How to Develop Programming Logic in Java?
Introduction
In the world of software development, Java programming language is one of the most powerful programming languages that is used to create a wide range of applications. It includes desktop, web, and mobile applications. For programmers to be able to understand and resolve complex problems in Java, it is exceptionally critical for them to establish solid programming logic that is easy to understand. The use of modern technologies and languages, as well as adapting to technological changes, requires programmers to write efficient and effective code.
One of the main benefits of Java is that it is platform-independent, meaning that Java code can run on a wide range of platforms, including Windows, macOS, and Linux, without modification. It is made possible using the Java Virtual Machine (JVM), which acts as a bridge between the Java code and the underlying platform.
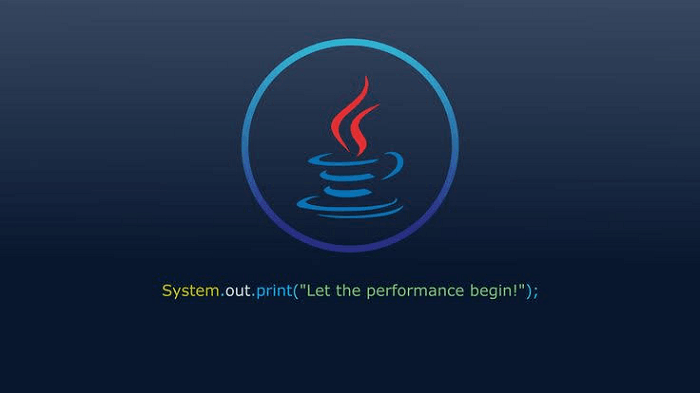
When you start to write your java code, it's essential to use good programming practices such as commenting on your code and using meaningful variable names. It will make it easier for others (or your future self) to understand and maintain the code. Additionally, it's essential to test your code and debug any errors that may occur. It will help you to ensure that the final solution is correct and behaves as expected. Overall, developing programming logic in Java requires understanding the problem, analyzing and designing solutions, and proficiency in Java syntax and constructs.
5 Ways to Develop Programming Logic in Java
1. Understand the basics of Java
As a programmer, you need to be familiar with a few basic concepts and constructs that you will need to know to develop programming logic in Java:
- Variables: In Java, a variable is a named location in memory where one can store a value. Before you use a variable in a program, you must declare it by specifying its data type and name. For example, to declare an integer variable named "x," you would use the following syntax:
int x ;
- Data types: Java has various built-in data types, such as int for integers, double for decimal numbers, and boolean for true/false values. Other commonly used data types include String for storing sequences of characters and char for storing individual characters.
- Operators: Operators are used to performing operations on variables and values. Common operators in Java include mathematical operators (+, -, *, /) and comparison operators (==, !=, >, <, >=, <=). There are also bitwise operators, logical operators, and ternary operators.
- Control structures: Control structures are used to control the flow of a program's execution. Common control structures in Java include if-else statements, for loops, while loops, and do-while loops. These structures help control the flow of a program and perform different actions based on various conditions.
- Methods: A method is a block of code that can be reused throughout a program. They can take parameters as input and return a value as output. In Java, methods are declared with the keyword "void" if they do not replace a deal, or with the data type of the value they produce.
- Arrays: An array is a collection of variables with the same data type, which can help store large amounts of data in a sequence and can be accessed using an index. Java supports single and multi-dimensional arrays.
- Exception handling: Java includes a built-in mechanism for handling errors and exceptions, which allows a program to recover from unexpected events and continue running. It helps to prevent the program from crashing and will enable it to continue running even in the presence of errors.
If you can grasp these basic concepts and constructs of Java language, you can develop your programming logic in Java by following these steps.
Furthermore, practicing and experimenting with sample codes and small projects is one of the most effective ways to improve your programming logic skills. It is in addition to improving your ability to implement them. When you become more comfortable with the language, you can begin with simple programs and gradually increase the complexity as you become more proficient at it.
