How to Split the String in Java with Delimiter
In Java, splitting strings is a significant and typically used activity while coding. Java gives different ways of dividing the String. The most widely recognized way is to use the split () technique for the String class. In this topic, we will learn how to split the String using the delimiter in Java. Similarly, we use the String Tokenizer and Scanner classes to split the String.
For splitting the String in Java with a delimiter. Firstly, we have to know what delimiter is.
In Java, the delimiter is the character that divides or splits the given String into tokens. It provides us to understand any character defined in Java as the delimiter. In Java, there are many splitting methods are there. One of the methods is the white space character, and this character is considered the default delimiter.
Before going to the programming code, let's see the theory of the given concept.
String: As we know, String is the Set of characters stored in the quotes. I.e., “java”. In Java, the String is provided with two types.
They are tokens and delimiters. Whereas the tokens are knowns as the words which make meaningful sense, the delimiters are known as the characters that divide or split into the tokens.
Let's see this topic in more detail with the examples:
To deal with the delimiters in Java, we must know the basics of Java regular expressions. It is significant whenever the delimiter is performed as the special characters like [.], [,], [|].
Example: Let's see the example for delimiter
Consider a string as Google is a search engine.
From the above String, the tokens we consider are Google, is, search, engine, and the delimiter we performed here are the white spaces between the two tokens.
How to Split a String in Java with Delimiter?
For splitting the String in Java, it provides the three methods:
- Using Scanner.next() Method
- Using String.split() Method
- Using StringTokenizer Class
- Firstly, let's see about the Scanner.next () Method:
Scanner.next() method is one of the scanner classes. This method finds the token and returns it to the next token from Scanner. It is used to separate or split the String into the tokens using a delimiter. The total token is considered by the info that matches the delimiter design.
Now let's see a simple example:
import java.util.Scanner;
public class Demo3
{
public static void main(String args[])
{
Scanner scan = new Scanner("Google/Is/The/Search/Engine");
scan.useDelimiter("/");
while(scan.hasNext())
{
System.out.println(scan.next());
}
scan.close();
}
}
Output:
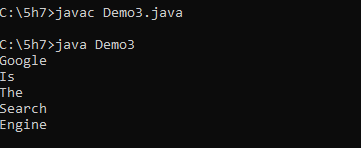
The String has been divided into tokens with delimiters in the above program.
1. The second method is Using String.split() Method:
The split () method for the String class is used to part a string into a variety of String objects given the particular delimiter that matches the ordinary expression.
String str= "java,is,platform,dependent,programming,language";
The commas split the above String. For splitting, we use the following expression in our code.
Syntax:
String [] tokens=sc.split(",");
For the above syntax, the String has been divided into tokens with special delimiter characters like comma (,).
Syntax:
public String[] split(String regex);
This method is used to pass the delimiter for the regular java expressions. For this program
It raises the exception. If the invalid syntax has presented in the program, it throws the exception called pattern syntax expression.
Let's see a simple code for the following syntax:
import java.util.Scanner.*;
public class Demo3
{
public static void main (String args[])
{
String s = "java,is,platform,dependent,programming,language";
String[] stringarray = s.split(",");
for(int i=0; i< stringarray.length; i++)
{
System.out.println(stringarray[i]);
}
}
}
Output:
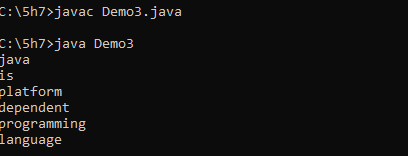
2. Now let's see with the third method using StringTokienizer Class:
As we know, that java StringTokienizer is the Superclass which is defined in the util.Scanner package. Mostly we use the split () method rather than the StringTokienizer class in the programming language.
Now let's see the simple code for StringTokienizer class:
import java.util.StringTokenizer;
public class Demo3
{
public static void main(String[] args)
{
String str = "java/is/object/oriented/programming/language";
StringTokenizer tokens = new StringTokenizer(str, "/");
while (tokens.hasMoreTokens())
{
System.out.println(tokens.nextToken());
}
}
}
Output:
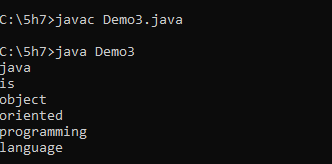
These three methods are to split the String in Java using the delimiters.