Java Trim
Leading and leaving spaces are removed by the built-in Java String trim() method. Space seems to have the Unicode element of "x0040." Java trim() function looks for all of these Unicode results before and after text, removes the spaces if they are present, and then returns the string that was omitted. In Java, trimming characters is also facilitated by the trim() function. To remove every preceding and trailing whitespace from a text in Java, use the trim() method. It is described in the Java.lang package String class.
It needs to be noted as the important point that:
Note: The intermediate spaces are not removed by the trim() technique.
Method Signature
The following is the syntax or signature of the trim() function for the String class:
public String trim()
Parameters: No parameters are accepted by the trim() function.
Return: trim() returns a String as its return type. No preceding or trailing spaces are included in the omitted string that is returned. Missing preceding and trailing spaces in a string.
Lets us have a look at how the trim method is implemented internally.
public String trim() {
int len = values.length;
int str = 0;
char[] valu = values; /* the getfield opcode should be avoided */
while ((str < len) && (valu[str] <= ' ')) {
str++;
}
while ((str < len) && (valu[len - 1] <= ' ')) {
len--;
}
return ((str > 0) || (len < values.length)) ? substring(st, len) : this;
}
The Java string trim() function is demonstrated below with the help of certain examples.
Example 1:
public class StringTrimDemo1{
public static void main(String args[]){
String s3=" hiii kamal ";
System.out.println(s3+"Koushik"); //without using the trim() method
System.out.println(s3.trim()+"Koushik"); //with the use of trim() method
}}
Output:

Example 2:
Let us see another example for the trim() method.
The trim() method is used in the example to show how it works. By eliminating all trailing spaces, this technique shortens the string. Here's an illustration.
StringTrimDemo2.java.
// Java programme to show how the
// Java string trim() technique works
import java.io.*;
class StringTrimDemo2 {
public static void main (String[] args) {
String s4 = " Sahithi the senior ";
// Before using of the trim() method
System.out.println("Before the Trim() method - ");
System.out.println("String - "+s4);
System.out.println("Size - "+s4.size());
// using the trim() method on the s4 string
S4=s4.trim();
// After using the Trim() method
System.out.println("\nAfter the Trim() method - ");
System.out.println("String - "+s4);
System.out.println("Size - "+s4.size());
}
}
Output:
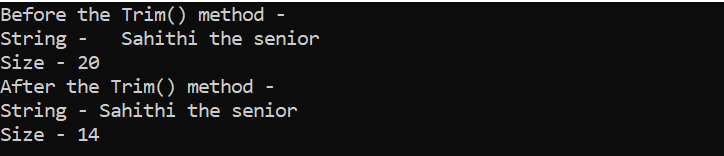
Example 3:
To determine whether a string contains only white spaces or not, use the trim() method. The identical thing is demonstrated in the example below.
StringTrimDemo4.
public class StringTrimDemo4
{
// it is the main method
public static void main(String argvs[])
{
String sr = " xyz ";
if((sr.trim()).length() > 0)
{
System.out.println("The string includes characters besides blank spaces \n");
}
else
{
System.out.println("There are just white spaces in the string \n");
}
sr = " ";
if((sr.trim()).length() > 0)
{
System.out.println("The string includes characters besides blank spaces \n");
}
else
{
System.out.println("There are just white spaces in the string \n");
}
}
}
Output:

Example 4:
Although strings through Java are immutable, the trim() method's manipulation of a string by removing whitespace results in a new string is returned. The reference to the same text is returned if the alteration is not carried out by the trim() method. Consider the example that follows.
Let the file name of the program is StringTrimDemo9.
public class StringTrimDemo9
{
// it is the main method
public static void main(String argvs[])
{
// the string has blank spaces in it
// reducing the spaces. As a result results in the
// creation of a new string
String sr = " xyz ";
// sr4 stores a new string
String str4 = sr.trim();
// Different hashcodes exist for sr and sr4.
System.out.println(sr.hashCode());
System.out.println(sr1.hashCode() + "\n");
// there is no white space in the string p.
// the s reference is returned as a result
// after calling the trim() method
String p = "xyz";
String p1 = p.trim();
// p and p1 share the same hashcode.
System.out.println(p.hashCode());
System.out.println(p1.hashCode());
}
}
Output:
