Converting Long to Date in Java
What Long and Date are in Java and how are they implemented in the Java programming language are the topics of this article. Additionally, we'll go into great detail on how to change a Long value in Java into a Date value. Let's now get into the specifics of the article.
What is Long in Java?
A simple data type, the long keyword is used in the Java programming language. In Java, the long keyword is used to declare large integer variables. A variable declared with the long keyword has an 8-byte default size and the value 0L by default. The long keyword may also be combined with methods. Let's now use a Java programming example application to understand the long data type.
Long is a primitive data type in Java. To declare variables, use this. It can also be applied to methods. A 64-bit two's complement integer can fit within it.
Remember:
- A long's minimum value is -263 and its greatest value is 263-1.
- With the introduction of Java 8, the long can now be represented as an unsigned 64-bit long with a minimum value of 0 and a maximum value of 264-1.
- The default setting is 0L.
- It is an 8-byte default size.
- When a larger range of integer values is required, it is employed.
Program to determine the usage of long
Filename: Example1.java
//Using the Java programming language, this program
//demonstrates how the "long" data type functions.
public class Example1 {
public static void main(String args[]) {
//two variables are declared using the long data type.
long S = 150000L ;
long V = -1500000L ;
// printing the values of long data types S and V.
System.out.println("The value of S is: " + S) ;
System.out.println("The value of V is: " + V) ;
}
}
Output
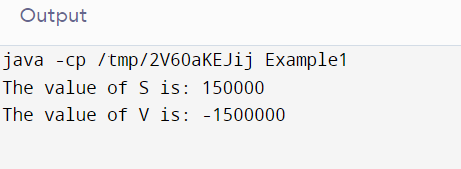
What is the Date in Java?
The Date is a class used to represent a particular moment in time. The Java Date class allows for millisecond-level precision when representing time. The "java.util" package contains the Date class, which provides a serializable, comparable, and cloneable interface. When dealing with date and time in Java, the Date class can be utilized using constructors and methods. The Date class uses a number of different constructors. Let's look at a few of the Date class's constructors.
How to convert Long to Date in Java?
We will start by providing an input of milliseconds, which is regarded as belonging to the "long" data type. Therefore, it is necessary to write a program that translates milliseconds that are initially in "long datatype" format into "date" format. The format used to display the date and time is often "dd Mmm yyyy HH: mm: ss: SSS Z," where "dd" stands for the date, "Mmm" for the month name's first three characters, and "yyyy" for the year. "HH" stands for the hour, "mm" for the minute, "ss" for the second, "SSS" for the first three digits of the millisecond, and "Z" stands for the time zone, These all relate to the date.
The milliseconds are determined using the date "1 January 1971," as it is when the idea of "date class" was developed and put into use. A constructor that assists the system in converting milliseconds into dates is offered by the "date" class. We can convert dates into the necessary format, i.e., " dd Mmm yyyy HH: mm: ss: SSS Z ", with the aid of the class " SimpleDateFormat ". Internally, the "SimpleDateFormat" class has a small number of constructors that handle formatting depending on the quantity and kind of parameters given. The class's constructors will be covered in detail below.
Constructors of SimpleDateFormat class:
Three distinct constructors are divided into categories based on the number of parameters and type of parameters passed. As follows:
- SimpleDateFormat( String pattern_arg )
- SimpleDateFormat( String pattern_arg, Locale locale_arg )
- SimpleDateFormat( String pattern_arg, DateFormatSymbols formatSymbols)
SimpleDateFormat( String pattern_arg ):
The pattern "pattern arg" often creates a standard and Simple Date format that activates the default date format symbols for the default FORMAT locale.
SimpleDateFormat( String pattern_arg, Locale locale_arg ):
While enabling the default date format symbols for FORMAT Locale " locale arg ", it often builds a Simple Date format in the pattern " pattern arg ".
SimpleDateFormat(String pattern_arg, DateFormatSymbols formatSymbols):
Typically, it creates a Simple Date format with any date format symbol enabled using the pattern "pattern_arg".
These three constructors provide a simplified example of the date format. Let's implement a handful of these constructors and create a program using the formatting class.
Program 1: demonstrates the conversion of milliseconds to Date:
Filename: Example4.java
//Java program that changes
//milliseconds into date format
//to meet the criteria,
//import the necessary packages
import java.util.Date ;
import java.text.DateFormat ;
import java.text.SimpleDateFormat ;
// main class
public class Example4
{
public static void main(String args[])
{
//assigning a variable that
//stores the millisecond’s value
long x = 3000000000000L ;
//starting the date format where
//the conversion needs to be done
DateFormat obj = new SimpleDateFormat("dd MMM yyyy HH:mm:ss:SSS Z") ;
// Creation of date from milliseconds
// using the " Date " constructor
Date sol = new Date(x) ;
// Formatting Date according to the
// given format
System.out.println(obj.format(sol)) ;
}
}
Output
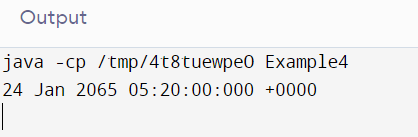
Program 2: demonstrates the conversion of milliseconds to Date:
Filename: Example5.java
//Java program that changes
//milliseconds into date format
//to meet the criteria,
//import the necessary packages
import java.util.Date ;
import java.text.DateFormat ;
import java.text.SimpleDateFormat ;
// main class
public class Example5
{
public static void main(String args[])
{
//assigning a variable that
//stores the millisecond’s value
long x = 409063289456L;
//The date format that must be used
//for the conversion is being initiated.
DateFormat obj2 = new SimpleDateFormat("dd MMM yyyy HH:mm:ss:SSS Z") ;
// Creation of date from milliseconds
// using the " Date " constructor
Date sol2 = new Date(x) ;
// Formatting Date according to the
// given format
System.out.println(obj2.format(sol2)) ;
}
}
Output
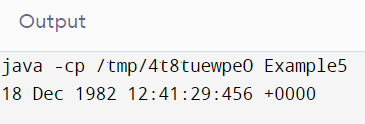