How to remove last character from String in Java
In java, there are predominantly three classes connected with the string. The classes are String, StringBuilder, and StringBuffer class that gives techniques connected with string control. Eliminating the first and last character from the string is likewise an activity that we can perform on the string.
There are four methods for eliminating the last character from a string:
- StringBuffer.deleteCahrAt() Class
- String.substring() Method
- StringUtils.chop() Method
- Regular Expression
StringBuffer class
StringBuffer is a companion class of strings that gives a large part of the usefulness of strings. The string addresses fixed-length, unchanging person groupings while StringBuffer addresses growable and writable person successions. StringBuffer might have characters and substrings embedded in the centre or affixed as far as possible. It will naturally develop to account for such increases and frequently has a larger number of characters preallocated than are really required to permit space for development.
Constructors of StringBuffer class
1. StringBuffer(): It holds space for 16 characters without redistribution
StringBuffer s1 = new StringBuffer();
2. StringBuffer( int size): It acknowledges a whole number contention that expressly sets the size of the cradle.
StringBuffer s2 = new StringBuffer(20);
3. StringBuffer(String str): It acknowledges a string contention that sets the underlying items in the StringBuffer object and stores space for 16 additional characters without redistribution.
StringBuffer s3 = new StringBuffer("Hello !!! World");
Methods of StringBuffer class
append(): Used to add text toward the finish of the current text.
Length (): The length of a StringBuffer can be tracked down by the length( ) strategy
capacity(): All-out distributed limit can be tracked down by the limit( ) strategy
delete(): Erases a grouping of characters from the summoning object delete.
