Java Control Statements
Control Statements:
Control statements in Java can also be referred to as decision-making while dealing with different problems. Control statements are helpful to sort out the flow of the program or build up the logic required for the program.
In Java, control statements are sub-categorized into three parts. They are:
1. Design making statements
2. Looping Statements
3. Jump statements
Decision-making statements:
With name specification, we can understand the decision-making statements easily. Decision-making statements are the single-step execution statement that is executed when the specific condition is satisfied.
These statements are divided into two types. They are:
- if and else statements
- Switch statements
if and else statements:
Generally, “if” and “else” statements come in pairs. An “if" statement can be executed without an else statement, but vice versa is not true.
The purpose of the "if" statement is to check whether the given condition is true or not. If the given condition is true, then the compiler proceeds with the "if" block; otherwise, it executes the "else” block.
“else if” is used when there are more than one or two conditions, and else if is executed when the previous “if” condition is wrong.
"else" block is executed at last when all the conditions given in the if and else if statements are wrong.
Syntax for simple if statement:
if (condition )
{
Statement 1; // statements will be executed
Statement 2;
.
.
Statement n;
}
Syntax for if and else statement:
if (condition )
{
Statement 1; //statements will be executed if the condition is true
}
else
{
Statement 1;
}
Syntax for if and else if statement:
if (condition)
{
Statement 1; //statements will be executed if the condition is true
}
else if (condition 2)
{
Statement 1; //statements will be executed if the condition is true
}
else
{
Statement 1;
}
Example: consider the following code
import java.io.*;
import java.util.*;
class IfDemo
{
public static void main (String args[])
{
Scanner sc = new Scanner (System.in);
int a = sc.nextInt ();
if (a > 18)
{
System.out.println (“Person is eligible for voting ”);
}
else if (age < 0)
{
System.out.println (“Age cannot be negative,
please enter the correct age”);
}
else
{
System.out.println (“ Person is not eligible for voting”);
}
}
}
You can check the below-attached screenshots for the above code for different test cases.
Output 1:
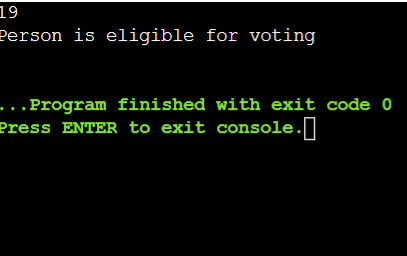
Output 2:
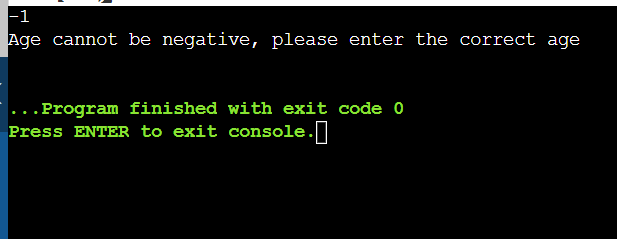
Output 3:
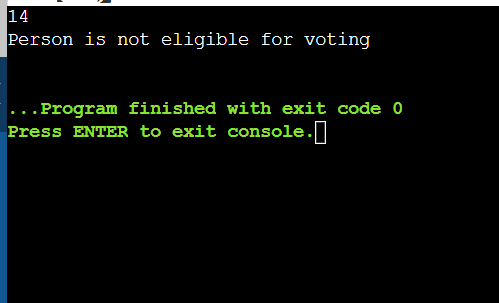
Nested if Statement
In nested if statements, we generally tend to write if or else if statement inside the previous if block.
Syntax for nested if:
if (condition )
{
if (condition ) // block will be executed if condition is true
{
Statement 1; //statements will be executed if the condition is true
}
else
{
Statement 1;
}
}
else
{
Statement 1;
}
Example:
import java.io.*;
import java.util.*;
class NestedIfDemo
{
public static void main (String args[])
{
int a = 63;
int b = 93;
if( a == 63 )
{ // executes if the condition is true
if( b == 93 )
{// executes if the condition is true
System.out.print("a = 63 and b = 93");
}
}
}
}
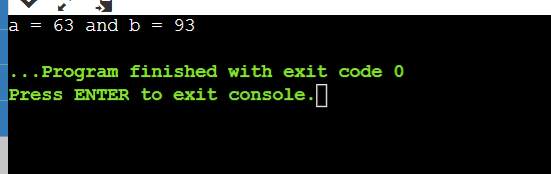
Switch Statement:
Switch statements in Java are comparable to if-else-if statements. According to the variable being changed, a single case from a collection of code blocks called cases in the switch statement is executed. Instead of using if-else-if statements, use the switch statement instead. Additionally, it makes the program easier to read.
NOTE:
- Case variables can take the form of an enumeration, byte, char, int, or short. Java version 7 now supports the string type as well.
- Cases cannot be duplicated.
- When any given case doesn't match the value of the expression, the default statement is carried out. It's not required.
- When the condition is met, the switch block is terminated by a break statement.
- If it is not used, the next case is handled.
- We must be aware that when using switch statements, the case expression will share the same type as the variable. It will, nonetheless, have a constant value.
Syntax for nested if:
switch (expression){
case 1:
statement1;
break;
case 2:
statement1;
break;
.
.
.
case N:
statementN;
break;
default:
default statement;
}
Example:
import java.io.*;
import java.util.*;
public class SwitchDemo
{
public static void main(String[] args) {
Scanner sc = new Scanner ( System.in);
System.out.println("enter the value of number");
int num = sc.nextInt();
switch(num)
{
case 0:
System.out.println("number is 0");
break;
case 1:
System.out.println("number is 1");
break;
default:
System.out.println("number is "+num);
}
}
}
Output 1:
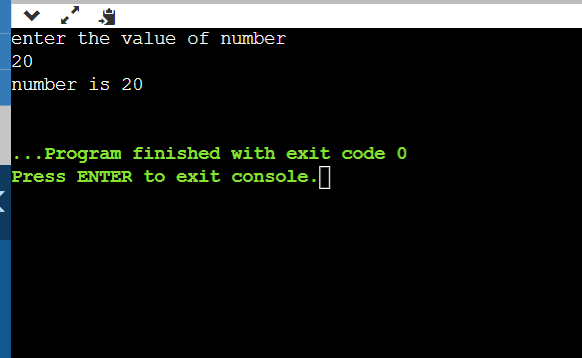
Output 2:
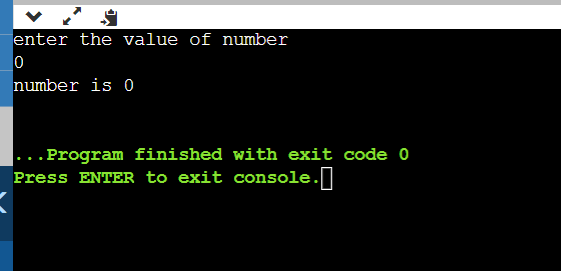
While dealing with case expression, we must notice that case expression will be the same type as the variable. And it will be a constant value.
Looping Statements:
While dealing with different problems, sometimes we require to execute or run a specific block repeatedly. In this case, we use looping statements.
Lopping statements are further divided into two types. They are
- Entry control loops
- Exit control loops
Entry control loops:
The test condition is checked first in an entry-controlled loop, then the body of the loop is executed. The loop body won't be executed in an entry-controlled loop if the condition is false.
Entry-controlled loops are used when a test condition must be verified before the loop body is executed.
Examples for entry control loop:
1. for loop
2. while loop
3. for-each loop
The “for” loop:
While dealing with for loop, we just write one line of code. We can initialize the loop variable, verify the condition, and increment or decrement in this line. Then the body of the loop is carried out with the main logic.
Generally, for loop is used when the number of iterations count is fixed.
