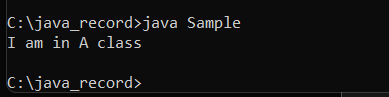
Here the output will be "I am in A class" because the methods are different in both classes. So, overriding is not possible.
If we use @Override in class B for printMeifcalled(), it will throw a compilation error because there is no method to override in class A.
class A{
public void printMeIfCalled(){
System.out.println("I am in A class");
}
}
class B extends A{
@Override
public void printMeifcalled(){ //here, the compilation error will be thrown because the name cannot be overridden and can be corrected.
System.out.println("I am in B class");
}
}
public class sample{
public static void main(String[] args){
B call= new B();
call.printMeIfCalled();
}
}
Let's look at some of the custom or secondary, or user-defined annotations.
It is very rare that an annotation is defined and used. Custom annotations are used to annotate the basic elements such as variables, and methods. This Annotation can be used before declaring an element.
public @interface<Testannotation>
{
String <test>() “testing annotation”;
}