Java callable example
What is callable in Java?
Callable is an interface that is present in Java. There are two methods for constructing threads: one is to inherit the Thread class, while the other is to combine a Runnable with a thread. The inability to force a thread to return results when it ends or when run () is finished is a feature that Runnable lacks. The Callable interface is introduced in Java to facilitate this functionality.
Callable example:
Let us create a java code that implements the callable interface.
Let's look at the snippet of code that uses the Callable interface and, after a delay of between 0 and 5 seconds, produces a random integer between 0 and 20.
CallableExample.java
// A Java code for the Callable interface implementation
// For generating random numbers between 0 - 20
// Importing the packages required.
import java. util. concurrent. Callable;
import java.util.Random;
class Jtp implements Callable {
public Object call () throws Exception {
Random obj = new Random ();
// Object creation for the Random class using new
Integer num = obj.nextInt(20);
// generating a random number between 0 to 20 using nextInt method.
Thread.sleep(num * 1000);
// the thread is kept in sleep mode for some random time
return num;
}
}
The callable interface is demonstrated in the previous sample of code. But the work is not yet finished. The returned object, accessible to the main thread, must be stored after the call () method exits. It is important as the main thread must know the call () method returning value. The same goal is achieved by using a Future object. The response from a separate thread is sent from the call () function and is stored in a Future object.
Future is an application just like Callable. Therefore, it must be put into practice to be used. The Future interface does not, however, need to be manually implemented. The FutureTask class from the Java library already implements the Runnable and Future interfaces.
CallableExample1.java
// A Java code for the Callable interface implementation
// For generating random numbers between 0 - 20
import java. util. concurrent. Callable;
import java.util.Random;
import java.util.concurrent.FutureTask;
// importing the packages required.
Class JCal implements Callable
{
@Override
public Object call () throws Exception
{
Random obj = new Random ();
// Object creation for the Random class
Integer num = obj.nextInt(10);
// generating a random number between 0 to 9 using nextInt method.
Thread.sleep(num * 1000);
// the thread is kept in sleep mode for some random time
// returning the object created that contains a random number
return num;
}
}
public class CallableExample1 {
// start of the main method
public static void main (String args[]) throws Exception {
// creating an array of 5 objects of the FutureTask class
FutureTask[] treads = new FutureTask[10];
// using a loop for creating and running 10 threads
for (int j = 0; j < 10; j++)
{
// Object creation for the JavaCallable class
Callable cobj = new JavaCallable();
//Creation of the FutureTask reference with Callable object
treads[j] = new FutureTask(cobj);
Thread tobj = new Thread(treads[j]);
tobj.start();
}
// Using a loop for generating the random numbers
for (int i = 0; i < 10; i++)
{
// Calling the get () method to invoke the thread
Object ojb1 = treads[i]. get ();
// While waiting for the result, the get method maintains control.
//When the method is disrupted, the get method may throw the checked exception.
// Due to this, we must include the throws statement in the main method.
// printing the numbers generated randomly
System.out.println("Random number generated is: " + obj1);
}
}
}
Output:
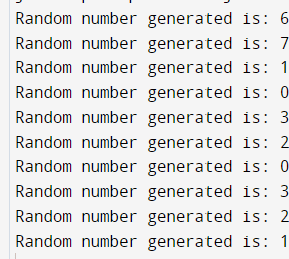
We created 10 separate threads in the code. The call () method is called by each thread, which generates a random number and returns it. The main thread receives the generated random number object from the various threads using the get () function. The FutureTask class implements the get () method, defined in the Future interface.
Callable Interface:
- If we are working with the Callable interface, the call () method must be defined.
- An Object is the return type of the interface's call () function. Consequently, an Object is returned by the call () function.
- An exception may be thrown by the call () method.
- The Callable interface does not allow for the Creation of threads.