Types of Statements in Java
In natural languages, statements and sentences are roughly equivalent. In general, statements are similar to valid English sentences. We will talk about a statement in Java and the different kinds of statements in this section.
What is the statement in Java?
A statement in Java is an executable instruction that instructs the compiler on what to do. It can contain one or more expressions and forms a complete command that can be carried out. A sentence may include one or more clauses to create a whole idea.
Types of Statements
The following categories can be used to classify Java statements:
- Expression Statements
- Declaration Statements
- Control Statements
Expression Statements
One type of statement in Java is the expression statement, typically used to produce a new value. By combining values, variables, operators, and method calls, expressions are constructed. However, a variable may occasionally be given a value by an expression.
Even though an expression usually results in something, it doesn't always. In Java, there are three different expression types:
- An expression that produces a value
- An expression that assigns a variable
- An expression that has no result
An expression that produces a value
Example 1
(5+6);
Example 2
(11-5);
An expression that assigns a variable
Example 1
result = (4 + 7);
Example 2
p = (11 - 6);
Operators used in an expression
- Arithmetic Operators: +, -, *, /, %
- Conditional Operators :||, &&, ? :
- Comparison Operators: ==, !=, > , >=, <, <=
An expression that has no result
Expressions that don't produce a result could have "side effects" because they can contain many components, like method calls or increment operators that change the program's state.
Below is an example for the expressions that have no result.
i++;
k--;
++i;
--k;
Declaration Statements
One type of statement in Java is a declaration statement, which names and declares a variable along with its data type.
Container (identifier) is the name of a variable used to store values in Java programs. A variable can have a value assigned to it at initialization and values calculated from expressions that change over time.
The variables int, boolean, and String are declared in the following sentences. These variables will be initialized with default values in this case.
int p;
int sal;
boolean isValid;
boolean isCompleted;
String eFirstName;
String eLastName;
In addition to declaring variables and types, we can initialize a statement with some literal values.
int p=20000;
int sal=50000;
boolean isValid=true;
boolean isCompleted=false;
String eFirstName="Virat";
String eLastName="Kohli";
We can also declare multiple variables of the same type on the same line, separated by commas, to reduce the number of lines of code and improve readability.
int sal, p=20000 ;
boolean isValid=true, isCompleted ;
String eFirstName="Virat", eLastName="Kohli" ;
Control Statements
The code is run from top to bottom by the Java compiler. The code's statements are carried out in the order that they appear. However, Java offers statements that can be used to manage how Java code is executed. These sentences are referred to as control flow statements. One of Java's core characteristics is ensuring a seamless program flow.
Java offers three different control flow statement types.
- Decision-Making statements
- if statements
- switch statement
- Loop statements
- do while loop
- while loop
- for loop
- for-each loop
- Jump statements
- break statement
- continue statement
Decision-Making statements
Decision-making statements, as the name implies, decide which statements to execute and when to execute them. Decision-making statements evaluate the Boolean expression and control the program flow based on the outcome of the provided condition. There are two types of decision-making statements in Java: If statements and switch statements.
if statements
The "if" statement in Java is used to evaluate a condition. Depending on the condition, the program's control is diverted. The If statement's condition returns a true or false Boolean value. There are four types of if-statements in Java, which are listed below.
- Simple if statement
- An if-else statement
- An if-else-if ladder
- Nested if-statement
Let's go through the if-statements one by one.
1. Simple if statement
It is the most fundamental of all Java control flow statements. It evaluates a Boolean expression and allows the program to proceed to the next block of code if the expression is true.
Syntax
The syntax of the if statement is shown below.
if(condition) {
statement ; //block of code is executed when the condition is met.
}
Look at the example below, where we used the if statement in Java code.
Filename: Example1.java
public class Example1 { //class name is Example1
public static void main(String[] args) {
int a = 5 ;
int b = 7 ;
if(a + b > 10) { //block is executed if the condition is satisfied
System.out.println("a + b is greater than 10") ; //prints the statement
}
}
}
Output
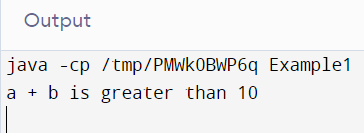
The above-displayed output shows that a + b is greater than 10.
