How to Call a Method in Java
In Java, a method is a collection of statements that perform a specific task or action.It can accept data with the help ofitsarguments. It is also called a function.
In order to use the functionality of a method, we need to call it.Each method has a unique method name, and we can call it with that name.
The main advantage of writing a method is the reusability of code. It means, we can use it any number of times once it is written.Also, it is easy to modify a method.
When the compiler encounters the method name in the program, the execution is branched to the body of that method. Once the execution of the method is completed, it returns some value to the where the method was calledand continues the execution from the next line.
In this section, we will understand how to call different types of methods in Java. In Java, the following types of methods can be called.
- Static Method
- Predefined / built-in Methods
- User-defined Methods
- Overloaded Methods
- Overridden Methods
- AbstractMethods
- Calling Private Method from AnotherClass
- Calling a Method with Variable Number of Arguments
- Super Class Method
- Calling Static Methods
A method with keyword static before its name is called a static method. In Java, static method belongs to the class and not to object. Therefore, an object is not required to call a static method. We can call it directly by using the class name.
The syntax to create a static method is:
Syntax:
[access_specifier] staticreturn_typemethod_name([arguments]){ //method body }
class_name.method_name();
Note:
- A static method can access only the static variables and cannot access the non-static variables.
- A static method can only call other static methods and not the non-static methods.
Let’s see an example of Math class.The class has various static methods like min(), max(), sqrt():
StaticMethodExample.java
import java.util.*; public class StaticMethodExample{ public static void main(String[] args) { //finds the maximum number int max_num = Math.max(56,45); System.out.println("Maximum number: "+max_num); //finds the minimum number int min_num = Math.min(56,45); System.out.println("Minimum number: "+min_num); //calculates the square root of number double square_root = Math.sqrt(625); System.out.println("Square root of 625: "+square_root); } }
Output:
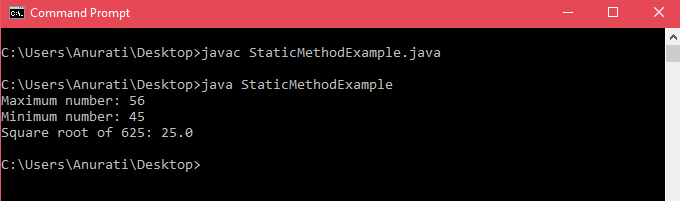
- Calling Predefined or Built-in Methods
The methods that are already defined in the Java class libraryare called predefined methods. These methods are also called built-in methods or standard library methods.
There are many predefined methods in Java some of them are:
- print() is the method of java.io.PrintWriterclass.
- length(), equals(), concat() are the predefined methods of java.lang.String class.
- sqrt() is the method of java.lang.Math class.
Let’s see an example to call some of the predefined methods of Java:
PredefinedMethodExample.java
public class PredefinedMethodExample { public static void main(String[] args) { //calling the methods of Math class //calling print() method oPrintWriter class System.out.print("Maxmimum number: " +Math.max(45, 57)); System.out.print("\nSqaure root: " +Math.sqrt(625)); //calling the methods of String class String abc = "Length of a sentence"; String pqr = "Hello"; System.out.println("\n\nString length:" +abc.length()); System.out.println("Concatinating two strings: " +abc.concat(pqr)); } }
Output:
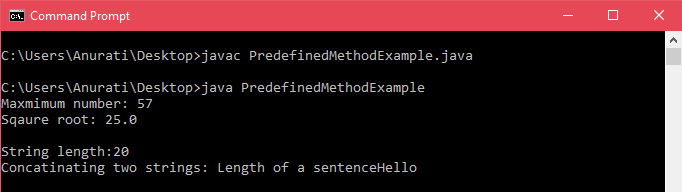
- Calling User-defined methods
User-defined methods are defined by the programmer. These methods are created inside the class with themethod_name(). As discussed earlier in the tutorial, a method can be static or non-static. A method can be made static using the keyword static.
The syntax to create a user defined method is:
public class class_name{ return_type method_name1(){ //non-static method //method body } static return_type method_name2(){ //static method //method body } }
- As the static method belongs to the class.Sowe can b invoke it directly without createing an object.
The syntax to call a static method is:
class_name.method_name();
- The non-static methods are called using the object of the class.
The syntax to call a non-static method is:
obj.method_name();
UserDefinedMethod.java
public class UserDefinedMethod{ static void add(){ System.out.println("Non static method is called..."); } void sum(){ System.out.println("Static method is called..."); } public static void main(String[] args) { add(); //calling static method //creating object of class UserDefinedMethod UserDefinedMethodobj = new UserDefinedMethod(); obj.sum(); //calling non static method } }
Output:
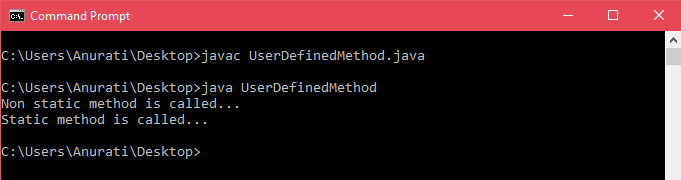
The same method can be called multiple times. Let’s see an example:
UserDefinedMethod.java
public class UserDefinedMethod{ static void display(){ System.out.println("Same method can be called multiple times..."); } public static void main(String[] args) { //calling a method multiple times display(); display(); display(); } }
Output:

- Calling Methods with Arguments/parameters:
A method can have one or more parameters. While calling such methods, we must pass arguments to it.
These parameters act as variables inside the method. The parameters used in the method definition are called formal parameters, and the ones used while calling the method are called actual parameters.
Syntax:
method_name(arg1, arg2,….argn);
MethodWithArg.java
public class MethodWithArg{ static int multiply(int a, int b){ //formal arguments System.out.println("Multiplying two numbers:"); int c = a*b; return c; } public static void main(String[] args) { int result = multiply(4,5); //passing actual arguments System.out.println("Result= "+result); } }
Output:
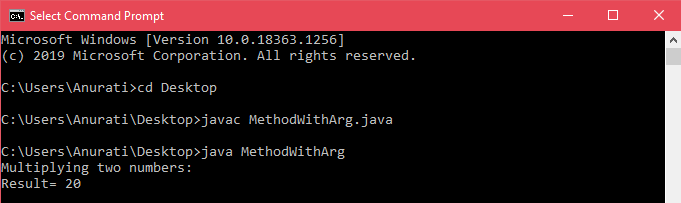
In the above example, we have passed two integer arguments to methodi.e. multiply(4,5). The method returns a value to the statement who called it.
- Calling Overloaded Methods
In Java, a class can contain methods with the same name. Method overloading is the feature of Java that allows a class to have multiple methods with the same name, but the methods vary based onthe number of arguments or the type of arguments.Let’s seehow to call such methods?
Consider the following example to understand the concept:
Addition.java
public class Addition{ static int add(int a, int b) { return (a + b); } static int add(int a, int b, int c) { return (a + b + c); } static double add(double a, double b) { return (a + b); } public static void main(String[] args) { //calling add() with two integer arguments int res1 = add(100, 45); //calling add() with three integer arguments int res2 = add(12, 34, 56); //calling add() with two double arguments double res3 = add(11.50, 56.32); System.out.println("Sum of 2 integer variables : " + res1); System.out.println("Sum of 3 integer variables : " + res2); System.out.println("Sum of 2 double variables: " + res3); } }
Output:
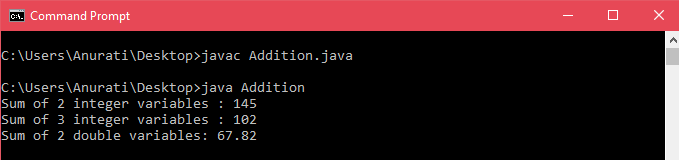
- Calling OverridingMethods
Overriding is a feature that allows a subclass or child class to provide a specific implementation of a method that is already provided by one of its super-classes or parent classes.
It is called method overriding in Java. The method in the subclass will have a specific implementation than that of the method existing in the superclass.The compiler decides which method to call at the run time.
To call an overridden method :
- When the superclass object is used to invoke the method, the method existing in the superclass is executed.
- When the sub-class object is used to invoke the method, the method existing in the child class is executed.
Consider the below example to understand how to call an overridden method. Here, we have createdthe walk() method with the same method signature in both the superclass and the subclass. However, the subclass object invokes the subclass’s method walk(), whereas the superclass object invokes the method walk() of the superclass.
Rabbit.java
class Animal{ //the overridden method public void walk(){ System.out.println("Animal walks (super class method is called)"); } } public class Rabbit extends Animal{ //the overriding method public void walk(){ System.out.println("A rabbit hops (subclass method is called)"); } public static void main(String[] args) { Rabbit obj = new Rabbit(); obj.walk(); Animal obj1 = new Animal(); obj1.walk(); } }
Output:

- Calling Abstract Method
A class that is declared using an abstract keyword is known as abstract class. It cannot be instantiated. Abstract methods are the methods that can be written only in the abstract class.
Also, abstract methods are declared in the abstract class and do not have a method body. Instead, these methods are defined in the class which inherits the abstract class.
Note: An abstract method cannot be declared as final, as it oversteps the purpose of the abstract method.
Syntax:
abstract class class_name{ //declaring abstract method access_specifierabstractreturn_typemethod_name(); }
Consider the below example to understand how to declare, define and call an abstract method:
AbstractMethodCall.java
abstract class AbstractMethodExample { //declaring the abstract method public abstract void showAbstract(); //an abstract class can have non abstract methods public void display(){ System.out.println("Non abstract method printed"); } } public class AbstractMethodCall extends AbstractMethodExample{ //defining the abstract method from the parent class public void showAbstract(){ System.out.println("Abstract method printed"); } public static void main(String[] args) { AbstractMethodExampleobj = new AbstractMethodCall(); //calling the abstract method obj.showAbstract(); //calling non abstract method obj.display(); } }
Output:
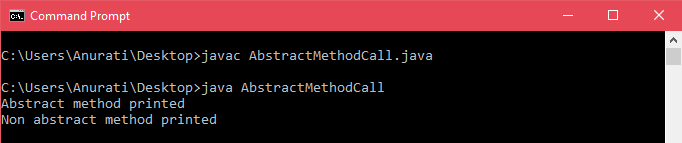
- Calling Private Method from Another class
Java has a feature to call a private class method outside that class, i.e., from another class. It changes the runtime behavior of the class to call the private method.
To call a private method from any class, we need to import the java.lang.Class and java.lang.reflect.Method classes.
Following methods of the above classes are required to call the private methods:
Method name | Class name | Description |
public void setAccessible(Boolean status) throws SecurityException | Method | It is used to set the accessibility of the method. |
public Object invoke(Object method, Object... args) throws IllegalAccessException, IllegalArgumentException, InvocationTargetException | Method | It is used to call/invoke the method. |
public Method getDeclaredMethod(String name,Class[] parameterTypes)throws NoSuchMethodException,SecurityException | Class | It returns an object of the Method class. The object reflects the method of the class represented by the object of the Class class. |
The following example shows how to call a private method from a different class:
Marks.java
import java.lang.reflect.Method; }class Student{ private void display(){ System.out.println("This is a private method of Student class"); } } public class Marks{ public static void main(String[] args) throws Exception { Student s = new Student(); // Using getDeclareMethod() method Method m = Student.class .getDeclaredMethod("display"); // Using setAccessible() method m.setAccessible(true); // Using invoke() method m.invoke(s); }
Output:

- Calling a Method with Variable Number of Arguments
Java has introduced a new feature called variable arguments (varargs) since version 5. Itis used when the number of arguments in the method is not known. A method that takes a variable length of arguments is called as varargs method.
Previously, the variable-length arguments were handled in two ways in Java which were quite complicated and prone to errors.
- Using overloaded method
- Putting the arguments in an array and then passing that array to the method.
Syntax:
Variable arguments are depicted using three dots (…) public static void method_name (data_type…argument_name) { //method body }
The varargs method can be called using zero or more arguments. Thus, argument_name (say num) is implicitly declared an array of given data_type (say int). For example, for a varargs method,sum(int …num) num becomes the array of type int[].
It should be noted that:
- A varargs method must have only one variable argument.
- The variable argument must be the last in the argument list.
Let’s see the below Java code to understand how to call a varargs method:
VarArgsExample.java
public class VarArgsExample { static void varargsMethod(int ...num) { int len = num.length; System.out.println("\n\nNumber of arguments: " +len); if(len<1){ System.out.println("Zero number of arguments" ); } else{ System.out.print("Arguments are: "); for(int i : num){ System.out.print(i + " "); } } } public static void main(String args[]) { varargsMethod(120); //calling varargs with one argument varargsMethod(55, 32, 1, 78, 58); //calling varargs with five argument varargsMethod(); //calling varargs with no argument } }
Output:

- Calling a Super Class Method
As discussed earlier, a parent class and a child class can have methods with the same name, return type, and the same number of arguments.If we want to call the method existing in the parent class, we can use the super keyword. The main purpose of the superkeyword is to resolve ambiguity.
Let’s understand how to call a parent class method using a super keyword with the below example:
Car.java
class Vehicle{ void display(){ System.out.println("This is a method of superclass Vehicle"); } } public class Car extends Vehicle{ void display(){ System.out.println("This is a method of subclass Car"); } void msg(){ super.display(); //invokes the parent class method display(); //invokes the child class method } public static void main(String[] args) { Car obj = new Car(); obj.msg(); } }
Output:

In this way, we have learned how to call different types of methods in Java.