Hybrid Inheritance in Java
The most crucial OOPs concept in Java is inheritance, which enables the transfer of a class's properties to another class. It describes the Is-A relationship generally. We can create a new class by deriving from an existing one using the inheritance mechanism. The following four types of inheritance are supported by Java:
- Single Inheritance
- Multi-level Inheritance
- Hierarchical Inheritance
- Hybrid Inheritance
Only hybrid inheritance in Java will be covered in this section, along with appropriate examples and various hybrid inheritance implementation strategies.
Hybrid Inheritance
Hybrid (mixture) often refers to something that is composed of multiple elements. A hybrid inheritance in Java is made up of two or more different inheritance types. The fundamental goal of hybrid inheritance is to modularize the code into simple classes. It also offers code reuse. The following combinations can be used to achieve hybrid inheritance:
- Multiple and Single Inheritance (not supported but can be achieved through an interface)
- Hierarchical and Multilevel Inheritance
- Single and Hierarchical Inheritance
How does hybrid inheritance work in Java?
There are four classes, let's say A, B, C, and D. Assume that class "C" is extended by class "A" and "B." Additionally, class "A" is extended by class "D". In this case, class "A" serves as both a parent class for class "D" and a child class for class "C." The illustration helps us to comprehend the example.
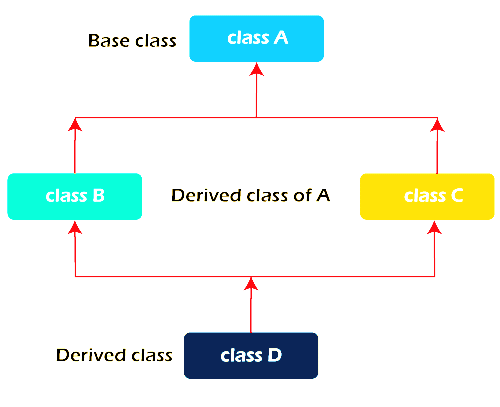
Single and Multiple Inheritance
By combining single inheritance with multiple inheritances in the following Java program, we were able to accomplish hybrid inheritance (through interfaces).
We used the human body as an example in our software because it has many distinct functions, like walking, talking, dancing, and eating. Male or female human bodies are both possible. Therefore, the HumanBody class has two interfaces, Male and Female. The features of the HumanBody class, which represents a single inheritance, are inherited by both the parent class and the child class.
Let's say a Male and a Female decide to have a child. Therefore, the functionality of the Male and Female interfaces is also inherited by the Child class. It is an illustration of multiple inheritances.
Filename: Child.java
public class Child extends HumanBody implements Male, Female
{
public void show()
{
System.out.println("Implementation of show() method defined in interfaces Male and Female");
}
public void displayChild()
{
System.out.println("Method defined inside Child class");
}
public static void main(String args[])
{
Child obj = new Child();
System.out.println("Implementation of Hybrid Inheritance in Java");
obj.show();
obj.displayChild();
}
}
class HumanBody
{
public void displayHuman()
{
System.out.println("Method defined inside HumanBody class");
}
}
interface Male
{
public void show();
}
interface Female
{
public void show();
}
Output
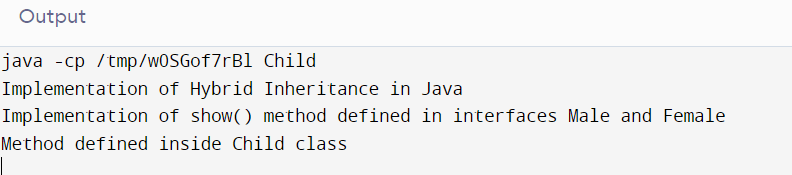
Using Multilevel and Hierarchical Inheritance
Grandfather is shown as a super class in the following diagram. The GrandFather class's properties are passed down to the Father class as a single inheritance is represented by Father and Grandfather. The Son and Daughter classes also inherit from the Father class. Father now serves as the Son and Daughter's parent class. These classes represent hierarchical inheritance. Together, they signify hybrid inheritance.
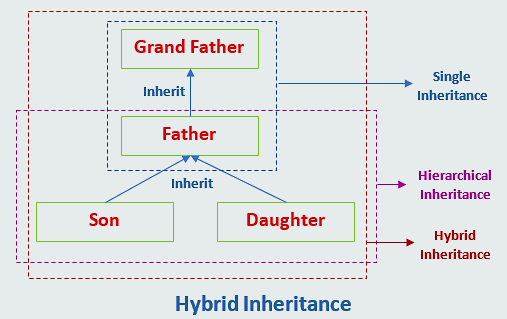
Let's use a Java program to implement the hybrid inheritance approach.
Filename: Daughter.java
//inherits Father's properties
public class Daughter extends Father
{
public void showD()
{
System.out.println("She is daughter.");
}
public static void main(String args[])
{
//Daughter obj = new Daughter();
//obj.show();
Son obj = new Son();
obj.showS(); // Accessing Son class method
obj.showF(); // Accessing Father class method
obj.showG(); // Accessing GrandFather class method
Daughter obj2 = new Daughter();
obj2.showD(); // Accessing Daughter class method
obj2.showF(); // Accessing Father class method
obj2.showG(); // Accessing GrandFather class method
}
}
//parent class
class GrandFather
{
public void showG()
{
System.out.println("He is grandfather.");
}
}
//inherits GrandFather's properties
class Father extends GrandFather
{
public void showF()
{
System.out.println("He is father.");
}
}
//inherits Father's properties
class Son extends Father
{
public void showS()
{
System.out.println("He is son.");
}
}
Output
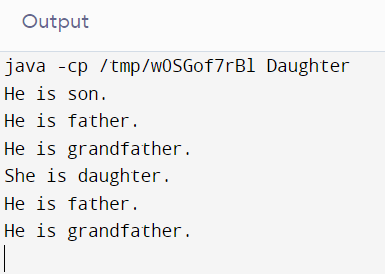
Hierarchical and Single Inheritance
By combining hierarchical and single inheritance in the Java program that follows, we were able to accomplish hybrid inheritance.
Here, classes A and B extend to class C, which stands for hierarchical inheritance. class D extends class A, which stands for single inheritance.
Filename: D.java
public class D extends A
{
public void disp()
{
System.out.println("D");
}
public static void main(String args[])
{
D obj = new D();
obj.disp();
}
}
class C
{
public void disp()
{
System.out.println("C");
}
}
class A extends C
{
public void disp()
{
System.out.println("A");
}
}
class B extends C
{
public void disp()
{
System.out.println("B");
}
}
Output
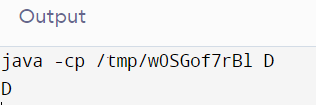