Syntax for “for” loop:
for (initialization; condition; updation)
{
//Executable block of statements
}
Example: program to print 1 to n numbers
import java.io.*;
import java.util.*;
public class ForDemo
{
public static void main(String[] args) {
Scanner sc = new Scanner ( System.in);
System.out.println("enter the value of number");
int num = sc.nextInt();
for(int i=0; i <= num; i++)
{
System.out.println("The number is "+i);
}
}
}
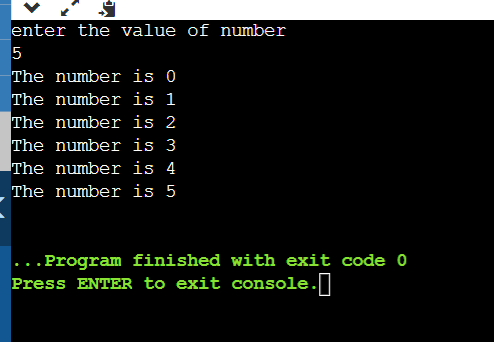
While Loop:
The while loop is also used to repeatedly loop through the number of statements. However, it is advised to use a while loop if we are unsure of the number of iterations. In contrast to for loop, while loop's initialization and increment/decrement operations do not happen inside the loop statement.
Since the condition is verified at the beginning of the loop, it is also referred to as the entry-controlled loop. If the condition is satisfied, the body of the loop will be run; otherwise, the statements following the loop will be run.
Syntax for “While” loop:
Datatype variable; //initialization
while(condition)
{
Statements;
Updating;
}
import java.io.*;
import java.util.*;
public class WhileDemo
{
public static void main(String[] args) {
Scanner sc = new Scanner ( System.in);
System.out.println("enter the value of number");
int num = sc.nextInt();
int i=0;
while(i<=num)
{
System.out.println("The number is "+i);
i++;
}
}
}
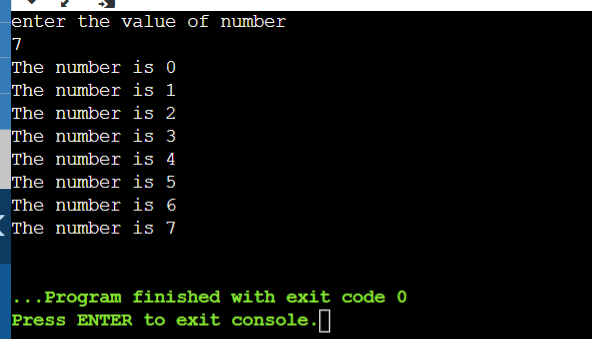
for-each loop:
Java offers an improved for loop for iterating through data structures like collections and arrays. We don't need to update the loop variable during the for-each loop.
Syntax for “for-each” loop:
for (datatype variable: array_name / collection_name)
{
/ /statements
}
Example:
import java.io.*;
import java.util.*;
public class ForEachDemo
{
public static void main (String[] args)
{
String [] months = {"Jan","Feb","Mar","Apr","May"};
System.out.println("Printing the array months:\n");
for(String month: months)
{
System.out.println(month);
}
}
}
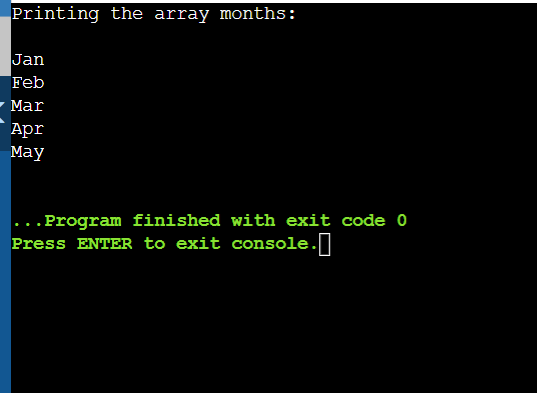
Exit control loop:
If the test condition is false, the loop body will only be executed once in an exit-controlled loop. It starts with the loop body and ends with the condition. When the test condition must be verified after execution, an exit-controlled loop is used.
