Types of Logical Operators in Java
In this tutorial, we are going to study logical operators. A logical operator is an operator that accomplishes a logical operation that is to connect two or more operations. These operators are of great importance in java as it helps to perform several crucial operations in java.
We will understand the types of logical operators with the aid of certain examples as well as some programs.
With the help of logical operators, one can execute logical “AND”, “OR” and “NOT” operations, just like “AND”, “OR” and “NOT” operations in digital electronics.
The gist of three logical operators.
- AND Operator ( && ) – if( a && b ) [if both the conditions are true, it executes otherwise it doesn’t]
- OR Operator ( || ) – if( a || b) [if one of the conditions is true, it executes otherwise it doesn’t]
- NOT Operator ( ! ) – !(a<b) [It returns false if the value of a is less than that of b]
Now, let us try to understand each of the three operators in detail through examples and java code.
AND Operator
Syntax - if(a && b )
Here, a refers to condition 1 and b refers to condition 2.
Itreturns only if both the conditions are true, otherwise, it doesn’t.
Its symbol is &&.
Example
x = 12, y = 15, z = 22
To check if the variable z is the largest among the three.
If both of these conditions are satisfied, only then we can conclude that z is the largest.
if(z > x && z > y)
Condition 1: if (z > x) // (22 > 12) // TRUE
Condition 2: if (z > y) // (22 > 15) // TRUE
Therefore, z is the largest.
If both of these conditions are satisfied, only then we can conclude that z is the largest.
Truth Table:
a | b | a && b |
1 | 1 | 1 |
1 | 0 | 0 |
0 | 1 | 0 |
0 | 0 | 0 |
- If condition 1 and condition 2 are true, the resultant is also true.
- If condition 1 is true and condition 2 is false, the resultant is false.
- If condition 1 is false and condition 2 is true, the resultant is false.
- If condition 1 is false and condition 2 is false, the resultant is false.
Implementation
Now, we will see a java program to understand the concept even better.
Example 1:
// Java code to illustrate the working of
// logical AND operator
import java.io.*;
class Logical_operator {
public static void main(String[] args)
{
// initializing the variables
int a1 = 35, a2 = 45, a3 = 12, a4 = 0;
// Displaying a, b, c
System.out.println("Variable 1 = " + a1);
System.out.println("Variable 2 = " + a2);
System.out.println("Variable 3 = " + a3);
// using the logical AND to verify
if ((a1 < a2) && (a2 == a3)) {
a4 = a1 + a2 + a3;
System.out.println(" sum = " + a4);
}
else
System.out.println(" conditions are False ");
}
}
Output:
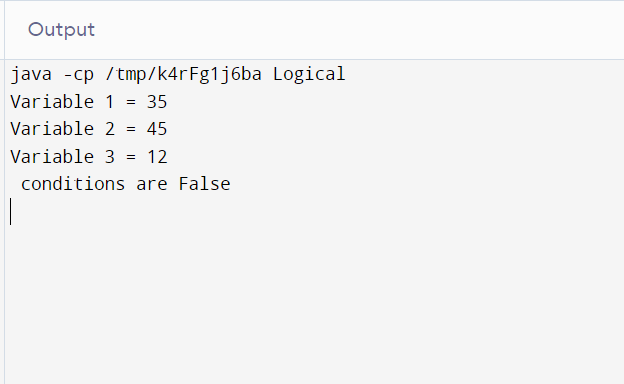
Explanation: In this java code, an illustration of the logical AND operator is shown.
