Copy data/content from one file to another in java
In this article, you will be acknowledged about how to copy data or content from one file to another file. Also, you will be acknowledged about the classes and methods leveraged in the process.
The content of one file can be copied to another file in Java. The FileInputStream as well as FileOutputStream classes are designed to handle this.
FileInputStream
One of the most popular and significant byte input stream class in Java is FileInputStream. It is mostly used to read data bytes from files. There are numerous methods available in the FileInputStream class for retrieving data from files.
The following syntax creates a new instance of a FileInputStream class:
FileInputStream f = new FileInputStream(file name);
The method constructor receives the file name that we want to retrieve data from. For replication of data from a file to another, we should use following two FileInputStream class methods.
- read()
- close()
read()
When extracting data from the file, the read() method is crucial. When its pointer approaches the file's end, it returns -1 and returns bytes of data as an integer.
close()
The FileInputStream class instance is closed using the close() function.
FileOutputSream
One of the most popular and significant byte output stream classes within Java is FileOutputStream. It is mostly employed to write bytes of data into files. There are numerous methods for transmitting the data into the file provided by the FileOutputStream class.
The following procedure is used to generate a FileOutputStream class instance:
FileOutputStream f= new FileOutputStream(file name)
The method constructor receives the file name that we wish to write data to. For replication of data of one file to another, we should use following two FileOutputStream class methods.
- write()
- close()
write()
When writing data (bytes of data) into the file, the write() method is crucial.
close()
The FileOutputStream class instance is closed using the close() function.
File
When writing or reading bytes of data from a file, the File class is employed to build an object of the file. The process used to generate a File class instance is as follows:
File fileobj = new File(file name);
Note: The File class shall generate a new file with the specified name if it does not already exist.
Let us understand how all of this will contribute to the replication pf data from one file to another.
File name: Copydata.java
import java.io.*;
import java.util.*;
public class CopyFromFileaToFileb {
public static void copyContent(File a, File b)
throws Exception
{
FileInputStream in = new FileInputStream(a);
FileOutputStream out = new FileOutputStream(b);
try {
int n;
// read() method to obtain the data from the given file
while ((n = in.read()) != -1) {
// write() method to retrieve the data to another file
out.write(n);
}
}
finally {
if (in != null) {
// close() method is used to close the stream
in.close();
}
// close() method is used to close the stream
if (out != null) {
out.close();
}
}
System.out.println("File Copied");
}
public static void main(String[] args) throws Exception
{
Scanner sc = new Scanner(System.in);
// obtains the name of the source file
System.out.println(
"Enter the name of the source file from which you wanted to copy the content or simply paste the file name :");
String a = sc.nextLine();
//to take source file
File s = new File(a);
// to get the file destination file
System.out.println(
"Enter the name of the destination file in which you want to copy the data or simply paste the file name :");
String b = sc.nextLine();
// to produce the destination file
File d = new File(b);
// method invoked to copy the data from the file
copyContent(s, d);
}
}
Output:
Enter the source filename from where you have to read/copy :
source.txt
Enter the destination filename where you have to write/paste :
Destination.txt
File Copied
Before running the above program.
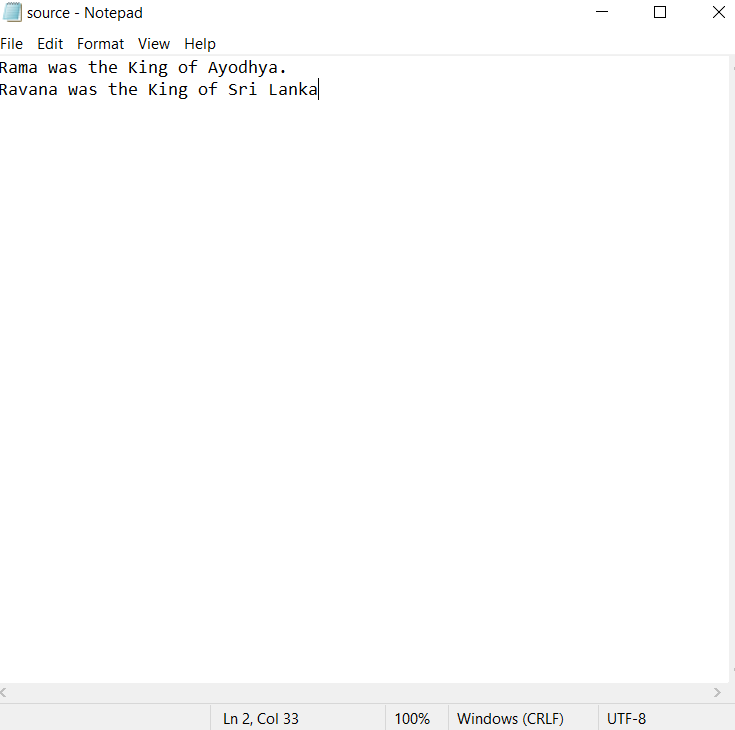
This is the screenshot of the source file with some text inside it.
This is the screenshot of the destination file with no content in it and before running the above program.
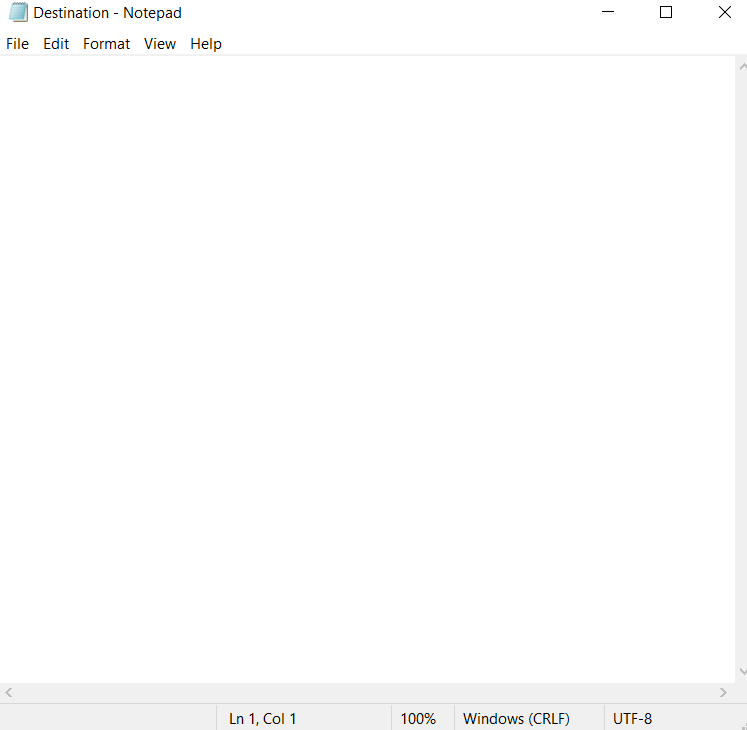
After successfully executing the above program.
This is the screenshot of the source file after running the program with some text in it.
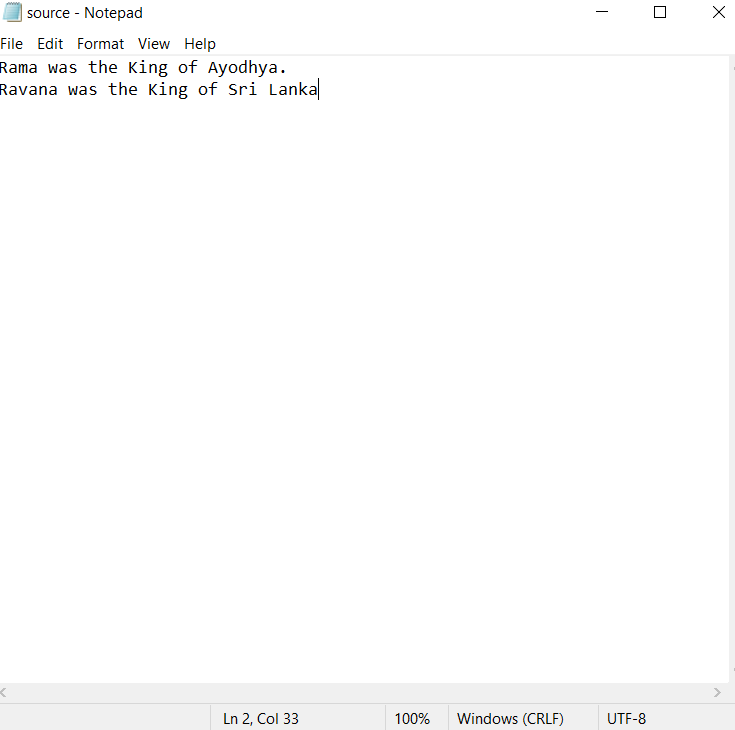
This is the screenshot of the destination file with copied content from the source file
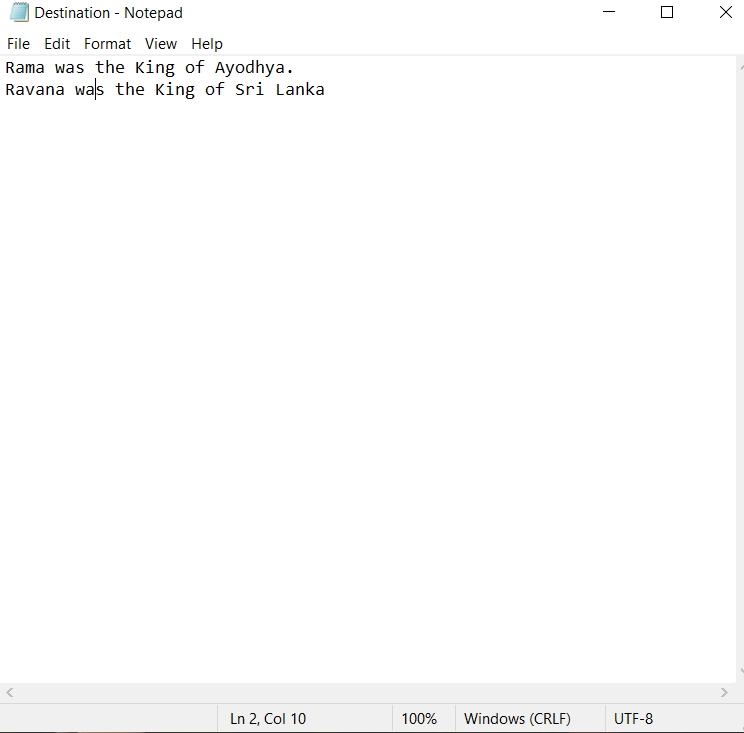