Types of Assignment Operators in Java
In this tutorial, we are going to study assignment operators and their types in Java language. Before proceeding to the types, let us know the term ‘assignment operator’. The assignment operator refers to those operators which are used to assign values written on the right side of the operator to the variable present on the left side of the operator. The data types of value and the variable should be identical to avoid compiler errors. The assignment operator has right-to-left associatively since the values present on the right end of the operand get assigned to the left end of the operator.
Syntax:
Variable operator(=) operand
Types of Assignment Operators
1. Simple assignment operator (=)
It consists of only one symbol.
It simply assigns the value present on the right side of the operator to the left side variable.
Syntax: variable = value
Example
a = 15;
b = 25;
Implementation 1:
Let us see a java program to understand the concept of simple assignment operators in a better way.
// Java code to understand the working of the "=" operator
import java.io.*;
class Simple_Assignment {
public static void main(String[] args)
{
// Declaration of variables
int x;
String s;
// Assigning values
x = 15;
s = "javaTpoint";
// Printing the values
System.out.println("The assigned number: " + x);
System.out.println("The assigned string: " + s);
}
}
Output:
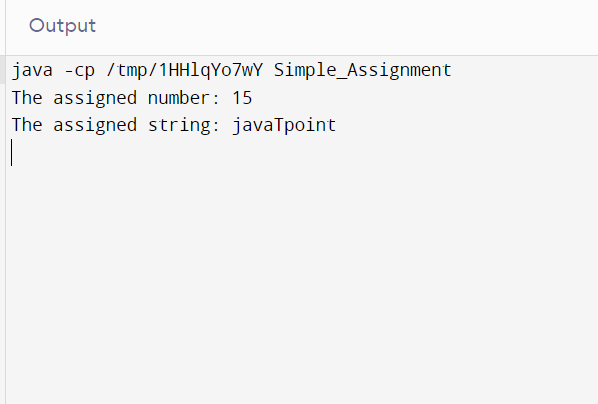
Implementation2:
Let us see another java program to understand the concept of simple assignment operators in a better way.
// Java code to understand the working of the "=" operator
import java.io.*;
class Simple_Assignment {
public static void main(String[] args)
{
// Declaration of variables
int a1;
double a2;
char c;
String s;
// Assigning the values
a1 = 15;
a2 = 15.0;
c = 'A';
s = "javaTpoint";
// Printing the values
System.out.println("The assigned integer: " + a1);
System.out.println("The assigned double value: " + a2);
System.out.println("The assigned character: " + c);
System.out.println("The assigned string: " + s);
}
}
Output:
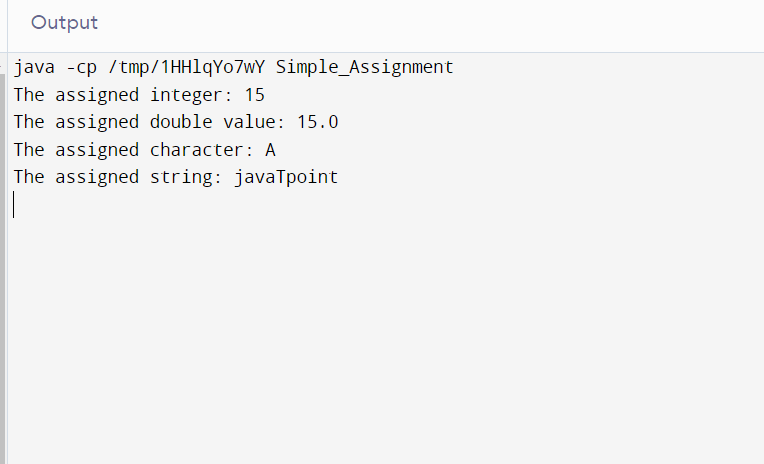
2. Compound assignment operator
It consists of two symbols. It contains one mathematical operator along with an equal to (=) symbol.
It assigns the value present on the right end of the operator to the variable written on the left after performing the indicated operation.
The following are its types.
- (+=) operator: This operator is a union of the ‘+’ and ‘=’ operators.
