Java Type Casting
Type casting is a technique or process used in Java to convert one data type into another, either manually or automatically. The compiler performs the automatic conversion, and the programmer handles the manual conversion. With appropriate examples, we will discuss type casting in this section.
Type casting is divided into the following types. They are
- Widening Type Casting
- Narrowing Type Casting
Widening Type Casting
Widening type casting refers to the transformation of a lower data type into a higher one. Casting down and implicit conversion are other names for it. It happens on its own. It is secure because there is no possibility of data loss. The event occurs when:
- It is necessary for both data types to be compatible.
- The target type must be larger than the source type.
Byteà short à char à int àlong àfloatà double
There is no automatic conversion from a numeric data type to a char or boolean data type. Additionally, the data types char and boolean are incompatible with one another.
Consider the following example.
TypeCast.java
import java.io.*;
import java.util.*;
class TypeCast
{
public static void main(String[] args)
{
Scanner sc = new Scanner(System.in);
System.out.println("enter the value of n");
int a = sc.nextInt();
//converts the integer into long
long b = a;
//converts the long into float
float c = b;
System.out.println("Before conversion, a= "+a);
System.out.println("After conversion, b= "+b);
System.out.println("After conversion, c= "+c);
}
}
Output:
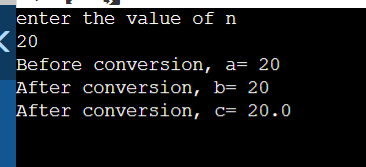
TypeCast1.java
import java.io.*;
import java.util.*;
class TypeCast1 {
public static void main(String args[]){
Scanner scan = new Scanner(System.in);
System.out.println("Enter an character value: ");
char n = scan.next().charAt(0);
int c = n;
System.out.println("converted integer value of the character is: "+c);
}
}
Output:

Narrowing Type casting
Narrowing type casting in Java refers to the conversion of a higher prioritized data type to the lower one. In this type, type casting is not done directly. It is done explicitly with the help of the cast operator.
The order of type casting is as follows,
Doubleà floatàlongàintàcharàshortàbyte
Example program:
Conversion of int to char
TypeCast2. java
import java.io.*;
import java.util.Scanner;
class TypeCast2 {
public static void main(String args[]){
Scanner scan = new Scanner(System.in);
System.out.println("Enter an integer value: ");
int n = scan.nextInt();
char c = (char) n;
System.out.println("converted charater value of the integer is: "+c);
}
}
Output:
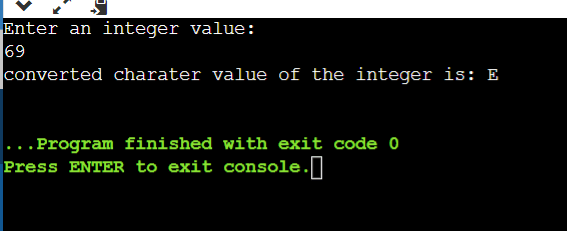
Conversion of char to byte
TypeCast3.java
import java.io.*;
import java.util.*;
class TypeCast3 {
public static void main(String args[]){
Scanner scan = new Scanner(System.in);
System.out.println("Enter a Character value: ");
char n = scan.next().charAt(0);
byte c = (byte) n;
System.out.println("converted byte value of the character is: "+c);
}
}
Output:
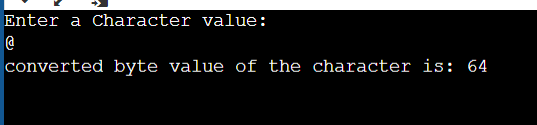
Conversion of double to byte:
TypeCast4. java
import java.io.*;
import java.util.*;
class TypeCast4 {
public static void main(String args[]){
Scanner scan = new Scanner(System.in);
System.out.println("Enter a Character value: ");
double n = scan.nextDouble();
char c = (char) n;
System.out.println("converted byte value of the character is: "+c);
}
}
Output:
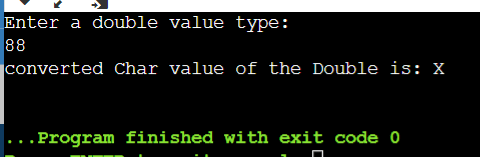