File Operation in Java
In Java, a file is an Abstract data type. These are used to store the data which is related. Files are named storage locations. With a file we can perform different types of operations.
File Operations
Operations mean performing a task. So, in the file, we perform certain operations. So, there are 5 operations that must be performed on a file. They are
- Creating a file
- Get file information
- Write to a file
- Read from a file
- Delete a file
Reading from and writing to files is an important aspect of any programming language not only Java.
Creating a file
By the name of the operation, we can come to know that this operation is used for creating a new file. We can use the “ file class “ in Java to create a file. We need to import the file class for creating a new file. For creating a file in Java we use the " createNewFile() " method. We get true if the file is successfully created. False is returned when the file is already present on the pc.
By using an example, you can easily understand how this method which is the createNewFile() method is used in creating a file in Java.
CreateNewFileExample.java
import java.io.File; // File class has been imported
import java.io.IOException; // IO Exception class has been imported to handle the unexpected errors
class CreateNewFileExample {
public static void main(String args[]) {
try {
File f1 = new File("d:FileOperationExample.txt"); // an object is created for the file
if (f1.createNewFile()) { // using createNewFile method
System.out.println(" File " + f1.getName() + " is successfully created in the directory . "); // printing the status
} else {
System.out.println(" Sorry ”);
}
} catch (IOException exception) { // exception
System.out.println(" Unfortunately an error has been occurred. ");
exception.printStackTrace();
}
}
}
Output:

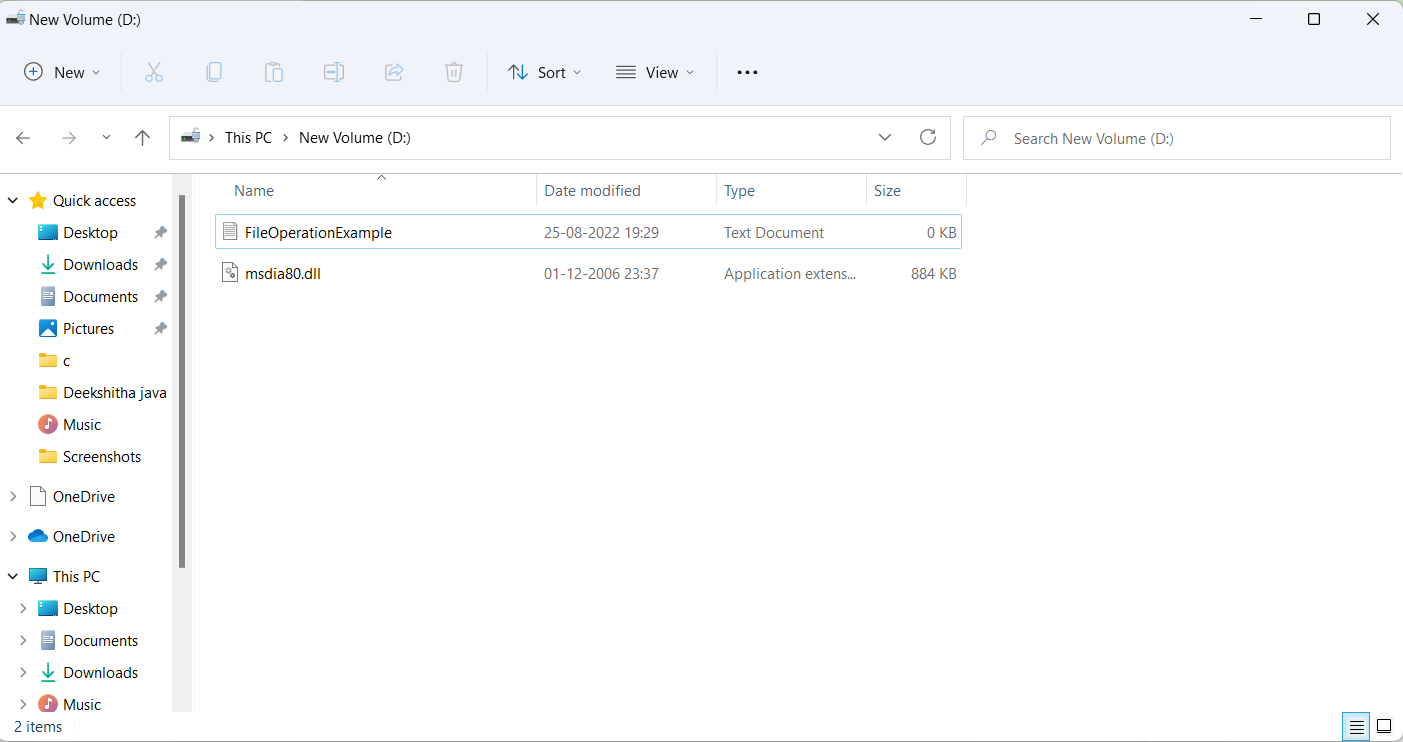
Code Explanation:
Firstly, we have imported the file class as well as the IO Exception class. As discussed earlier the File class is used to create a file. And the IO exception class is used to handle the errors. Next, we have created an object for the file class that is f1. And, we have specified the directory location. By using the createNewFile() method in the try block we have created a new file in the d directory. This program will return success if the file is created successfully. Or else it will give output as a file already existed if the file is already there in the directory. If there are any errors, then an unexpected error is given as output.
Get file information
