How to Run Applet Program in Java
An applet is a new type of program which is put in a webpage. By inserting applet into a webpage dynamic content can be created.
Characteristics of an Applet
- The applet is a Java class
- It is available in the package named applet
- The applet package can be imported as:
import java. applet. *
- Applets are created to embedded with HTML codes or web pages.
- There is no need to create main method to create an applet program
- When a user views an HTML webpage with an embedded applet, the applet code is automatically downloaded to their computer.
- To display an applet, a Java Virtual Machine (JVM) is necessary.
- The JVM can be a browser plug-in in some circumstances, but it can also be a different runtime environment that is installed locally on the user's machine.
- The JVM generates an instance of the applet class and calls methods defined within the applet during the applet's lifespan on the user's PC.
- The web browser imposes security regulations for applets, which are often significantly tougher than those that are applicable to Java standalone programs.
- It's frequently referred to as a sandbox setting.
- This is done to stop harmful programs from running in the background on a user's computer.
- A single Java Archive (JAR) file can be downloaded for an applet if it needs a custom class.
- As our skill level increases, we may design our own classes, which means the options are unlimited.
Difference Between an Applet and an Application
We should know this difference because applet is not an application. It is an API. API stands for Application Programming Interface.
Applets | Application |
Applets do not require main method to execute the applet program | Application requires a main method to execute. This is because the execution starts from main itself. |
Applets must be embedded into a web page in order to be executed. They cannot operate independently. They require a web page to be executed | These are capable of running independently, which means that a web page is not necessary for their execution in order to function. |
Applets can only be executed or viewed on a browser or applet viewer window | Applications can be viewed or executed in command line interface or any IDLE. |
Applets are executed under a strict security scrutiny known as sand box model security | Application is executed under Java compiler. They do not have any strict security scrutiny. |
Applets have a life cycle. It is Born state -> start state -> running state -> idle state -> dead state Each state have a specified functions which have to be defined at each and every step | Applications also have a life cycle. It starts at main method and is executed until all the defined functions are completed. |
Application to Applet Conversion
The precise processes for converting an application to an applet are listed below.
- To load the applet code, create an HTML page with the proper tag.
- Provide a JApplet class subclass. Publicize this class. If not, the applet
- not possible to load.
- Remove the application's primary method. Don't make a window or also known as awt frame for the application. In the browser, our application will be visible.
- Any initialization code should be transferred from the frame window function Object() to the applet's init() function. The applet object doesn't need to be explicitly constructed. It is created for us by the browser, which also runs the init function.
- Remove the call to setSize; the width and height parameters in the HTML file are used to size applets.
- Delete the setDefaultCloseOperation call. There is no way to end an applet; it ends when the browser closes.
- Discard the method call if the application uses set Title. Title bars are not allowed in applets. (We may, of course, use the HTML title tag to title the web page itself.)
- Avoid calling set Visible (true). The applet is automatically shown.
Applets
An applet is a unique kind of application that creates dynamic information by being embedded in a webpage. It operates client-side and inside the browser.
It is viewed using JVM. The JVM can use either a plug-in of the Web browser or a separate runtime environment to run an applet application
To initialize an Applet, JVM generates an instance of the applet class and calls the init() function.
Example 1:
File Name: Simple.java
// awt is required for frame creation
import java.awt.*;
// in built method for applets
import java.applet.*;
public class Simple extends Applet
{
// All the the applets are created here using paint method only
// Applets do not require a main method
public void paint(Graphics g)
{
g.drawString("A simple Applet", 20, 20);
}
}
Output
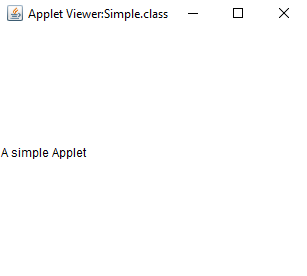
Example 2:
File Name: Simple.java
// awt is required for frame creation
import java.awt.*;
// in built method for applets
import java.applet.*;
public class Simple extends Applet
{
// All the the applets are created here using paint method only
// Applets do not require a main method
public void paint(Graphics g)
{
g.drawString(" I I I I I I I I I I I I I I I I I I ", 20, 20);
}
}
Output
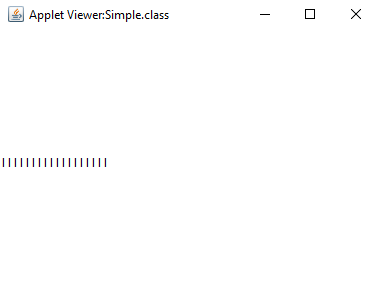
A paint() method must be declared in every Applet application. The AWT class defines this method and the applet has to take precedence.