Understand the Object-Oriented Programming
A programming paradigm based on objects, which are instances of classes, is called object-oriented programming (OOP). To develop programming logic in Java using OOP, you should focus on understanding and applying the following concepts in detail:
- Classes and objects: A class is a blueprint for an object, an instance of the class. It can be defined using the class keyword and can include properties (also known as fields or member variables) and methods (also known as functions or member functions). You can instantiate an object using the new keyword followed by the class name and the constructor. In addition, Java provides four access modifiers you can use on class members: public, private, protected, and package private. They control the access of the class members to other classes and outside the box.
- Inheritance: Inheritance allows you to create a hierarchy of classes, where a subclass inherits the properties and methods of its superclass. The subclass can also add or override properties and methods of its superclass. Using inheritance, you can model real-world relationships like "is-a" and "kind of" and reuse existing code. By polymorphism, objects belonging to different classes are treated as belonging to the same superclass. Who can achieve it through interfaces and abstract classes? The critical idea of polymorphism is that you can write code that can work with many different types of objects without needing to know the specific object type at compile time.
- Encapsulation: Encapsulation is the process of hiding the implementation details of a class and controlling access to its properties and methods. Who can achieve it through access modifiers, such as private, protected, and public? Information hiding is the practice of hiding the internal details of an object, such as its data, and exposing only the methods that operate on the data. It helps to protect the internal state of the thing and ensures that Who can only change it in a controlled and predictable way.
- Polymorphism: polymorphism refers to the ability of an object or reference to take on many forms. It allows for a single object or method to be used in various ways, depending on the context in which it is used. Polymorphism is implemented through interfaces, abstract classes, and approach overriding. An object can be of multiple types, as it can inherit from various classes or implement multiple interfaces. This polymorphism feature allows objects to be treated as their superclass or interface types, enabling standard methods for objects of different classes. In Java, polymorphism is achieved by declaring a method with the same name in multiple classes. The Java runtime then determines which method to call based on the actual object type at runtime. It allows the same method to perform different actions depending on the object's class.
- Interfaces: An interface defines a set of method signatures but no implementation. There can be more than one class that implements an interface. One can use interfaces to create more flexible and reusable code by allowing you to specify a class's behavior without limiting how the behavior is implemented.
- Design patterns: These are reusable solutions to common programming problems. Understanding these patterns can help you to write more efficient and elegant code. Some common design patterns in Java include the Singleton pattern, which ensures that a class has only one instance; the Factory pattern, which creates objects without specifying their classes; and the Observer pattern, which allows objects to be notified of changes in other objects. Study and understand the patterns, and learn when and how to use them and when to not.
- Exception handling: Exceptions are events that occur during the execution of a program that disrupts the normal flow of code. Exception handling is a mechanism for dealing with errors and exceptions in a controlled and predictable way. Java provides a built-in mechanism for exception handling, using the 'try,' 'catch,' and 'finally' keywords. The 'try' block encloses the code that might throw an exception, the 'catch' block contains the code to handle the exception, and the final block contains always executed, whether an exception is thrown or not. You can also define custom exceptions by creating new classes inherited from the Exception class or one of its subclasses, such as 'RuntimeException' or 'IOException.'
By understanding these concepts and how to apply them in practice, you will be well on your way to developing robust, maintainable programming logic in Java.
Study About the Data Structure and Algorithm
Studying algorithms and data structures is critical to developing programming logic in Java. Algorithms are instructions for solving a specific problem, and data structures are how data is organized and stored in a program. Understanding how to analyze and implement standard algorithms and data structures can help you develop your logical thinking skills in several ways:
Data Structures: Data structures are how data is organized and stored in a program. Common data structures in Java include arrays, linked lists, stacks, queues, trees, and graphs. Each data structure has advantages and disadvantages and is suited for different problems. For example, when studying arrays, you should learn how they are stored in memory, their time and space complexity for various operations, and how to implement them in Java. When studying linked lists, you should learn about the different types of linked lists, such as single and doubly linked lists, and their time and space complexity for further operations. When studying trees and graphs, you should learn about the different types of trees and graphs and their time and space complexity for other operations, such as traversals.
Algorithms: Algorithms are instructions for solving a specific problem. Standard Java algorithms include sorting, searching, and graph algorithms. Each algorithm has its advantages and disadvantages and is suited for different problems. For example, quicksort is a commonly used sorting algorithm that is efficient for large data sets, while a linear search is a simple algorithm suitable for small data sets. Understanding the characteristics and capabilities of different algorithms can help you choose the right one for a given problem and improve your logical thinking skills.