When we create a new file, we want to know about the file information like the name of the file, the location in which directory it is located, information like the length of the file, and many more. So, to get the file information this operation is used.
Here let us understand how the file class methods are used to get the information from the respective file.
GettingFileInfoExample.java
import java.io.File; // file class has been imported
class GettingFileInfoExample{
public static void main(String[] args) {
// Creating object f1 for the file class
File f1 = new File("D:FileOperationExample.txt");
if (f1.exists()) {
// Using the getName() method
System.out.println(" The name of the file is: " + f1.getName());
// using the getAbsolutePath() method
System.out.println(" The absolute path of the file is: " + f1.getAbsolutePath());
// using the canWrite() method
System.out.println(" Is the file writeable ?: " + f1.canWrite()); // true
// Using the canRead() method
System.out.println(" Is the file readable ? " + f1.canRead()); // true
// Using the length() method to find the length of the file
System.out.println(" File size is : " + f1.length()); // 0
} else {
System.out.println(" Sorry, the file of which you want to get the information does not exist. ");
}
}
}
Output:
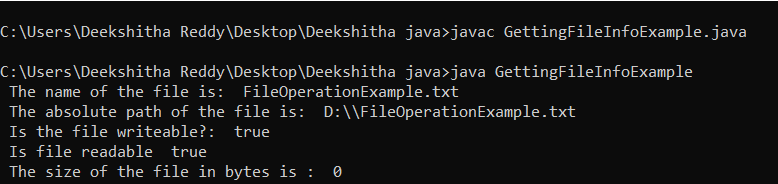
Code explanation:
Here we have first imported the file class and then created a class that is GettingFileInfoExample. In the main method, we have created an instance that is object f1 for the file class. Next, we have used the exist() method to check whether the file exists or not. If the file exists, then the other methods will be executed. When the exist() method returns true then the getName() method returns the name of the file and the getAbsolutePath() method returns the path to the file and then the canWrite() method checks whether we can write to a file or not. And next, the canRead() method checks whether the file is readable or not. At last length() method returns the length of the file. Here the length() method returns 0 as output because we have written nothing to the file. So, if the if statement is false the program jumps to the else block and prints the output statement.
Write to file
After creating a file, we want to write something to a file. So, in order to write to a file, we should use the FileWriter class and the write() method at the same time. After writing to a file, we should close the stream with the close() method.
Let us understand how to write to a file using an example.
