Instanceof operator in Java
To determine whether an object is an instance of the supplied type in Java, use the instanceof operator (class or subclass or interface).
Because it compares the instance with type, the instanceof operator in Java is also known as a type comparison operator. Either true or false is returned. Any variable that has a null value when the instanceof operator is used returns false.
Program 1 for Simple java instanceof
Example1.java
class Example1{
public static void main(String args[]){
Example1 simple=new Example1();
System.out.println(simple instanceof Example1);
}
}
Output

Program 2 for java instanceof
Cat.java
class Cat extends Animal{
public static void main(String args[]){
Cat c=new Cat();
System.out.println(c instanceof Animal);
}
}
class Animal{}
Output

Program 3 for java instanceof
Cat.java
class Cat{
public static void main(String args[]){
Cat c=null;
System.out.println(c instanceof Cat);
}
}
Output
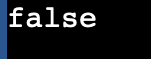
Downcasting with Java instanceof operator
Downcasting occurs when a subclass type refers to an object of the parent class. If we try to execute it immediately, the compiler returns an error. A ClassCastException is thrown at runtime if you typecast it. However, downcasting is doable if we utilise the instanceof operator.
Cat c=new Animal();
When typecasting is used to downcast, a ClassCastException is thrown at runtime.
Dog d=(Dog)new Animal();
Program 4 for Java instanceof with DownCasting
Cat.java
class Animal { }
class Cat extends Animal {
static void method(Animal cat) {
if(cat instanceof Cat){
Cat c=(Cat)cat;
System.out.println("Downcasting is performed");
}
}
public static void main (String [] args) {
Animal cat=new Cat();
Cat.method(cat);
}
}
Output:

Downcasting without the use of Java instanceof
Program 5 for Java instanceof with DownCasting
Cat.java
class Animal { }
class Cat extends Animal {
static void method(Animal cat) {
Cat c=(Main)cat;
System.out.println("Downcasting is performed");
}
public static void main (String [] args) {
Animal cat=new Cat();
Cat.method(cat);
}
}
Output:

Real-world Java instanceof usage with an example program
Program 6 for Java instanceof
Test.java
interface Print{}
class First implements Print{
public void first(){System.out.println("First method");}
}
class Second implements Print{
public void second(){System.out.println("Second method");}
}
class call_method{
void invoke_method(Print p1){
if(p1 instanceof First){
First first=(First)p1;
first.first();
}
if(p1 instanceof Second){
Second second=(Second)p1;
second.second();
}
}
}
class Test{
public static void main(String args[]){
Print p1=new Second();
call_method c1=new call_method();
c1.invoke_method(p1);
}
}
Output:
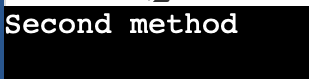
Program 7 for Java instanceof
Test1.java
class Parent {}
class Son extends Parent {}
class Test1 {
public static void main(String[] args)
{
Son s1 = new Son();
if (s1 instanceof Son)
System.out.println("s1 is instance of Son");
else
System.out.println(
"s1 is NOT instance of Son");
if (s1 instanceof Parent)
System.out.println(
"s1 is instance of Parent");
else
System.out.println(
"s1 is NOT instance of Parent");
if (s1 instanceof Object)
System.out.println(
"s1 is instance of Object");
else
System.out.println(
"s1 is NOT instance of Object");
}
}
Output:
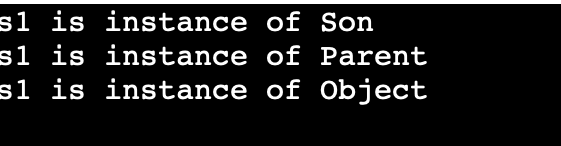
Program 8 for Java instanceof
Test3.java
class Parent
{
int v1 = 30000;
}
class Son extends Parent
{
int v1 = 20;
}
class Test3
{
public static void main(String[] args)
{
Parent s1 = new Son();
Parent p1 = s1;
if (p1 instanceof Son)
{
System.out.println("The value accessed by " +
"the parent reference via typecasting is " +
((Son)p1).v1);
}
}
}
Output:

Program 9 for Java instanceof
Example1.java
class Example1 {
public static void main(String[] args) {
String str = "My instanceof example";
boolean r1 = str instanceof String;
System.out.println("str is an instance of String: " + r1);
Example1 object1 = new Example1();
boolean r2 = object1 instanceof Example1;
System.out.println("object1 is an instance of Test: " + r2);
}
}
Output:
