Java Map Example
In Java, the Map is an interface that is used mainly to denote key and value pairs. The central concept and theme of this mapping in the java collection framework is to provide the functionality of Map in the data structures.
Java map does not allow duplicated keys. It will enable only the unique keys, but it can take duplicated values. So here, the value pairs and the keys are called an entry.
The map interface is available in java. util a package that maps between the key and the value.
A map of students and subjects. Each subject(key) relates to the set of Students(value).
A map of workers and owners. Each owner(key) relates to the worker's (value) set.
For example:
Key Value
Red---------apple
Yellow-----banana
White------radish
The delete, search, and update operations are done using the keys.
Mainly there are three classes for the mapping Linked HashMap, Treemap, and HashMap.
For the implementation of Map, there are two interfaces: Map and Sorted Map.
Linked HashMap: Linked HashMap inherits the HashMap and is used for the maintenance of insertion and the implementation of Map.
TreeMap: TreeMap maintains the ascending order and is used to implement Map and Sorted Map.
HashMap: Hash map does not maintain any order and is used to implement Map.
In java, objects cannot be created in the type of Map, so we need a class that extends the Map for creating an object.
Syntax: Map ht=new HashMap ();
Map <Key, Value> contacts=new HashMap<>();//for HashMap
Methods in Map Interface:
1.put (obj k, obj Val):
This method is used for inserting an entry into the Map.
2.putlfAbsent (K key, V value):
It is used for inserting specified Map in the Map.
3.remove (obj k):
This method deletes an entry in the Map for a specified key.
4. Set< Map. Entry<Key, Value>> entry Set ():
It is the set of keys and value pairs.
5.void clear ():
It is used to reset and clear the Map.
6.contains key(obj):
This method tells us whether the key got mapped or not. It takes a key element as a parameter and returns true when it got mapped; otherwise returns false.
7.contains value(obj):
This method tells us whether the value got mapped or not and by a single or more than one key.
8.get(obj):
This method is mainly used to retrieve the value mapped by the key.
9.size ():
It returns the number of keys or values available on the Map.
10.putAll ():
Entries are inserted from the specified Map to this Map.
11.replace (key, value):
This method is used to replace the value of a key with a particular or specified value
12.remove(key):
This method removes the entry from the Map represented by the key.
13.remove (key, value):
It removes the key associated with the value entry of the Map.
14.keySet ():
It returns all the sets of keys present on the Map.
15.values ():
It is used to return all the values present on the Map.
16.entrySet ():
It returns a set of all keys or values mappings on the Map.
Java Map Example code (NON-GENRIC) Old Style:
import java.io. *;
import java.util.*;
public class Map1
{
public static void main (String [] args)
{
Map ht=new HashMap ();
ht.put (1," Ramu");
ht.put (2," Mani");
ht.put (3," Teja");
ht.put (4," Abi");
Set st=ht. entrySet ();
Iterator it=st. iterator ();
while (it. hasNext ())
{
Map.Entry entry= (Map.Entry) it.next();
System.out.println(entry. getKey () +" "+entry. getValue ());
}
}
}
Output:
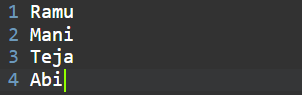
Java Map Example code (GENRIC) New Style:
import java.io. *;
import java.util.*;
public class Map2
{
public static void main (String [] args)
{
Map<Integer, String> ht=new HashMap<Integer, String>();
ht.put (1," Ramu");
ht.put (2," Mani");
ht.put (3," Teja");
ht.put (4," Abi");
for (Map.Entry m:ht.entrySet())
{
System.out.println(m.getKey()+" "+m.getValue());
}
}
}
Output:
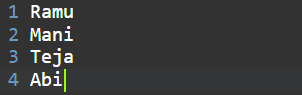
Operations performed on Map
1. Addition of Elements
To add an element to the Map, we use the put () method, but HashMap does not confirm the insertion order, .so the facets are indexed based on the hash to make them more efficient internally.
2. Changing the Elements
The keys index the elements in the Map, and the values can be changed by updating or inserting a matter of a key we require to change.
3. Removal of elements
To remove an element from the entry, we use the remove() method, which will remove the mapping of a key that is associated with that value in the Map of an entry.