Shallow copy in Java
Java's most important task is making a copy or clone of an object. In this part, we'll talk about shallow copies in Java and how to make them of Java objects. The definition of a copy in Java and the distinction between a reference duplicate and an item copy will be covered first before going on to the shallow copy.
According to its name, a reference copy duplicates a reference variable pointing at an object. As an illustration, if we create a reference duplicate of a Byke object with a myByke variable pointing to it, the original object will still exist despite the two myByke variables.
A copy of an object is made of the actual object. So, if we were to copy our byke object once more, we could make a copy of the original and a second reference variable that pointed to it.
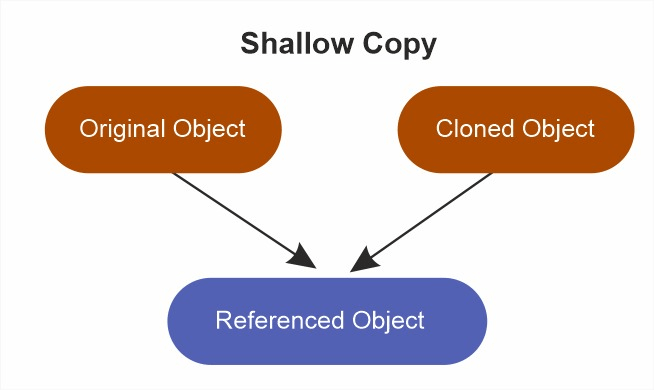
A new object with the same instance variables as the original is said to be a shallow duplicate of the original. For instance, a shallow duplicate of a Set shares objects with the original Set by pointers and comprises the same members as the previous Set. Some claim that shallow copies employ reference meanings.
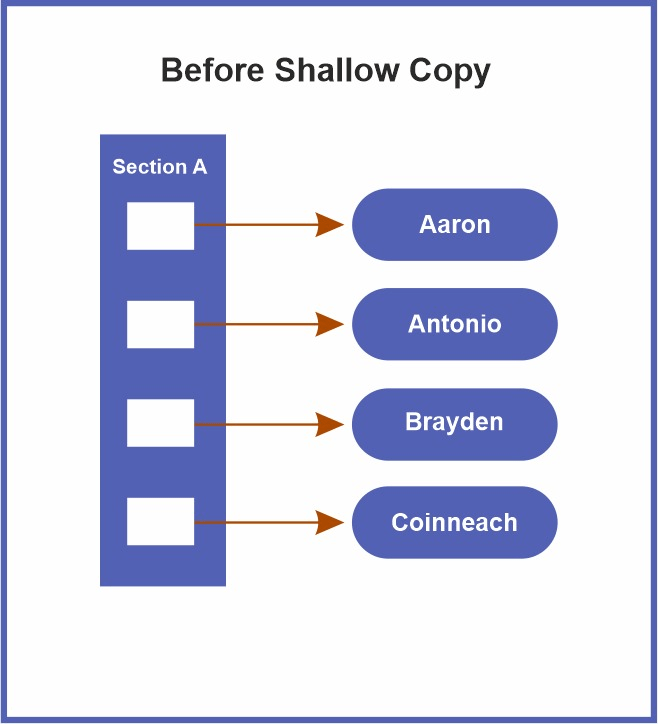
Establishes a fresh reference to the exact memory location. In other words, we might conclude that only references are duplicated in shallow copy. As a result, the original and the object also refer to the same source. It's important to note that any modifications we make to the duplicated object's information also affect the original.
We copy all fields from the original objects whenever we generate a copy of an object that uses a shallow copy technique. However, only references to the objects, not the actual objects, are duplicated if it includes objects as the fields. It's important to note that only primitive data types are transferred but not the object references.

Note: clone() method can default create the shallow object.
Take a look at the following code example.
Public class ShallowCopy
{
private int[] d;
//this method will create a shallow copy
public ShallowCopy(int[] value)
{
d= value;
}
public void displayData()
{
System.out.println(Arrays.toString(d) );
}
}
Java Program for shallow copy
ShallowExample.java
//This program is for shallow copy in java
//import section
import java.io.*;
import java.util.*;
//creating the People class
class People implements Cloneable
{
//a lower level of object
private Bike bike;
private String names;
public Bike getBike()
{
return bike;
}
public String getNames()
{
return names;
}
public void setName(String str)
{
names = str;
}
public People(String str, String temp)
{
names = str;
bike = new Bike(temp);
}
public Object clone()
{
//shallow copy
try
{
return super.clone();
}
catch (CloneNotSupportedException e)
{
return null;
}
}
}
class Bike
{
private String names;
public String getNames()
{
return names;
}
public void setNames(String str)
{
names = str;
}
public Bike(String str)
{
names = str;
}
}
//main section
public class ShallowExample
{
public static void main(String args[])
{
//Original Object of the class People
People p1 = new People("People-A", "Civic");
System.out.println("Exact Values (orginal values): " + p1.getNames() + " - "+ p1.getBike().getNames());
// the clone contains the shallow object
People q1 = (People) p1.clone();
System.out.println("Clone (the changes before): " + q1.getNames() + " - "+ q1.getBike().getNames());
//the primitive member of the clone has been changed
//q1.setNames("People-B");
//the lower level object has been changed
q1.getBike().setNames("Mossiac");
System.out.println("Clone (after change): " + q1.getNames() + " - "+ q1.getBike().getNames());
System.out.println("Original (the values after the modification of the clone object): " + p1.getNames()+ " - " + p1.getBike().getNames());
}
}
Output

ShallowExample2.java
//This program is for shallow copy in java
//import section
import java.io.*;
import java.util.*;
class XYZ
{
// an instance variable n is created for the class XYZ
int n = 30;
}
public class ShallowExample2
{
// Main method of the program
public static void main(String argvs[])
{
//creating an object for the class XYZ
XYZ object1=new XYZ();
//the reference will be created, but the value is not copied
XYZ object2 = object1;
// the value is updated to 10
// using the reference variable object2
object2.n=10;
//the result is printed using the reference variable object1
System.out.println("The number value is : " + object1.n);
}
}
Output

Shallow Copy Vs. Deep Copy
Shallow copy | Deep copy |
Original objects and cloned objects really aren't entirely distinct. | Original items and cloned items don't connect at all. |
Cloned objects can be modified and then imitated in original objects, or vice versa. | An original thing can be altered and replicated in turn, or vice versa. |
The clone() method's default implementation produces a shallow duplicate of an object. | We must override the clone() function to construct the deep duplicate of an object. |
A shallow copy is recommended if such an object contains just primitive fields | . Should use a deep duplicate if an item contains references to those other items as fields. |
It is much less costly and also quick. | The Deep is very slow, and it is more expensive. |
In Shallow copy, memory utilization is very efficient. | As in the case of Deep copy, the memory utilization is not upto the mark. |
It is very much efficient to error-prone. | It is not efficient to error-prone. |