Study the Limitations of Algorithms and Data Structures: Studying the limitations of algorithms and data structures in Java can help you make better decisions about which to use in different situations. Different algorithms and data structures have advantages and disadvantages and may only be suitable for some problems. For example, some algorithms have a worst-case time complexity of O(n^2), while others have a best-case time complexity of O(n log n). Additionally, some data structures have higher memory requirements, making them less suitable for limited memory. Understanding the limitations of different algorithms and data structures can help you choose the best one for a specific problem and improve your logical thinking skills.
Study the use of data structures and algorithms in real-world applications: Studying the use of data structures and algorithms in Java can help you understand how these concepts are applied in practice and improve your ability to write efficient and effective code. Search engines, social media platforms, e-commerce websites, financial institutions, gaming industries, and healthcare providers all use data structures and algorithms in Java to manage and analyze large amounts of data, make more informed decisions, and create more efficient and interactive systems. Some examples include hash tables, inverted indexes, PageRank algorithms, graph algorithms, recommendation algorithms, sorting, searching algorithms, decision tree algorithms, clustering algorithms, pathfinding algorithms, and spatial partitioning algorithms
Time and Space Complexity: Time and space complexity measures how efficient an algorithm or data structure is in terms of the number of operations it performs and the amount of memory it uses. Understanding the time and space complexity of different algorithms and data structures can help you make informed decisions about which to use in a given situation. It can help you develop your logical thinking skills by forcing you to think about how different solutions will perform in other conditions.
By studying data structures and algorithms in detail, you can develop a strong understanding of the concepts and techniques used to solve problems in computer science. This understanding can help you grow your logical thinking skills and improve your ability to write efficient and effective code in Java.
Keeping up-to-Date With the Latest Developments:
Keeping up-to-date with the latest developments in programming can help you develop your programming logic in Java. Some ways to do this include:
- Following industry leaders and influencers in the Java community on social media platforms such as Twitter, LinkedIn, and GitHub can help you stay informed about the latest trends, best practices, and developments in Java programming. These experts often share their insights, tips, and experiences, which can help you improve your skills and knowledge.
- Participating in online forums and discussion groups related to Java programming can help you connect with other programmers and developers and learn from their experiences. These groups often have a wealth of knowledge and resources to help you stay current with the latest developments.
- Attending conferences, meetups, and workshops related to Java programming can allow you to hear from experts in the field, learn about new technologies and developments, and network with other programmers and developers.
- Reading blogs, articles, and tutorials on the latest trends, best practices, and developments in Java programming can help you stay informed about the latest advancements and techniques.
- Experimenting with new libraries, frameworks, and technologies as they become available can help you stay up-to-date with the latest developments. It allows you to gain hands-on experience with new tools and helps you understand different technologies' capabilities and limitations.
- Staying update with the latest version of Java and its features.
- Enrolling in online courses or certification programs can give you an in-depth understanding of the latest trends and developments in Java programming.
By keeping up-to-date with the latest developments in Java programming, you can improve your skills, knowledge, and understanding of the language and develop your programming logic. It can help you write more efficient and effective code and stay current with the industry's best practices and standards.
Create a Project
One way to develop programming logic in Java is by creating a project. Here are some steps you can take to create a project:
- Choose a project idea: Choosing a project that aligns with your interests, skills, and experience is a great way to start. It can be anything from a simple command line application to a complex web application. One way to choose a project idea is to start with something simple and gradually increase its complexity as you develop your programming logic. For example, you could start with creating a simple calculator application and then move on to creating a more complex project like a web application.
- Plan your project: Planning your project is essential in development. This plan should include the overall goal of your project, a list of features you want to have, a timeline for completing the project, and a list of technologies and tools you will use. This plan can help you stay on track and meet your project's objectives.
- Research and gather resources: Researching the technologies and tools you will use for your project is essential. Research the best practices, design patterns, and libraries that can help you achieve your project goals. Gather needed resources, such as tutorials, documentation, and sample code. This research and gathering of resources will help you understand the capabilities and limitations of different technologies and tools.
