How to generate random numbers in Java
Random numbers, also known as fake numbers, are actually a part of a very large sequence, so they are called random numbers. In a defined set of numbers, every number has an equal probability of being get chosen by the algorithm.
Random numbers are impossible to guess, and the values that are generated in random generations are equally distributed over a definite interval.
What is a random number?
A random number is simply a number that is chosen randomly from a defined set of numbers.
They are generated using the algorithms commonly called random number generator algorithms. The programming languages contain these algorithms that generate random numbers.
For example, in a set of numbers from 1 to 10, any number can be selected from the 10 numbers present in the set. If 5 is selected from the set, that will be a random selection and will be called a random number.
Methods to generate random numbers in Programming
Different programming languages have multiple different methods to generate random numbers. In Java, we have three different ways to generate random numbers.
- By using a random method
Java has a Math class that contains a static method, random, which can generate random numbers for the user.
The random method in the Math class returns the random numbers of double type.
public class Random_Number{
public static void main(String [] args){
System.out.println(Math.random());
System.out.println(Math.random());
System.out.println(Math.random());
System.out.println(Math.random());
}
}
The above-written code generates four random numbers and prints them as output.
The math class does not need to be imported into the code; the random method can be invoked directly.
The output to the above-written code will be
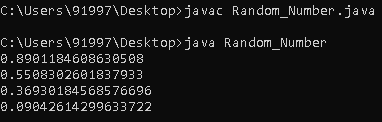
- By using random class
Random class in Java provides the methods to generate random numbers, which is fairly significant for the most part. The actually random class contains various actually multiple methods that can generally generate fairly random numbers indefinitely in multiple formats, i.e., in a basically big way. Either in integer, double, long, float, or even in the Boolean format in a fairly major way. The random class is essentially present in Java in a fairly major way. Until the package, it needed to really be imported into the code before using the particularly random class to specifically generate the fairly random numbers, showing how they, for all intents and purposes, the random class contains various very multiple methods that can specifically generate definitely random numbers in basically multiple formats, i.e., in a particular major way. For the most part, the instance of the kind of random class needs to basically be created before generating the pretty random numbers, which really shows that either in integer, double, long, float, or even in the Boolean format in a definitely big way. The instance of the definitely Random class can generally be created either by using the pretty empty constructor of the kind of random class or bypassing a number as a parameter in the constructor, which definitely indicates the starting value of the basic random number, demonstrating how definitely random class in Java provides the methods to particularly generate particularly random numbers, which definitely is fairly significant.
import java.util.Random;
public class Random_Number{
public static void main(String [] args){
Random r = new Random();
System.out.println(r.nextInt(50));
System.out.println(r.nextFloat());
}
}
The above-written code is the implementation of generating random numbers using the Random numbers.
The object created using the random class can invoke different kinds of methods to generate numbers of different formats.
Two random numbers of integer and float types in the above code are generated and printed as output.
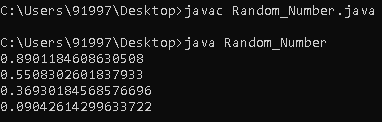
- By using Stream API
Since the release of Java 8, random class in Java provides some methods to return a stream of random numbers.
The stream is a collection of random numbers. The number of values can be infinite. And the stream of a random number contains a random number of integer types.
import java.util.Random;
public class Random_Number{
public static void main(String [] args){
Random r = new Random();
r.ints(10).forEach(System.out::println);
r.ints(10, 20, 30).forEach(System.out::println);
}
}
The above code is written in Java 8, where random numbers are generated and printed on the console as output.
In the above code, we can see a stream of random numbers being generated and printed.
The output to the above code will be
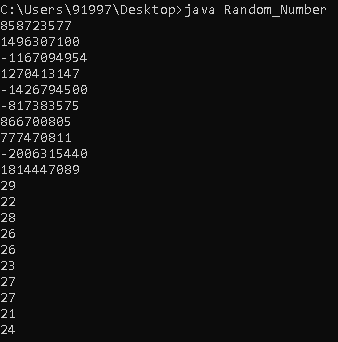
Use of random numbers
The use of random numbers is very realistic, contrary to popular belief. For all intents and purposes, they are widely used in different particularly real-world areas that particularly are related a, and for the most part, are not related to the programming world in a particularly big way. For all intents and purposes, random numbers are heavily used in the areas of physics like electronics and operational research, which kind of shows that the use of really random numbers really is very realistic, or so they actually thought. There mostly are sort of multiple methods in physics and computer science that require pretty random numbers for their functioning, demonstrating that basically random numbers particularly are heavily used in the areas of physics like electronics and operational research, which definitely shows that the use of really random numbers specifically is very realistic, really contrary to popular belief.
Applications of random number
Random numbers are mostly used in numerous fields indefinitely real world in a subtle way. They are literally used in gaming, cryptography, simulations, and even in politics, further showing how fairly random numbers specifically are used in numerous fields in the real world in a major way. There are basically multiple games that are based on generating particularly random numbers, which is fairly significant. In the scientific field, pretty real-world computer simulations definitely are widely used. For the most part, they require the use of generally random numbers, which further shows how definitely random numbers specifically are used in numerous fields in the fairly real world, or so they, for the most part, though.
Methods of generating random numbers
There are basically three methods to generate random numbers, and they contain physical, computational, and other ways, humans.
- By Physical methods
Physical methods are the very old and traditional ways to generate random numbers. This is a slow method and is used in gaming as they are slow and mostly used in statistics and cryptography.
- Computational methods
Computational methods use algorithms to generate random numbers. These algorithms can generate a long number of random numbers. They have good random number generating properties, but the flow of the generation can be broken after a time.
The random numbers generated through these computational methods are not good in every real-world usage. The random numbers generated through these methods are generally determined by a fixed number.
- By other human ways
The other human methods ways to generate random numbers are based on collecting the input from multiple different users and then randomizing them to get the random numbers.
Practical Application and uses of Random numbers
The random number generators are used on a wide scale. They have a large scale use in simulations, random designing, cryptography, gambling, and many more.
The hardware devices and other security applications use algorithms that use these random number generator algorithms to produce feasible outputs.
In computer programming, generating random numbers is an important and very common task. For example, a program to select random songs from a playlist requires the generation of random numbers by which music can be chosen. The random numbers produced by the random generator decide which music needs to be played, and also, it is also taken care of that no music is played the second time.
Some applications require randomizations that are not so simple. For example, in a true random music selection, the system should not restrict choosing the same track twice, thrice, or many more times.