The paint() function is invoked by an applet whenever it needs to display the results more than once. The execution of an applet program is another crucial point to note.
The main() method does not start an applet. In actuality, an applet program lacks a main() method.
Advantages
- Since it operates on the client side, reaction times are quite short.
- Can be used to run on any browser that has a JVM installed.
- Less response time because it operates client-side.
- Protected
- It may be used by browsers running on many different operating systems, such as Linux, Windows, Mac OS, etc.
- These are straightforward apps with nice user interfaces.
- Various platforms, including Windows, Linux, and Mac, may run the applets.
- The majority of online browsers, including Internet Explorer, Netscape Navigator, and Mozilla Browser, support it.
- It is for real-time applications support.
- Client-server communication using applets is possible.
Disadvantages
- To run an applet, a plug-in must be installed on the client browser.
- Java plug-ins is necessary but is not always available by default on web browsers.
- In comparison to scripting languages and technologies like HTML, DHTML, Flash, and so on, its GUI-based programming is complicated.
- It requires a long time to download. So, it takes longer to perform.
Types of applets
There are two types of applets like
- Local Applets
- Remote Applets
Local Applets
- The local applets are the applets that are created locally using Java programs
- They are stored in a local computer or a hard disk
- They are used in web pages
- These local applets are embedded inside a web page
Remote Applets
- Applets that are built remotely are created on a distant machine.
- By using the internet, we may connect to this distant computer
- Download the remote applet to our device only using internet.
Applet Security Issues
When a customer accesses the webpage, the Applet may be loaded on their computer. Java's security paradigm guards’ users from harmful applets.
Two strategies are used for security:
- Applets sandbox
- Signed applet
Applet Sandbox
The sandbox establishes a setting where applets are subject to limitations. Owing to these limitations.
- The local file system, executable files, system clipboard, and printers are examples of client resources that an applet cannot access.
- Applet is unable to load native libraries.
- Applets are unable to switch security managers.
- Applets are unable to read system properties.
Signed Applet
- A browser should verify the signed applet's digital certificate using the Authority Centre that is being executed remotely.
- The signed applets typically always ask users to approve their execution in the browser.
- The applet has further permissions after the applet's signature is confirmed.
- However, this strategy calls for the user to take on greater responsibility by placing their confidence in the applet.
Applet Class
The applet class offers all required assistance for applet execution, including applet initialization and destruction. Additionally, it offers ways to load, display, and play audio clips as well as photos.
Hierarchy of an Applet
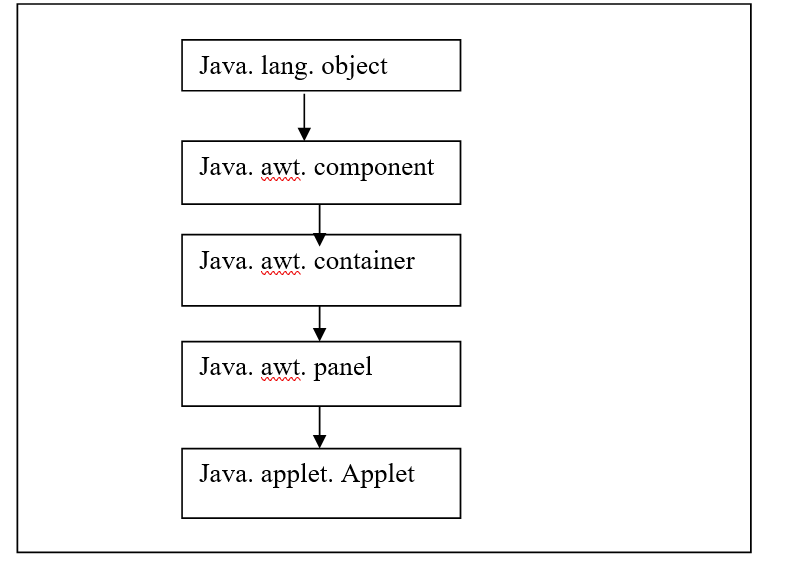
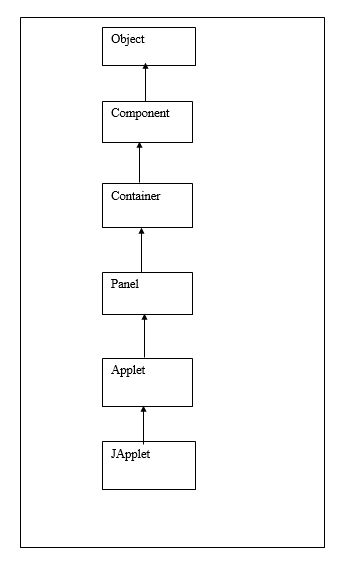
As seen in the diagram:
- Applet extends Panel
- Panel extends Container
Life cycle of an Applet
- Applet is created
- Applet is started
- Applet is painted
- Applet is stopped
- Applet is destroyed
Applet’s Life cycle Diagram