The best example for exit controlled loop is a do-while loop.
do-while loop:
The do-while loop executes the loop statements before checking the condition at the end of the loop. Use a do-while loop if the number of iterations is unknown, but the loop must be run at least once.
Syntax for “do-while” loop:
do
{
// executable block of statements
} while (condition);
Example:
import java.io.*;
import java.util.*;
public class DoWhileDemo
{
public static void main (String [] args)
{
Scanner sc = new Scanner (System.in);
System.out.println("enter the value of number");
int num = sc.nextInt();
int i = 0;
do
{
System.out.println ("The number is "+i);
i++;
} while (i < num);
}
}
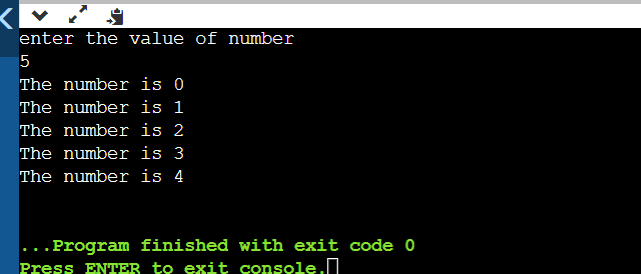
Jump Statements:
A jump statement can be used to alter the program's execution flow. They are also referred to as branching statements because they cause a different branch in the execution flow.
Jump statements are further divided into the following types. They are:
1. Break Statements
2. Continue Statements
Break Statements:
Break Statements are used with looping statements like for loop, while loop, or do while loop.
When a break statement is used inside a loop of a program, the loop is terminated immediately, and the execution proceeds with the next blocks of the program.
Generally, the break statement is either used with loops or a switch statement. It breaks the flow of the program under specific conditions. If it is used in nested loops, then it will break the loop in which it is mentioned. Let us understand the break statement with the following program.
Example program:
import java.io.*;
import java.util.*;
//consider the following program
//Java Program to demonstrate the use of break statement
class BreakExample {
public static void main(String[] args) {
Scanner sc = new Scanner (System.in);
System.out.println("enter the value");
int n = sc.nextInt();
//using for loop
for(int i=1;i<=n;i++)
{
if(i==n/2) // stopping condition or break condition
{
break;//jump statement
//the given condition prints the statements
//from 1 to n values
}
System.out.println(i);
}
}
}
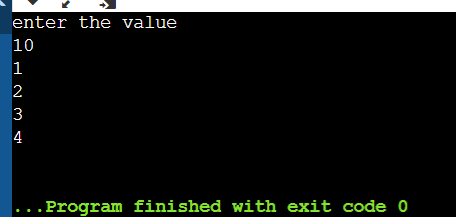
Continue Statement
Continue statement is a part of jumping statements in Java. It is used to skip a particular part of code.
It skips some part of the code when the continue statement is passed in that block of code.
It can be used with for loop or while loop. When the continue statement is used, it will be evaluated, the remaining code is skipped at the required condition, and the program's current flow is continued. It only continues the inner loop when there is one.
Example program:
import java.io.*;
import java.util.*;
//consider the following program
//Java Program to demonstrate the use of continue statement
class ContinueExample {
public static void main(String[] args) {
Scanner sc = new Scanner (System.in);
System.out.println("enter the value");
int n = sc.nextInt();
//using for loop
for(int i=1;i<=n;i++)
{
if(i==n/2) // skiping condition or continue condition
{
continue;//jump statement
//the given condition prints the statements
//from 1 to n values
}
System.out.println(i);
}
}
}
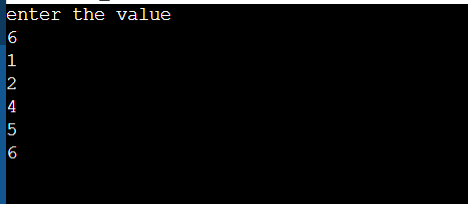