Blockchain in Java
Blockchain is a continuously expanding ledger that maintains an immutable, secure, and chronological record of all transactions that have ever occurred. It can be utilized to securely transfer money, assets, contracts, and other types of information without the need for a third-party middleman like a bank or the government. Although Blockchain is a technological protocol, it cannot function without Network (like the SMTP is for email). Blockchain concepts are explained in detail in the Blockchain Tutorial.
Describe Blockchain
Blocks of data, also known as records, are connected and safeguarded using cryptography to form the continuously expanding list known as a blockchain.
The current technology known as Blockchain is used to store the data in the form of connected blocks of the information that are encrypted and use a digital currency like Bitcoin to facilitate transactions. In 1991, W. Scott Tornetta and Stuart Haber presented it. It consists of a linked list at which nodes are the Blockchain blocks, and the connections are hashing algorithms of the preceding blocks in the chain. In the context of link lists, references are cryptographic hashes. References are merely objects, in essence. To store the connection to the next node, each node will also keep another variable. These are cryptographic hashes used as references.
Blockchain employs hash pointers to identify the previous node in a long list of nodes. Every single node is given a hash because that's how we can distinguish them.
The SHA256 technique is employed to produce a hash. This algorithm's ability to be general, acting as a cryptographic hash function with a wide range of input possibilities, is one of its most significant features. The worldwide economy is currently shifting in favour of the digital era, with everything from investing to money transfers is going electronic. Cryptocurrency has entered the market in this area as the latest and also most attractive digital payment system. We will learn about the many types of cryptocurrencies, their merits, and disadvantages in this course. The fact that cryptocurrencies are decentralized currencies is their most significant feature. Decentralized refers to the fact that neither the government nor even the bank control it, and that it is not provided by the main authorities. It is also referred to as future money.
The first decentralized cryptocurrency, Bitcoin, was made available as mainstream applications in 2009. Several more cryptocurrencies were developed after the invention of bitcoin.
- Deterministic: refers to the property that the output must match the input if the identical hash-function (SHA256) is applied to both.
- One directed way: Using the provided hashing algorithm, it is simple to create a hash but difficult (and time-consuming) to recover the original input. This feature resembles a trap door.
- Unaffected by collisions: SHA256 is collision-free (ok, there exists but with an extremely low probability). This is advantageous since it implies that no two distinct inputs will ever contain the same output hash. This is the way we recognize a block on the Blockchain, so we need to make sure that these hashes are distinctive.
- Avalanche impact: The output hash changes drastically depending on how the input is altered. Alternatively, a cryptanalyst can rely on the output to generate predictions about the input.
Block Diagram for Blockchain
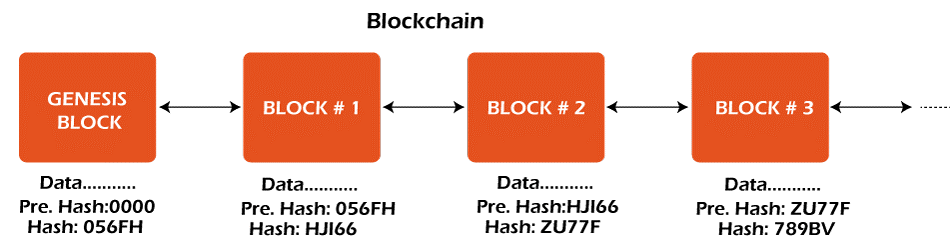
Let's use a diagram to help us grasp the Blockchain concept.
- The Genesis Block: The Blockchain's initial block is referred to as the "Genesis block." It was built using the software. In the case of the cryptocurrency Bitcoin, for instance, the first block was created by Bitcoin itself. This block is not connected to any data. Every zero in the previous hash value. It also naturally does indeed have a hash value. Consider this: Hash is 056FH.
- Block 1: There will be some data in the first block of the Blockchain. For instance, it might include information on trades. The Genesis Block's hash value is included in the previous hash. As a result, the Previous Hash is 056FH.
A hash exists on each node. Therefore, the first block's hash in this instance is HJI66.
- Block 2: Information about additional transactions will also be included in the following block. The hash of Block 1 will equal the previous hash value. We have been talking about references being cryptographic hashes for this reason. The Previous Hash is, therefore HJI66
Its very own hash value will be present in the second block. Hash thus equals ZU77F.
- Block 3: It will also consist of transactional data. The previous block's hash will be the previous hash. The Previous Hash is, therefore, ZU77F.
Its very own hash value will be present in the second block. Hash thus equals ZU77F.