Example 2:
import java.io.*;
class shortCircuit_effect {
public static void main(String[] args)
{
// initializing the variables
int a1 = 30, a2 = 40, a3 = 36;
// printing the value of variable b
System.out.println("Value of a2 : " + a2);
// Using logical AND operator
// Short-Circuiting effect as condition 1 is
// incorrect, thus condition 2 is never been touched
// and so ++a2 (pre-increment) doesn't take place and
// the value of a2 remains unchanged
if ((a1 > a3) && (++a2 > a3)) {
System.out.println("Inside the if block");
}
// Printing the value of a2
System.out.println("Value of a2 : " + a2);
}
}
Output:
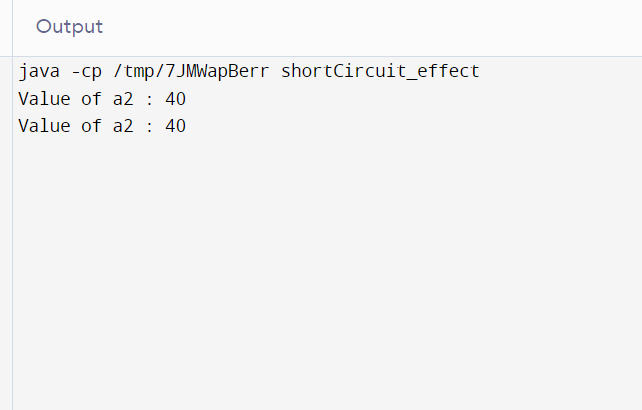
Explanation: In this java code, an illustration of the logical AND operator is shown. Here, the case of the short circuit effect is illustrated.
OR Operator
Syntax – if(a || b)
Here, a refers to condition 1 and b refers to condition 2.
Itreturns even if the only condition is true. If the first operand is true, it doesn’t even check the second operand.
Its symbol is ||.
Example
x = 5, y = 8
z = (x > 0 || y > 0) // (5 > 0 || 8 > 0)
5 > 0 // TRUE
The first condition is true, so the resultant is true and there is no need to check the second condition.
Truth Table:
a | b | a && b |
1 | 1 | 1 |
1 | 0 | 1 |
0 | 1 | 1 |
0 | 0 | 0 |
If condition 1 and condition 2 are true, the resultant is also true.
If condition 1 is true and condition 2 is false, the resultant is true as the working of the OR operator is such that even if one of the conditions is true, the output is also true.
If condition 1 is false and condition 2 is true, the resultant is true.
If condition 1 is false and condition 2 is false, the resultant is false.
Implementation:
Now, we will see a java program to understand the concept even better.
// Java code to understand the working
// logical OR operator
import java.io.*;
class Logical_operator {
public static void main(String[] args)
{
// initializing variables
int a1 = 35, a2 = 45, a3 = 12, a4 = 0;
// printng the values of a1, a2, a3
System.out.println("Variable 1 = " + a1);
System.out.println("Variable 2 = " + a2);
System.out.println("Variable 3 = " + a3);
System.out.println("Variable 4 = " + a4);
// logical OR operators being used
if (a1 > a2 || a3 == a4)
System.out.println("Either of the two conditions are true");
else
System.out.println("Neither of the two conditions are true");
}
}
Output:
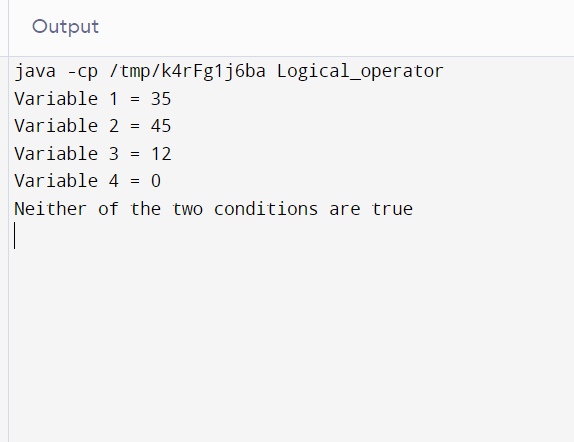
Explanation: In this java code, an illustration of the logical OR operator is shown.
NOT Operator
Syntax -!(condition)
If the value is true, it will return false, and vice versa.
Its symbol is !.
Example
y = !(0)
output – 1
y = !(x == 5)
output - 0
a = 5, b = 7
!(a<b) // !(5 < 7) // !(true) // false
It complements the result. It gives out false if the result was true and true if the result was false.