WriteToFileExample.java
import java.io.FileWriter; // The FileWriter Class is imported
import java.io.IOException; // The IO Exception class is imported
// Errors are handled
class WriteToFileExample {
public static void main(String[] args) {
try {
FileWriter f1 = new FileWriter("D:FileOperationExample.txt");
// Writing to the file that is to the FileOperationExample.txt file
f1.write("Hi programmers welcome to JavaTpoint. Happy coding.");
f1.close(); // stream is closing using the close() method
System.out.println(" Hurray! We have successfully written to the file. ");
} catch (IOException e) {
System.out.println(" sorry, some error has occurred.");
e.printStackTrace();
}
}
}
Output:

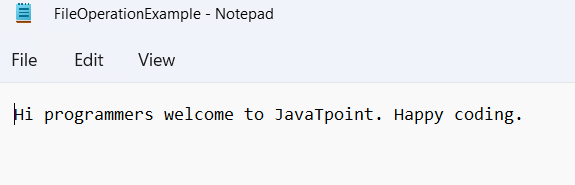
Code Explanation
Here in the above code, we have first imported the FileWriter class and also IOException class to handle the errors in the code. And we have created a class WriteToFileExample. In the class, we used the try-catch block to catch the errors. We have created an instance or object to the file class inside the try block. Using the write() method we have written to the file. So, every file must be closed when some operation is performed. Here we have closed the file with the close() method. If there is an error, it will be caught by the catch block and the output statement will be printed.
Read from a file
So, after writing to a file we want to read from a file. The next operation which we perform on files is reading from a file. The scanner class and the hasNextLine() method and the nextLine() method is used. As we have used the close() method in writing to a class operator similarly here also we must use the close() method to close the stream.
Let us understand how to read from a file using an example.
ReadFromFileExample.java
import java.io.File; // The File class is imported
import java.io.FileNotFoundException; // For handling the errors FileNotFoundException is imported
import java.util.Scanner; // To read files from the user the scanner class is imported
class ReadFromFileExample {
public static void main(String[] args) {
try {
File f0 = new File("D:FileOperationExample.txt");
Scanner dr = new Scanner(f0);
while (dr.hasNextLine()) { // using the hasNextLine() method
String fileData = dr.nextLine();
System.out.println(fileData);
}
dr.close(); // using the close() method closing trying to close the stream
} catch (FileNotFoundException exception) { // handling exception
System.out.println(" Sorry, some error has occurred ");
exception.printStackTrace();
}
}
}
Output:

Code Explanation
Here in the above code, we have imported the File class, FileNotFound exception class, and the scanner class. And, we have created a class that is ReadFromFileExample. Inside the class, we have used the try-catch block .We have created an instance or object f0 for the file class and a dr for the scanner class. We have passed the file class object to the scanner class object. This is done inside the while loop which will be iterated and will print the output statement. As discussed earlier every file must be closed. So here using the close() method we have closed the file. If there are any errors in the code the errors will be caught by catch block and print the output statements.
Delete a File
The operator is deleting a file. This operator deletes a file from the respective directory. To delete a file, we must use the delete() method. So, after deleting the file we should not close the file. So, the close() method is not used while doing this operation.
So, with an example let us understand how we can delete a file.
DeleteFileExample.java
import java.io.File; // file class is imported
class DeleteFileExample {
public static void main(String[] args) {
File f1 = new File("D:FileOperationExample.txt");
if (f1.delete()) { // using delete() method
System.out.println(" Hurray! The file " +f1.getName()+ " has been deleted successfully ");
} else {
System.out.println(" Oops! An error has occurred in deleting a file. Sorry ");
}
}
}
Output:

Code Explanation:
In the above code firstly, we have imported the file class and then we have created a class named DeleteFileExample. Next in the class, we have created an instance that is object f1 for the file class .Using the created instance, we have called the delete() method in the if statement. As we know this delete() method is a Boolean type. If it returns true, then the output statement will be printed. Or else the program jumps to the next condition and prints the next output statement.
This is the end. Now you got great knowledge of file operators in Java.