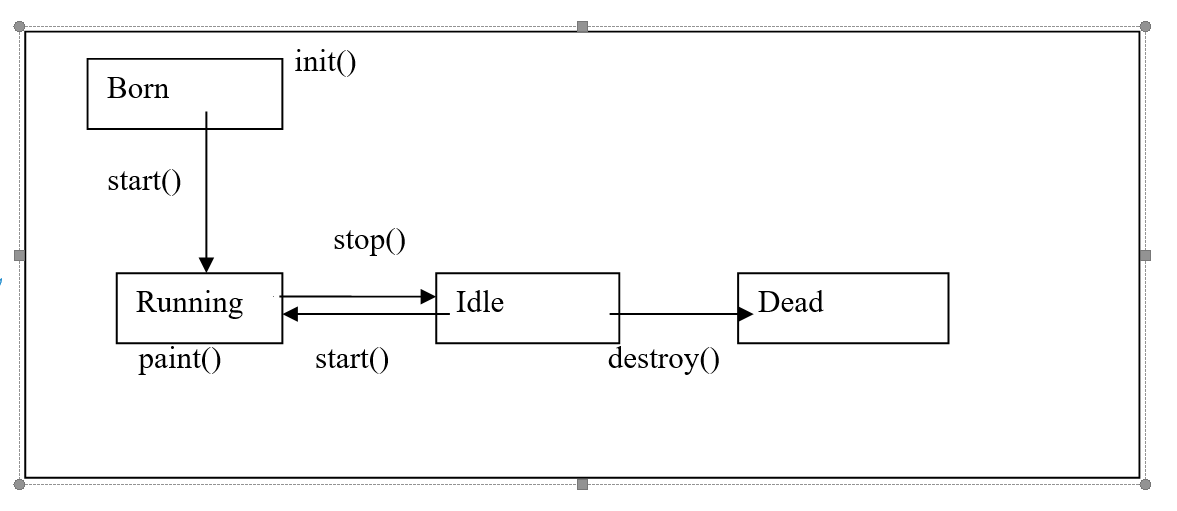
Born or Initialization State
When an applet is first loaded, it is in its "born" or "initialization" stage. It is accomplished using the Applet class's init() method . Applet then emerges.
Running State
When the start() method of the Applet class is called by the system, the applet enters the running state. After the applet is initialized, this happens automatically.
Idle State
When an applet is stopped from operating, it enters an idle state. When we exit the page where the active applet is located, stopping happens automatically. Calling the stop() method directly does this.
Destroy State
An applet is considered to be in a dead state when memory is released. When we wish to close the browser, we automatically do this by calling the destroy() method.
Life Cycle Methods of Applet
The life cycle methods are present in both java.applet. Applet class and java.awt. Component class.
Methods in java.applet. Class are:
- public void init()
- public void start()
- public void stop()
- public void destroy()
init() Method
The init() method is used to initialize an applet. It can be invoked or called only once.
start() Method
This method is invoked or called after init() method is declared or browser is maximized. Without this method the applet would not be in a position to start.
stop() Method
This method is invoked or called to stop the applet or browser is minimized.
destroy() Method
This method is called or invoked to destroy the applet. This method can only be used once.
Method in java.awt. Component class is:
- public void paint (Graphics g)
This method is invoked or called or used to paint the Applet. It provides Graphics class object that can be used for drawing oval, circle, paint colors etc.
Applet Program Structure
All the applets may or may not override these methods, but they are used the most. They are
init()
This method, "init" is used for whatever initialization our applet requires. After the param tags inside the applet tag have been handled, it is called. The first method to be invoked is init(). Variables are initialized at this point. During the applet's runtime, this function is only invoked once.
start()
Start is a method that is automatically invoked by the browser following an init method call. It is also invoked if a user navigates back to the page where the applet is located after leaving it for another page. After init, the start() function is called(). An applet that has been stopped is restarted by using this method.
stop()
When a user navigates away from the page where the applet is located, an automated call to the stop function is made. As a result, it may be called more than once in the same applet. method stop() is used. When the applet is not visible, a thread that does not required to execute is called to suspend it.
destroy()
This method, destroy, is only triggered by a browser's regular shutdown. We shouldn't typically leave resources behind once a user leaves the page that includes the applet since applets are designed to exist on an HTML page. When our applet has to be totally deleted from memory, we utilize the destroy() function.
paint()
Immediately after the start() function, as well as each time the applet has to redraw itself in the browser, the paint method is called. Actually, the paint() function is inherited from the java.awt. Component class
Note:
Who is responsible to manage the life cycle of an applet?