Truth Table:
a | b |
0 | 1 |
1 | 0 |
Implementation:
Now, we will see a java program to understand the concept even better.
// Java code to understand the working of
// logical NOT in java
import java.io.*;
class Logical {
public static void main(String[] args)
{
// initializing the variables
int a1 = 22, a2 = 1;
// Printing the values of a1 and a2
System.out.println("Variable 1 = " + a1);
System.out.println("Variable 2 = " + a2);
// logical NOT operator being used
System.out.println("!(a1 < a2) = " + !(a1 < a2));
System.out.println("!(a1 > a2) = " + !(a1 > a2));
}
}
Output:
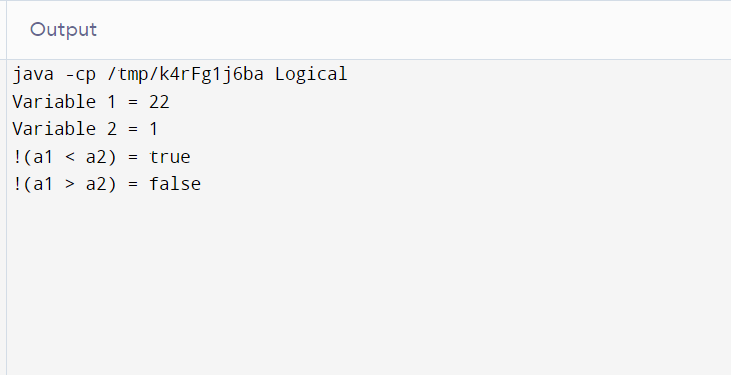
Explanation: In this java code, an illustration of the logical NOT operator is shown.
Example of Logical Operator
Illustrating the working of logical AND, logical OR, and logical NOT operators together in one java program.
class Logical_operators {
public static void main(String[] args) {
int a = 4, b = 6;
// illustrate the working of && operator
System.out.println("Outputs of AND operator");
boolean x = ((a > b) && (a > b));
System.out.println(x);
x = ((a > b) && (a < b));
System.out.println(x);
// illustrate the working of || operator
System.out.println("Outputs of OR operator");
x = (a < b) || (a > b);
System.out.println(x);
x = (a > b) || (a < b);
System.out.println(x);
x = (a < b) || (a < b);
System.out.println(x);
x = (a > b) || (a > b);
System.out.println(x);
// illustrate the working of! operator
System.out.println("Outputs of NOT operator");
x = !(a == b);
System.out.println(x);
x = !(a > b);
System.out.println(x);
}
}
Output:
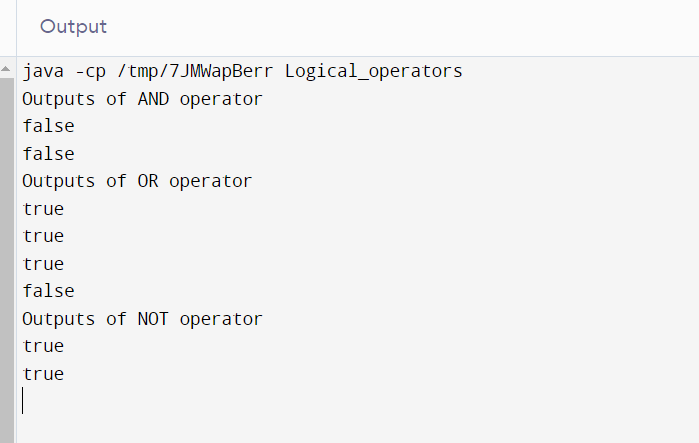
Explanation: In this java code, an illustration of the logical AND, logical OR, and logical NOToperatorsare shown.
Summary
This tutorial was all about the types of logical operators in Java language. We saw the three logical operators namely – logical AND, logical OR, and logical NOT. We saw the syntax, truth table, and working of each of these operators and finally, undertook a practical approach for which we wrote java codes to understand the concept even better.