2. An if-else statement
The if-else statement is a development of the if-statement, which employs the else block of code. If the if-condition block is deemed false, the else block is executed.
Syntax
The syntax of the if-else statement is shown below.
if(condition) {
block of code ; //block of code is executed when the condition is true
}
else{
block of code ; //block of code is executed when the condition is false
}
Look at the example below, where we used the if-else statement in Java code.
Filename: Example2.java
public class Example2 { //class name is Example2
public static void main(String[] args) {
int a = 5 ;
int b = 4 ;
if(a + b > 10) { //block is executed if the condition is satisfied
System.out.println("a + b is greater than 10") ; //prints the statement
}
else{
System.out.println("a + b is less than 10") ; //prints the statement
}
}
}
Output
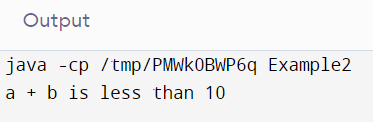
The above-displayed output shows that a + b is less than 10.
3. An if-else-if ladder
Numerous else-if statements follow the if-statement in an if-else-if statement. In other words, we can say that the series of if-else statements create a decision tree, and the program can enter the code block when the condition is true. At the end of the chain, we can also define an else statement.
Syntax
The syntax of the if-else-if ladder statement is shown below.
if(condition 1) {
block of code ; //block of code is executed when condition 1 is true
}
else if(condition 2) {
block of code ; //block of code is executed when condition 2 is true
}
else {
block of code ; //block of code is executed when the conditions are false
}
Look at the example below, where we used the if-else-if statement in Java code.
Filename: Example3.java
public class Example3 { //class name Example3
public static void main(String[] args) {
String name = "Virat" ;
if(name == "Dhoni") {
System.out.println("name of the person is Dhoni") ; //prints the statement
}else if (name == "Rohit") {
System.out.println("name of the person is Rohit") ; //prints the statement
}else if(name == "DK") {
System.out.println("name of the person is DK") ; //prints the statement
}else {
System.out.println(name) ; //prints the statement
}
}
}
Output
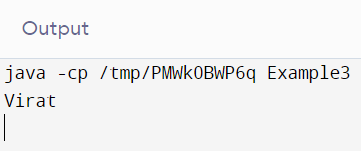
The above-displayed output prints Virat.
4. Nested if-statement
The if statement can have an if or an if-else statement inside another if or else-if statement in nested if statements.
Syntax
The syntax of the Nested if statement is shown below.
if(condition 1) {
block of code ; // block of code is executed when condition 1 is true
if(condition 2) {
block of code ; //block of code is executed when condition 2 is true
}
else{
block of code; //block of code is executed when condition 2 is false
}
}
Look at the example below, where we used the Nested if statement in Java code.
Filename: Example4.java
public class Example4 { //class name is Example4
public static void main(String[] args) {
String Cricket = "Dhoni,CSK" ;
if(Cricket.endsWith("CSK")) {
if(Cricket.contains("Jadeja")) {
System.out.println("the player is Ravindra Jadeja") ; //prints the statement
}else if(Cricket.contains("Dhoni")) {
System.out.println("the player is MS Dhoni") ; //prints the statement
}else {
System.out.println(Cricket.split(",")[0]) ;
}
}else {
System.out.println("You are not a Player in CSK") ; //prints the statement
}
}
}
Output
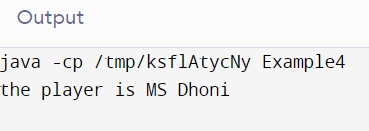
The above-displayed output displays “the player is MS Dhoni”.
Switch statement
Switch statements in Java are comparable to if-else-if statements. Depending on the variable being switched, a single case from a collection of code blocks called cases in the switch statement is executed. Instead of using if-else-if statements, use the switch statement instead. Additionally, it makes the program easier to read.
Consider the following switch statement points:
- The case variables can be an int, a short, a byte, a char, or an enumeration. Since Java 7, the String type has also been supported.
- Cases are not interchangeable.
- When any of the cases does not match the value of the expression, the default statement is executed. It is entirely optional.
- When the condition is met, the break statement ends the switch block.