- Start coding: Start coding your project using the technologies and tools you have researched. Keep your plan in mind as you code, and stay organized by using comments and good naming conventions. It is essential to test your code as you go and debug any issues that come up as soon as they are found.
- Test and debug: Testing and debugging your code is essential in the development process. It will ensure that your code is working as intended and fix any bugs that might be present.
- Finalize and submit: Once you have completed your project, finalize it by cleaning up your code, adding documentation, and packaging it for submission. There should be no confusion or ambiguity in code.
- Share your project: Sharing your project with others is a great way to get feedback and improve your programming logic. You can share your project on a website or a coding platform with friends, family, or other developers. It will help you understand the strengths and weaknesses of your code and get suggestions on how to improve it.
- Reflect on your project: Reflect on what you have learned during the development process, and think about what worked well and what didn't. Reflect on your design decisions, and think about what could be improved. This reflection can be a great way to identify areas where you need to improve and make a plan for future development.
Creating a project is a great way to develop programming logic in Java, as it allows you to apply what you've learned to a real-world scenario and helps you understand how to organize and structure code. With each project you work on, you will develop your problem-solving skills, coding skills, and understanding of the language and its features.
Plan the Solution
Planning a solution before developing a programming logic in Java includes a clear understanding of the problem, improved efficiency and performance, easy maintenance and modification, and reduced likelihood of errors. It also helps to improve the scalability and security of the code, as well as make the code more readable and understandable.
To plan the solution for developing a programming logic in Java in detail, you can use the following steps:
- Understand the problem statement: Understand the requirements of the problem and what inputs and outputs are expected. Take the time to carefully read the problem statement and ensure you understand what the program needs to do.
- Break down the problem: Break the problem down into smaller, manageable tasks that Who can solve individually. Identify the main tasks that need to be performed and any sub-tasks that will be required.
- Sketch out the solution: Using pen and paper, sketch the solution using flowcharts or pseudocode. It will help you visualize the logic of the program and ensure that it meets the requirements of the problem statement.
- Identify the critical data structures and algorithms: Identify the data structures and algorithms used to solve the problem. It's essential to choose the appropriate data structures and algorithms for the problem, as this can significantly affect the performance and efficiency of the program.
- Define the control flow: Define the control flow of the program, including any loops, conditions, and branching.
- Identify the inputs and outputs: Identify the inputs and outputs of the program, such as user input, file input/output, and any other data that needs to be passed to or from the program.
- Best practices: While developing the solution, consider best practices such as commenting on your code, using meaningful variable names, and following proper indentation. Additionally, using debugging tools like print statements or a debugger can be very helpful in understanding your code's flow and finding errors.
Write a Clean Code
Best Practices for writing clean and organized Java code include:
- Meaningful and descriptive variable and method names: By giving variables and methods clear, meaningful words, you make it easier for other programmers (and yourself) to understand what the code is doing. For example, instead of using x and y as variable names, you could use width and height to indicate that they represent the dimensions of a rectangle. It is essential because, as your codebase grows, it becomes increasingly more challenging to understand the code with clear and descriptive names. Clear and descriptive names also help in debugging and troubleshooting the code.
- Keep lines of code to a reasonable length: Long lines of code can be challenging to read, especially when you have to scroll horizontally to see the entire line. Keeping lines of code shorter does reading and understanding the code easier. It is essential because it makes the code more readable and easier to maintain. It also helps with debugging and troubleshooting the code.
- Proper indentation and spacing: Indentation and spacing can significantly affect the readability of your code. Using proper indentation, you can see the structure of the code and how different blocks of code relate to each other. Spacing can also help to separate different parts of the code and make it easier to read. It is essential because it makes the code more readable and easier to understand. Proper indentation and spacing also help in debugging and troubleshooting the code.
- Comments: Comments can be used to explain what the code is doing and why it is doing it. Including comments in your code makes it easier for other programmers (and yourself) to understand how the code works and make any necessary changes. It's essential to leave it simple with comments but to include them in key places, such as complex algorithms or where the code might not be immediately apparent. It is essential because it makes the code more readable and understandable and helps debug and troubleshoot the code.
