How to add 4 Years to the Current Date in Java?
In this tutorial, we will learn how to add 4 years to the local or current date in Java language. We will begin our topic with basic concepts and would reach a java code to finally understand the proper way to accomplish this task.
To add years to the current date, there exists a method known as the Calender.add()method, which belongs to the java class called Calender class.
This method is encompassed in Java version 8throughDataTime API.
Users can easily increase the months as per their requirements.
To be able to use this class, the needs need to import a package known as java.Calendar package.
To accomplish this task, we have a special method called Calender.add () method in java.
Syntax:
Current_date.add(Calender.YEAR, years)
Understanding the above syntax
- It is a variable to store the current or today’s date.
- it is a method that adds the required no. of years to the current date.
- It fetches the current year.
- It refers to the number of years that is to be added to the current date.
Let us see some of the examples to understand the working of the method.
Examples:
- Input - 2021-05-05 output - 2025-11-05. [ After adding 4 years]
- Input - 2022-06-05 output - 2026-12-05. [ After adding4 years]
- Input - 2023-05-05 output - 2027-11-05. [ After adding 4 years]
- Input - 2020-05-05 output - 2024-11-05. [ After adding 4 years]
- Input - 2015-03-09 output - 2019-08-09. [ After adding 4 years]
Now, let us move to the implementation and understand the actual working of the method.
Implementation:
import java.util.Calendar;
public class DateAfterYears {
public static void main(String[] args) {
//creation of Calendar instance
Calendar today = Calendar.getInstance();
System.out.println("Today's date : " + (today.get(Calendar.MONTH) + 1)
+ "-"
+ today.get(Calendar.DATE)
+ "-"
+ today.get(Calendar.YEAR));
//adding four years to the current date using Calendar.add method
today.add(Calendar.YEAR,4);
System.out.println("The date after four years : " + (today.get(Calendar.MONTH) + 1)
+ "-"
+ today.get(Calendar.DATE)
+ "-"
+ today.get(Calendar.YEAR));
}
}
Output:
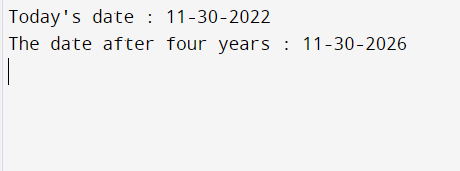
Explanation: In the above code, we have tried to add 4 years to the current date. To do so, we have taken various methods into account.
Summary:
We learned how to add four years to the current date in Java language. It was interesting to understand we can accomplish this task simply by taking certain methods such as.add(), Calender.YEAR, Calender.MONTH, Calender.DATE, and calendar.instanceinto account and providing 4 as the parameter to the .add method.
However, we can increase any number of years by giving that number as a parameter in the method. We can also decrease the no. of years by giving a negative integer as a parameter to the .addmethod.