If not utilized, the following case is handled instead.
- The case expression will have the same type as the variable when switch statements are used. Therefore, we must be aware of this. Even so, it will have a constant value.
Syntax
Following is the syntax for using the switch statement.
switch (expression){
case value1: //case keyword is used
statement1 ;
break ;
.
.
.
case valueN:
statementN ;
break ;
default:
default statement ;
}
Look at the example below, where we used the switch statement in Java code.
Filename: Example5.java
public class Example5 implements Cloneable { //class name is Example5
public static void main(String[] args) {
int rank = 2 ; //rank variable
switch (rank){
case 1: //case 1
System.out.println("rank is 1") ; //prints the statement
break ;
case 2: //case 2
System.out.println("rank is 2") ; //prints the statement
break ; //break statement
default: //default
System.out.println(rank) ; //prints the rank
}
}
}
Output
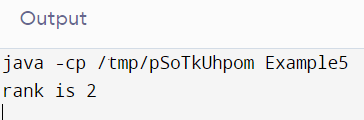
The above-displayed output displays the rank as 2.
While using switch statements, we must be aware that the case expression will share the same type as the variable. It will, nonetheless, have a constant value. Only int, string, and enum type variables can be used when the switch is active.
Loop Statements
In programming, it may be necessary to continually run a block of code while a specific condition is true. However, the set of instructions is carried out repeatedly using loop statements. The fulfilment of the given set of instructions depends upon a particular circumstance.
Three different loop types exist in Java, and they all function similarly. However, there are differences in their condition-checking times and syntax.
- for loop
- while loop
- do-while loop
Let's examine each of the loop statements individually.
Java for loop
For loops in Java are identical to those in C and C++. A single line of code allows us to initialize the loop variable, check the condition, and increment or decrement. The for loop is only used when we are certain of the number of times we want to run a code block.
Syntax
for(initialization, condition, increment/decrement) {
//block of code
}
To comprehend the proper operation of the for loop in Java, look at the example below.
Filename: Example6.java
public class Example6 { //class name is Example6
public static void main(String[] args) {
//for loop
int s = 0 ;
for(int j = 1; j<=10; j++) {
s = s + j ;
}
System.out.println("The first 10 natural numbers added together give us: " + s) ;
//prints the sum of the first ten natural numbers and stores the sum in s.
}
}
Output

The above-displayed output displays that the first ten natural numbers sum to 55.
Java for-each loop
Java offers an improved for loop for iterating through data structures like collections and arrays. We don't need to update the loop variable during the for-each loop. The for-each loop's Java syntax is provided below.
Syntax
for(data_type var : array_name/collection_name){
//block of code
}
To comprehend how the Java for-each loop functions, see the example below.
Filename: Example7.java
public class Example7 { //class name is Example7
public static void main(String[] args) {
String[] lang = {"Java","C","C++","Python","JavaScript"} ; //array elements
System.out.print("Printing the content of the array lang:\n") ;
for(String name:lang) {
System.out.println(name) ;
}
}
}
Output
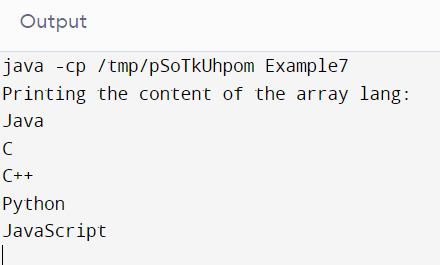
The above-displayed output prints the elements of the array- Java, C, C++, Python, and Javascript.
Java while loop
The while loop is also used to loop through the number of statements repeatedly. However, it is advised to utilize a while loop if we are unsure of the number of iterations. In contrast to for loop, while loop's initialization and increment/decrement operations do not happen inside the loop statement.
Since the condition is verified at the beginning of the loop, it is sometimes referred to as the entry-controlled loop. If the condition is satisfied, the body of the loop will be run; otherwise, the statements after the loop will be run.
Syntax
The while loop's syntax is provided below.
while(condition){
//looping statements
}
To comprehend how the Java while loop functions, look at the example below.