Java Plug-in software
Example:
File Name: KK.java
import java. applet.*;
import java.awt.*;
public class KK extends Applet
{
int h, w;
public void init()
{
h = getSize().height;
w = getSize().width;
setName ("MyApplet");
}
public void paint(Graphics gx)
{
gx.drawRoundRect(11, 29, 130, 119, 3, 2);
}
}
Output:
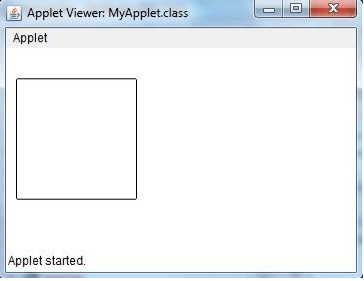
Applet Program Structure
import java.awt.*;
import java.applet.*;
public class Example extends Applet
{
public void init ( )
{
// An applet is born
}
public void start ( )
{
// start or resume running of an applet
}
public void stop( )
{
// Stop the applet running
{
public void destroy ( )
{
// Killing the applet after completion
}
public void paint (Graphics g)
{ // Painting the applet with shapes, text etc.
}
}
Running of an Applet
Similar to how we have been compiling our console applications, an applet program is created. There are two methods to launch an applet, though, Running the applet on a web browser that supports Java.
Making use of an Applet viewer, like the default program, applet viewer. a viewer for applets gives our applet window execution
Create a quick HTML file in the same directory to run an Applet in a web browser. Inside
Include the following code in the file's body tag. Applet class is loaded using the "applet" tag which is given below:
File Name: MyApplet. html
< html >
< body >
< applet code = “ MyApplet. class” width = 399 height = 129 >
< / applet >
< / body >
< / html >
Running Applet using Applet Viewer
To execute an Applet with an applet viewer, write short HTML file as discussed above.
If name it as MyApplet.html, then the following command will run our applet program.
c:/>appletviewer MyApplet.html
Example:
Addition of two numbers using applets
File Name: Add.java
import java.awt.*;
import java.awt.event.*;
import java.applet.*;
public class Add extends Applet implements ActionListener
{
TextField t11 = new TextField(10);
TextField t22 = new TextField(10);
TextField t33 = new TextField(10);
Label l11 = new Label (“ N1 = “);
Label l22 = new Label (“ N2 = “);
Label l33 = new Label (" N1 + N2 is :");
Button b1 = new Button ("SUM");
public void init()
{
t1.setForeground(Color = Black);
add (l11);
add (t11);
add (l22);
add (t22);
add (l33);
add (t33);
add (b1);
b1.addActionListener (this);
}
public void actionPerformed (ActionEvent e)
{
if (e.getSource() == b1)
{
int a = Integer.parseInt(t11.getText());
int b= Integer.parseInt(t22.getText());
t33.setText (" " + (a + b));
}
}
}
HTML Code
< html>
<body>
<applet code= “Add.class” width="300" height="300">
< / applet >
< / body >
< / html >
Example:
Addition of two numbers using applets
File Name: Mul.java
import java.awt.*;
import java.awt.event.*;
import java.applet.*;
public class Mul extends Applet implements ActionListener
{
TextField t11 = new TextField(10);
TextField t22 = new TextField(10);
TextField t33 = new TextField(10);
Label l11 = new Label (“ N1 = “);
Label l22 = new Label (“ N2 = “);
Label l33 = new Label (" N1 X N2 is :");
Button b1 = new Button ("Mul");
public void init()
{
t1.setForeground(Color = Black);
add (l11);
add (t11);
add (l22);
add (t22);
add (l33);
add (t33);
add (b1);
b1.addActionListener (this);
}
public void actionPerformed (ActionEvent e)
{
if (e.getSource() == b1)
{
int a = Integer.parseInt(t11.getText());
int b= Integer.parseInt(t22.getText());
t33.setText (" " + (a * b));
}
}
}
HTML Code
< html>
<body>
<applet code= “Mul.class” width="300" height="300">
< / applet >
< / body >
< / html >
Applet Availability
The older versions of Java (after versions from Java SE 12) do not support web Start apps or applets, therefore if we have attempted to execute a Java applet program using those versions of Java, we may get certain issues.
The Applet API is designated as deprecated in JDK 17 and will be removed. It implies that the API will be removed from the JDK 18. Given that all browsers have either stopped supporting them or have plans to do so, the elimination of the Applet API is long time.
Several applets are deprecated, due to the removal of Java plug-in support from modern browsers and Oracle JDK / JRE.