CharAt(): Erases the person at the file determined by loc
ensureCapacity(): Guarantees limit is essentially equivalent to the given least.
Insert (): Guarantee limit is essentially equivalent to the given least.
length(): Returns the length of the string
reverse(): Invert the characters inside a StringBuffer object
replace(): Supplant one bunch of characters with one more set inside a StringBuffer object
The StringBuffer class gives a technique deleteCharAt(). The technique erases a person from the predetermined position. We utilize the technique to eliminate a character from a string in java. It acknowledges a boundary list of type int. The list is the place of a person we need to erase. It returns this object.
Syntax:
public StringBuffer deleteCharAt(int index)
Example
public class RemoveLastCharcterEx
{
public static void main(String args[])
{
String str = "Hello !!! World";
//constructor of StringBuffer class
StringBuffer sb= new StringBuffer(str);
sb.deleteCharAt(sb.length()-1);
//prints the string after deleting the character
System.out.println(sb);
}
}
Output
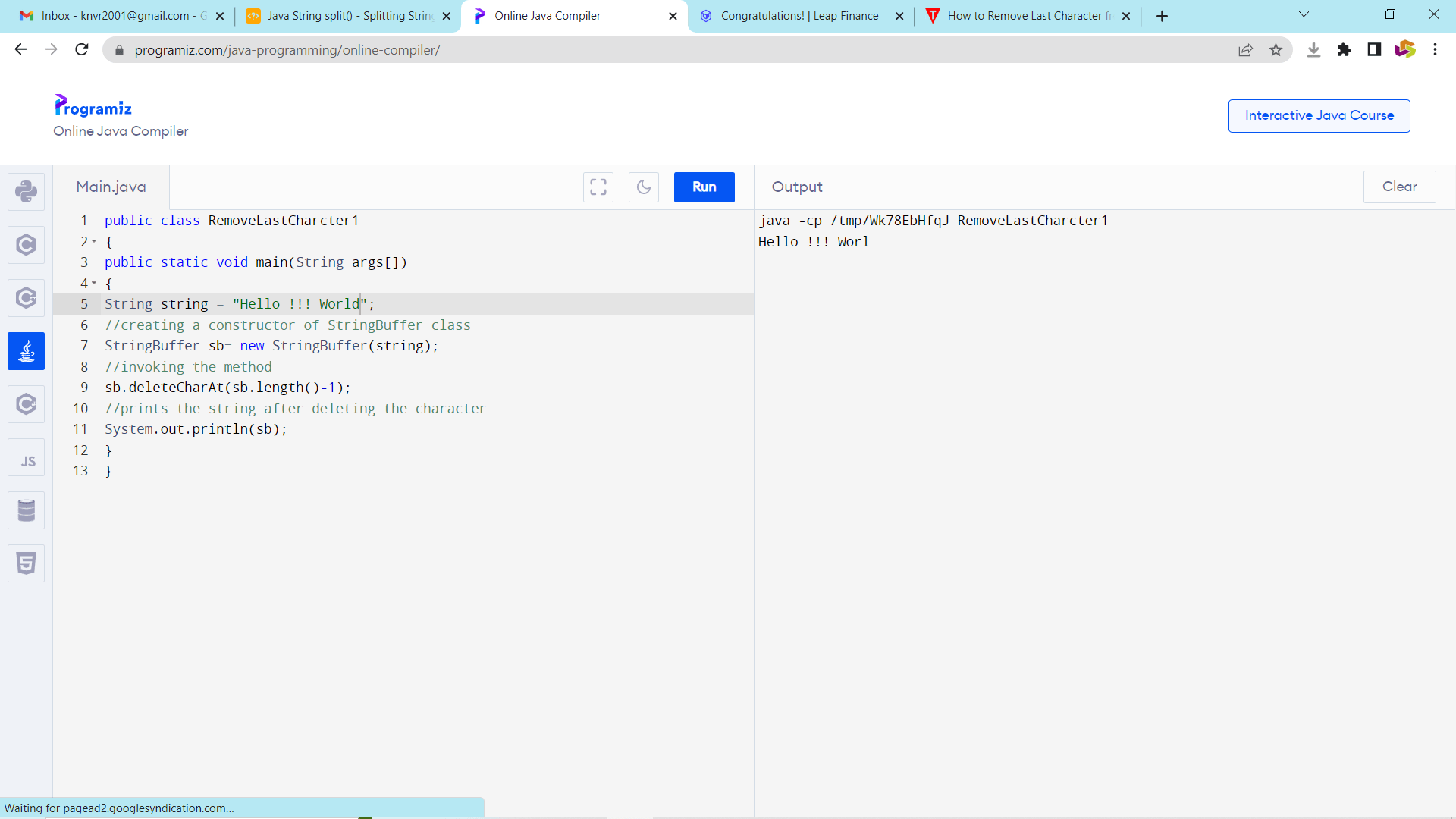
String.substring() Method
The Java String class substring() technique returns a piece of the string. We pass the beginIndex and endIndex number situation in the Java substring strategy where beginIndex is comprehensive, and endIndex is restrictive. All in all, the beginIndex begins from 0, while the endIndex begins from 1.
There are two sorts of substring techniques in Java string.
- public String substring(int startIndex)
- public String substring(int startIndex, int endIndex)
Accepting that the beginIndex is negative or beginIndex > endIndex or the endIndex > length of the string it throws IndexOutOfBoundsException.
The substring() is the technique for the String class. It parses two boundaries, beginIndex and endIndex of type int. It returns another string (sub-string). It isn't strung safely because it doesn't toss a special case assuming the string is invalid or void.