Filename: Example8.java
public class Example8 { //class name is Example8
public static void main(String[] args) {
int t = 1 ;
System.out.println("printing the odd numbers in a range from 1 to 10 ") ;
//prints the odd numbers
while(t<=10) { //while loop
System.out.print(t+" ") ;
t = t + 2 ;
}
}
}
Output
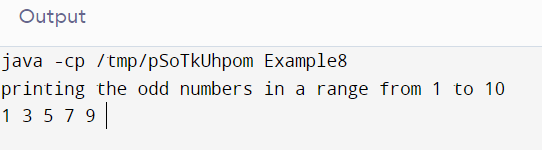
The above-displayed output displays the odd numbers from 1 to 10.
Java do-while loop
After running the loop statements, the do-while loop verifies the condition at the end of the loop. Do-while loops can be used when the number of iterations is unknown, but we must run the loop at least once.
Because the condition is not checked beforehand, it is often referred to as the exit-controlled loop.
Syntax
Below is a list of the do-while loop's syntax.
do
{
//block of code
} while (condition) ; // do-while
To comprehend how the Java do-while loop functions, see the example below.
Filename: Example9.java
public class Example9 { //class name is Example9
public static void main(String[] args) {
int p = 2 ;
System.out.println("Printing the list of even numbers in the range of 1 to 10\n") ;
//prints the list of even numbers.
do {
System.out.print(p+" ") ;
p = p + 2 ;
}while(p<=10) ; //do-while loop
}
}
Output
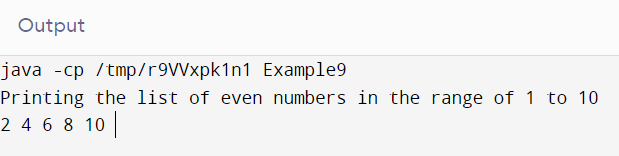
The above-displayed output shows the list of even numbers.
Jump Statements
Programming statements are typically executed sequentially. However, there are numerous Java control statements that we can use to change the flow.
Jump statements are used to pass program control to specific statements. Jump statements, in other words, move the execution control to another part of the program.
There are two types of jump statements in Java: break and continue.
Java break statement
The break statement, as the name implies, is used to interrupt the current flow of the program and transfer control to the following statement outside of a loop or switch statement. In the case of a nested loop, however, it only breaks the inner loop.
In a Java program, the break statement cannot be used independently; it must be written within a loop or switch statement.
Take a look at the example below, where both the for loop and the break statement were used.
Filename: Example10.java
public class Example10 { //class name is Example10
public static void main(String[] args) { //main method in java
for(int q = 1; q<= 10; q++) {
System.out.print(q+" ") ;
if(q==5) {
break; //break statement
}
}
}
}
Output
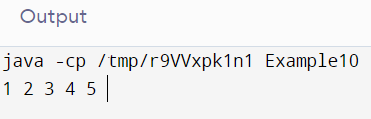
The above-displayed output shows numbers from 1 to 5.
Example of a break statement within a labelled for loop
Filename: Example11.java
public class Example11 { //class name is Example11
public static void main(String[] args) {
a: //label is used
for(int p = 1; p<= 10; p++) {
b: //label b
for(int q = 1; q<=15;q++) {
c: //label c
for (int r = 1; r<=20; r++) {
System.out.print(r+" ") ;
if(r==7) {
break a ;
}
}
}
}
}
}
Output

Java continue statement
The continue statement in Java, in contrast to break, can only be used inside loops. It does not exit the loop like a break would. Instead, it compels the loop's subsequent iteration to skip every statement that is covered by it.
To comprehend how the continue statement in Java works, consider the example below.
Filename: Example12.java
public class Example12{ //class name is Example12
public static void main(String[] args) { //main method
for(int p = 0; p<= 2; p++) {
for (int q = p; q<=5; q++) {
if(q == 3) {
continue ;
}
System.out.print(q+" ") ;
}
System.out.println("") ;
}
}
}
Output
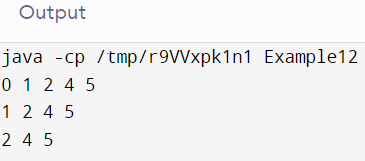
Java does not allow goto; nonetheless, it is reserved as a keyword if it were to be added in a future version.
These are the types of statements in java.