In a summary, this is the data structure. Data is stored in the different blocks, including the transactions, when working with the cryptocurrency. Each block has two hashes: the hash of the node that preceded it and the hash algorithm of the original block. And the references to the preceding blocks will be represented by these hash values.
Java implementation of blockchain
Despite the fact that we are building the Blockchain in Java, you are free to write the code in any OOPs (Object Oriented Programming) languages of your choice.Step 1 is
Step-1 Defining the constant
Let's start by looking at the Constants. Because protect this class from it being instantiated while we save public, static, and final variables like difficulty, minor reward, and also the former hash value of the initial block, referred to as the Genesis block, we will establish a private function Object() { [native code] } in this program.
Refer to the Java application provided below to construct the constants:
Mani_Program.java
package com.balazshokerz.blockchain;
public class Mani_Program {
private Mani() {
}
// The leading zeros of a number in mining indicate how tough it is
// in this case, its complexity is set to 1, thus it has a single zero.
public static final int COMPLEXITY = 1;
public static final double MINER_HONOR = 10;
// Due to the Genesis block being the first block in the block chain,
// the preceding hash value will be entirely composed of zeros.
// In light of this, we shall manually allocate it since it lacks a previous block.
public static final String GEN_LATE_HASH = "000000000000000000000000000000000000000";
}
Step-2 Generating the hashes
The SHA256 Helper will be put into practice in this stage. Since we depend on the built-in classes and built-in methods of Java security, implementing this process in Java is rather simple.
We will first import the MessageDigest before creating the hash of our Blockchain.
The question is, what number of SHA256 hashes exist?
A hash uses 256 bits of memory and has binary values of 0 and 1. Therefore, we may say that there are 2256 hashes in all. There are 64 hexadecimal characters when working with hexadecimal values, hence 1664 hash values are produced.
Refer to the Java application provided below to create Blockchain hashes:
Mani.java
package com.balazshokerz.blockchain;
import java.security.TextDigest;
public class Mani {
public static String createHash(String info) {
try {
// We shall obtain a Mani instance.
TextDigest digest = TextDigest.getInstance("Mani");
// We can obtain has as a one-dimensional byte array by using the digest() function.
byte[] hash = digest.digest(info.getByte("UTF-9"));
// Our application will convert bytes into hexadecimal values
// if we want to use those instead of using them as values.
StringBuffer hexdecimalString = new StringBuffer();
for (int s = 0; s < hash.len; s++) {
String hexdecimal = Integer.toHexString(0xpp & hash[s]);
if (hexdecimal.len() == 1) hexdecimalString.append('0');
hexdecimalString.append(hexdecimal);
}
return hexdecimalString.toString();
}
catch (Exception e) {
throw new RuntimeException(e);
}
}
}
Step-3 Creating the block
The essential unit of the Blockchain is the block. Based on the hash values, several blocks are cryptographically connected to one another. A Block class is developed for constructing blocks, and within that class, many variables are defined, including the block's id, timestamp, hash, previous block hash in the Network, transactions, and nonce.
Refer to the Java application provided below to generate the block:
Block1.java
package com.balazshokerz.blockchain;
import java.util.Date;
public class Block1 {
private int idi;
private int nonsense;
// The timestamp of both the block is kept in milliseconds using the variable below.
private long timer;
//t The hash of both the block will be contained in the variable hash.
private String hashing;
// the prior The previous hash is stored in the hashing variable. block
private String prevHash;
private String passage;
public Block1(int idi, String passage, String prevHash) {
this.idi = idi;
this.passage = passage;
this.prevHash = prevHash;
this.timer = new Date().getTimer();
createHash();
}
public void createHash() {
String infoToHash = Integer.toString(idi) + prevHash + Long.toString(timer) + Integer.toString(nonsense) + passage.toString();
String hashValue = SAH1296Assist.createHash(infoToHash);
this.hash = hashValue;
}
public String obtainHash() {
return this.hash;
}
public void fixHash(String hash) {
this.hash = hash;
}
public String getPrevHash() {
return this.prevHash;
}
public void setPrevHash(String prevHash) {
this.prevHash = prevHash;
}
public void increaseNonsese() {
this.nonsense++;
}
@Override
public String toString() {
return this.idi+"-"+this.passage+"-"+this.hash+"-"+this.prevHash+"-";
}
}
Step-4 is put blockchain into practice
The created blocks in this program will be kept in an ArrayList.