Working -It adds the current value of the variable on the left end to the value present on the right end and then assigns the resulting value to the variable on the left.
Syntax:
x += y
It is equivalent to x = x + y
Example
x += 40;
It is x = x + 40
Implementation:
Let us see a java program to understand the concept of compound assignment operators in a better way.
// Java code to understand the working of the "+=" operator
import java.io.*;
class Compound_Assignment {
public static void main(String[] args)
{
// Declaration of variables
int a1 = 15, a2 = 35;
System.out.println("first number = " + a1);
System.out.println("second number = " + a2);
// Adding &assigning the sum to a1
a1 += a2;
// Printing the assigned values
System.out.println("final value = " + a1);
}
}
Output:
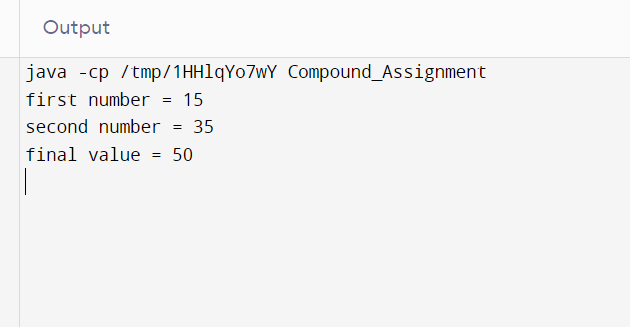
- (-=) operator: This operator is a union of ‘-‘and ‘=’ operators.
Working -It subtracts the value written on the right end of the operator from the current value of the variable on the left end and then assigns the resulting value to the variable on the left.
Syntax:
x -= y
It is equivalent to x = x – y
Example
x -= 40;
It is x = x - 40
Implementation:
Let us see a java program to understand the concept in a better way.
// Java code to understand the working of the "-=" operator
import java.io.*;
class Compound_Assignment {
public static void main(String[] args)
{
// Declaration of variables
int a1 = 15, a2 = 35;
System.out.println("first number = " + a1);
System.out.println("second number = " + a2);
// Subtracting andassigning the difference to a1
a1 -= a2;
// Printing the assigned values
System.out.println("final value = " + a1);
}
}
Output:
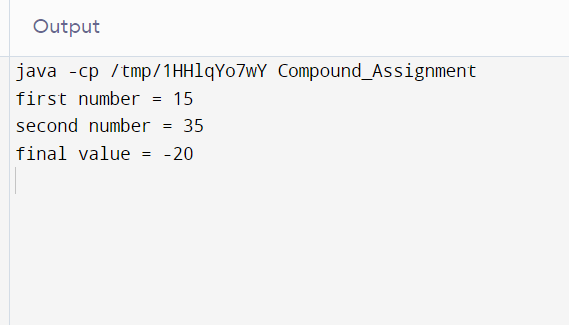
- (*=) operator: This operator is a union of the ‘*’ and ‘=’ operators.
Working -It finds the product of the current value of the variable on the left and the value on the right and then assigns the resulting value to the operand on the left end.
Syntax:
x *= y
It is equivalent to x = x * y
Example
x *= 40;
It is x = x * 40
Implementation:
Let us see a java program to understand the concept in a better way.
// Java code to understand the working of the "*=" operator
import java.io.*;
class Compound_Assignment {
public static void main(String[] args)
{
// Declaration of variables
int a1 = 15, a2 = 35;
System.out.println("first number = " + a1);
System.out.println("second number = " + a2);
// Multiplying andassigning the product to a1
a1 *= a2;
// Printing the assigned values
System.out.println("final value = " + a1);
}
}
Output:
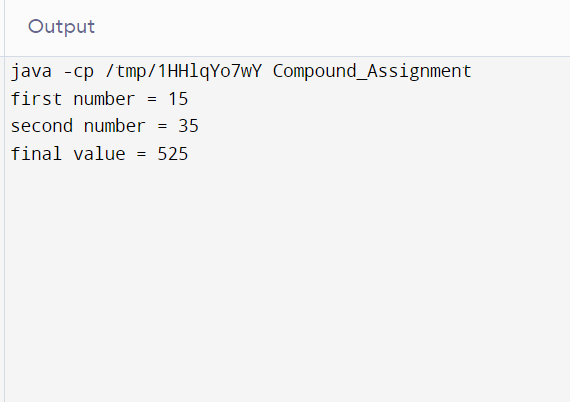
- (/=) operator: This operator is a union of the ‘/’ and ‘=’ operators.