- Object-oriented design principles: Using good object-oriented design principles, such as encapsulation and inheritance, you can create more modular and more accessible to maintain code. Encapsulation allows you to hide the implementation details of a class and expose only the necessary information to other parts of the code. Inheritance enables you to build on existing classes and reuse their functionality, which can significantly reduce the amount of code you need to write. It is essential because it makes the code more maintainable and reusable.
- Breaking up complex code: Complex code can be challenging to understand and maintain. You can make the code more manageable and easier to understand by breaking up complex code into smaller methods or classes. It also makes the code more modular, which makes it easier to test and maintain. It is essential because it makes the code more readable and understandable and helps debug and troubleshoot the code.
- Exception handling: Exception handling is a way to handle unexpected errors in the code. Using proper exception handling, you can prevent the code from crashing and provide a more user-friendly way to handle errors. It also helps to make the code more robust and less likely to fail in production. It is essential because it makes the code more powerful and less prone to errors.
- Avoiding global variables: Global variables can make it difficult to understand how the code works and can lead to unexpected behavior. Instead, use local variables and pass them as parameters to methods when necessary. It is essential because it makes the code more readable and understandable and helps debug and troubleshoot the code.
- Naming conventions: Using a consistent naming convention, such as camelCase variable and PascalCase for the class name, can make the code more readable and easier to understand. It also helps to make the code more consistent, which makes it easier to maintain. It is essential because it makes the code more readable and understandable and helps debug and troubleshoot the code.
- Grouping related code: By grouping related code together, such as all the methods for a class in one section and all the import statements at the top of the file, you can make the code more organized and easier to navigate. It also helps to make the code more modular, which makes it easier to test and maintain. It is essential because it makes the code more readable and understandable and helps debug and troubleshoot the code
Here is an example of clean and well-organized Java code for a simple program that calculates the area of a rectangle:
public class Rectangle {
private double width;
private double height;
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
public double getWidth() {
return width;
}
public double getHeight() {
return height;
}
public void setWidth(double width) {
this.width = width;
}
public void setHeight(double height) {
this.height = height;
}
public double calculateArea() {
return width * height;
}
public static void main(String[] args) {
Rectangle rect = new Rectangle(5, 10);
System.out.println("The area of the rectangle is: " + rect.calculateArea());
}
}
Output
The area of the rectangle is: 50.0
This code defines a Rectangle class with private member variables width and height, as well as getter and setter methods for these variables. The class also has a method calculateArea() which returns the area of the rectangle, and a main() method which creates a new Rectangle object and calculates its area.
In addition to these best practices, it is essential to write test cases to validate the code's correctness and to use code reviews to get feedback from other developers to improve the code quality. The test cases should cover all the functionalities of the code, and it should be able to handle different edge cases and errors. Code review is also a critical practice, where other developers review the code and provide feedback on the code quality, readability, and maintainability. By following these best practices and utilizing code review, you can ensure that your code is clean, well-organized, and easy to understand and maintain.
Conclusion
Developing programming logic in Java involves understanding the basics of the Java programming language, as well as its syntax, control structures, and object-oriented programming concepts.
To begin with, you should be familiar with the basic elements of the Java syntax, including variables, operators, loops, and conditionals. Understanding how to use control structures such as if-else statements, for and while loops, and switch statements are essential for developing programming logic in Java.
Object-oriented programming (OOP) is an integral part of Java, and understanding concepts such as classes, objects, inheritance, polymorphism, and encapsulation are necessary for developing robust and maintainable code.
You will also need to be familiar with the development environment, such as Eclipse or IntelliJ IDEA, and have a strong understanding of algorithms and data structures, as well as problem-solving and debugging skills.
Practicing coding, reading code, and understanding how to use Java's built-in libraries and frameworks will also help develop programming logic. For example, the Java Standard Library provides classes and methods for everyday tasks such as working with strings, arrays, and collections. Java's built-in libraries for working with databases and web services are also essential for many programming projects.
In summary, developing programming logic in Java requires a solid understanding of the Java language, its syntax, control structures, and OOP concepts, a strong knowledge of algorithms and data structures, problem-solving skills, and the ability to use Java's built-in libraries and frameworks. Additionally, practicing coding, reading code, and debugging skills are essential.