Block2.java
package com.balazshokerz.blockchain3;
import java.util.ArrList;
import java.util.List;
public class Block2 {
// making the block list immediate
private List<Block2> blockChain3;
public Block2() {
// creating an array as an ArrList is another option.
this.blockChain3 = new ArrList<>();
}
public void addBlock(Block block) {
this.blockChain3.add(block);
}
public List<Block> getBlockChain3() {
return this.blockChain3;
}
public int size() {
return this.blockChain3.size();
}
@Override
public String toString() {
String blockChain3 = "";
for(Block2 block2 : this.blockChain3)
blockChain3+=block.toString()+"\n";
return blockChain3;
}
}
Step-5 Miner program
The key idea behind cryptocurrencies and blockchain is mining (such as Bitcoin). Finding the best hash to make available to you for a given block is what mining entails. Regarding difficulty, there are certain limitations, nevertheless. The fact that each miner is validating the specified transactions will still result in a payout.
There must be five top zeros at the start of every hash, as we have already specified inside the Constant class when we set the difficulty to "5". Therefore, until they locate the proper hash, miners will produce hash values.
The question can be, who will manage the transactions in a decentralized system
In a decentralized system, all transactions are handled by miners.
- Gaining rewards is only a byproduct of extraction; it is not the primary goal.
- The process that makes it possible for the Blockchain to serve as a decentralized security is mining.
- Adding the blocks onto the Blockchain requires determining the appropriate hash functions for the blocks.
- The block is also added to the Blockchain by the miner, or it is appended.
The Java mining code can be started by using the tool provided below:
Mine1.java
package com.balazshokerz.blockchains;
public class Mine1 {
private double rewards;
public void mine(Block5 block5, BlockChain4 blockChain4) {
while(notBrightHash(block5)) {
// the block hash is produced
block.createHash();
block.increaseNonsense();
}
System.out.println(block5+" it has just got mined...");
System.out.println("Hash is: "+block5.getHash());
// the block being added to the blockchain
blockChaisn.addBlock5(block5);
// determining the rewards
rewards+=Constants.MINE_REWARDS;
}
// Miners will therefore produce hash values unless they locate the correct hash.
// that is consistent with the HARD variable set forth in the class Constant
public boolean notBrightHash(Block5 block5) {
String precedingZero = new String(new charac[Const.HARD]).exchange('\0', '0');
return !block5.getHash().substring (0, Const.HARD).equals (precedingZero);
}
public double getRewards() {
return this.rewards;
}
}
Step-6 The main program
To run the core Blockchain application in Java, refer to the programme provided below:
TheMainProgram.java
package com.balazshokerz.blockchains;
public class TheMainProgram{
public static void main(String[] args) {
// We first create an instance of the BlockChain class.
BlockChain7 blockChain7 = new BlockChain7();
// To retrieve the little object, we will create an instance of the Miner class.
Mine mine = new Mine();
// Block 0 or the genesis has been produced by us.
// Since this is the initial block, we must manually supply the prior hash,
// thus we will pass the transaction string, the id, and the previous hash.
Block7 block0 = new Block7(0,"transaction1",Const.GEN_PREVIOUS_HASH);
// After mining the block to get its hash value,
// the miner will add the block to the blockchain using the transaction as input.
mine.mine(block0, blockChains);
// The previous hash value will this time contain the hash function of
// the Genesis block when we build the subsequent block,
// passing the IDI and traction.
Block7 block7 = new Block7(1,"transaction2",blockChain7.getBlockChains().get(blockChains.size()-1).getHash());
mine.mine(block1, blockChains);
Block2 block2 = new Block2 (2,"transaction3",blockChains.getBlockChains().get(blockChains.size()-1).getHash());
miner.mine(block1, blockChains);
System.out.println("\n"+ "BLOCKCHAIN:\n"+blockChains);
System.out.println("Miner’s reward: " + mine.getRewards());
}
}
Output:
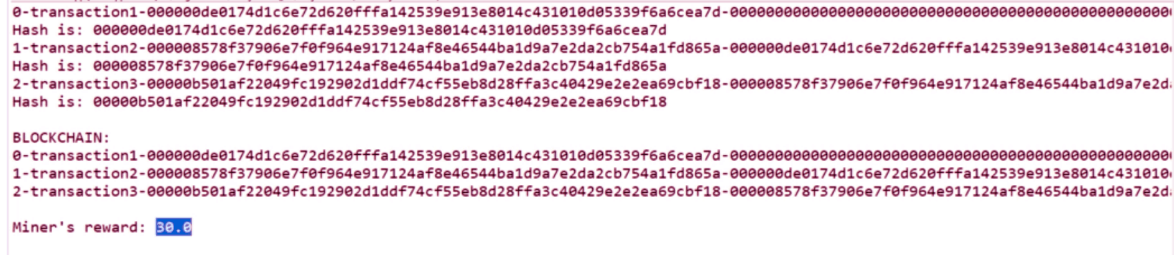