Working -It divides the current value of the variable present on the left endof the operator by the value on the right end and then assigns the quotient or the resulting value to the operand on the left end.
Syntax:
x /= y
It is equivalent to x = x / y
Example
x /= 40;
It is x = x / 40
Implementation:
Let us see a java program to understand the concept in a better way.
// Java code to understand the working of the "/=" operator
import java.io.*;
class Compound_Assignment {
public static void main(String[] args)
{
// Declaration of variables
int a1 = 35, a2 = 15;
System.out.println("first number = " + a1);
System.out.println("second number = " + a2);
// Dividing and assigning values
a1 /= a2;
// Printing the assigned quotient to a1
System.out.println("final value = " + a1);
}
}
Output:
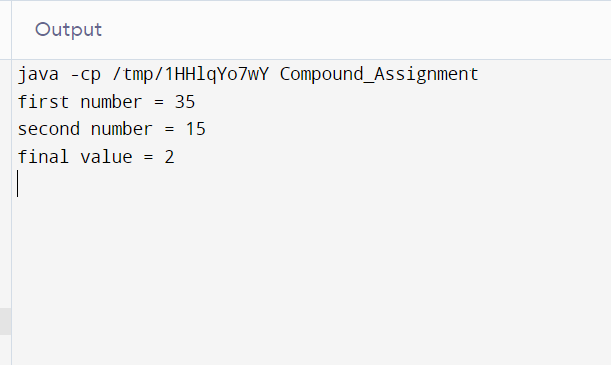
- (%=) operator: This operator is a compound of the ‘%’ and ‘=’ operators.
Working -It divides the current value of the variable present on the left end of the operator by the value on the right and then assigns the remainder to the operand on the left end.
Syntax:
x %= y
It is equivalent to x = x % y
Example
x %= 40;
It is x = x % 40
Implementation:
Let us see a java program to understand the concept in a better way.
// Java code to understand the working of the "%=" operator
import java.io.*;
class Compound_Assignment {
public static void main(String[] args)
{
// Declaration of variables
int a1 = 35, a2 = 15;
System.out.println("first number = " + a1);
System.out.println("second number = " + a2);
// Dividing and assigning the remainder to a1
a1 %= a2;
// Printing the assigned values
System.out.println("final value = " + a1);
}
}
Output:
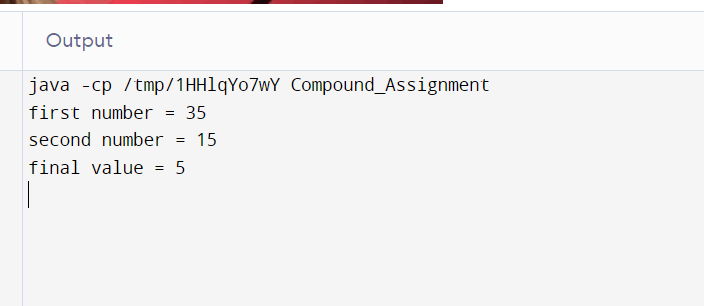
Table of Operators
Operators | Examples | Meaning |
= | a1 = a2 | a1 = a2; |
+= | a1 += a2 | a1 = a1 + a2; |
-= | a1 -= a2 | a1 = a1 - a2; |
*= | a1 *= a2 | a1 = a1 * a2; |
/= | a1 /= a2 | a1 = a1 / a2; |
%= | a1 %= a2 | a1 = a1 % a2; |
This table shows a list of assignment operators along with examples and meanings we studied previously.
Summary
We understood the meaning of assignment operator. We saw there exist two main types of java assignment operators – simple and compound. Further, we saw the six types of compound assignment operators offered in java language and dealt with each type with the aid of examples and java code.