Example
public class RemoveLastCharacterEx
{
public static void main(String[] args)
{
//object of the class
RemoveLastCharacterEx R1 = new RemoveLastCharacterEx();
String str="Hello !!! World";
//method calling
str=R1.removeLastChar(str);
System.out.println(string);
}
private String removeLastChar(String s)
{
// returns the string in the wake of eliminating the last person
return s.substring(0, s.length() - 1);
}
}
Output
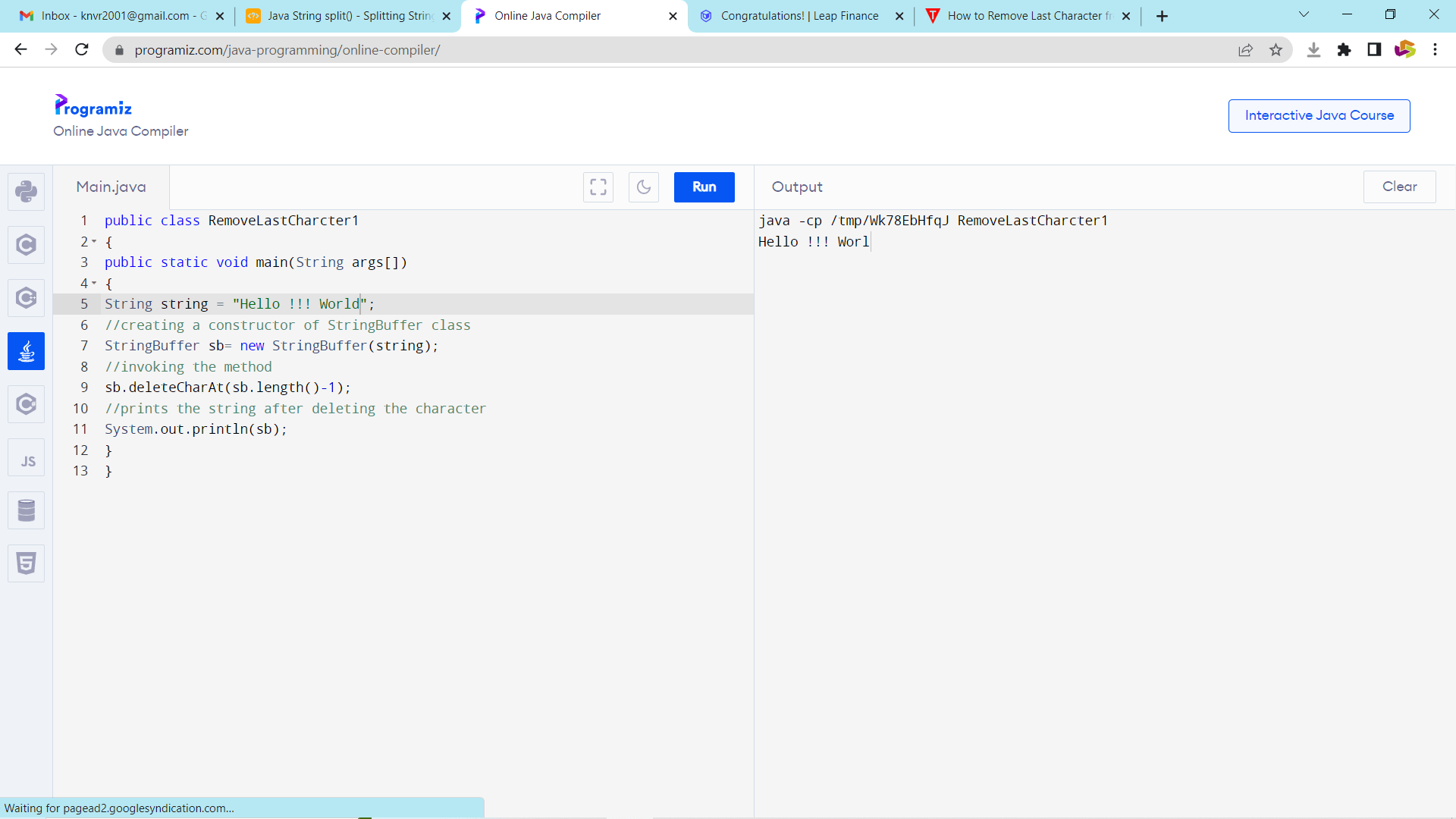
StringUtils.chop() Method
The StringUtils class gives a chop() technique to eliminate the last person from a string. The technique parses a boundary of type String. It likewise acknowledges invalid as a parameter. It returns the string subsequent to eliminating the last person. It likewise returns an invalid string when we input an invalid string.
Syntax:
public static String chop(String str)
For utilizing the chop() technique for the StringUtils class, we want to add the accompanying reliance in the pom.xml document. At the point when we add the Apache lodge lang3 container in the pom document, it downloads the container record and adds the container record to the way. We should import the bundle org.apache.commons.lang3.StringUtils.
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version>
</dependency>
Example
import org.apache.commons.lang3.StringUtils;
public class RemoveLastCharacter3
{
public static void main(String[] args)
{
String string=" Google";
//invoking method
s=StringUtils.chop(string);
System.out.println(s);
}
}
Output
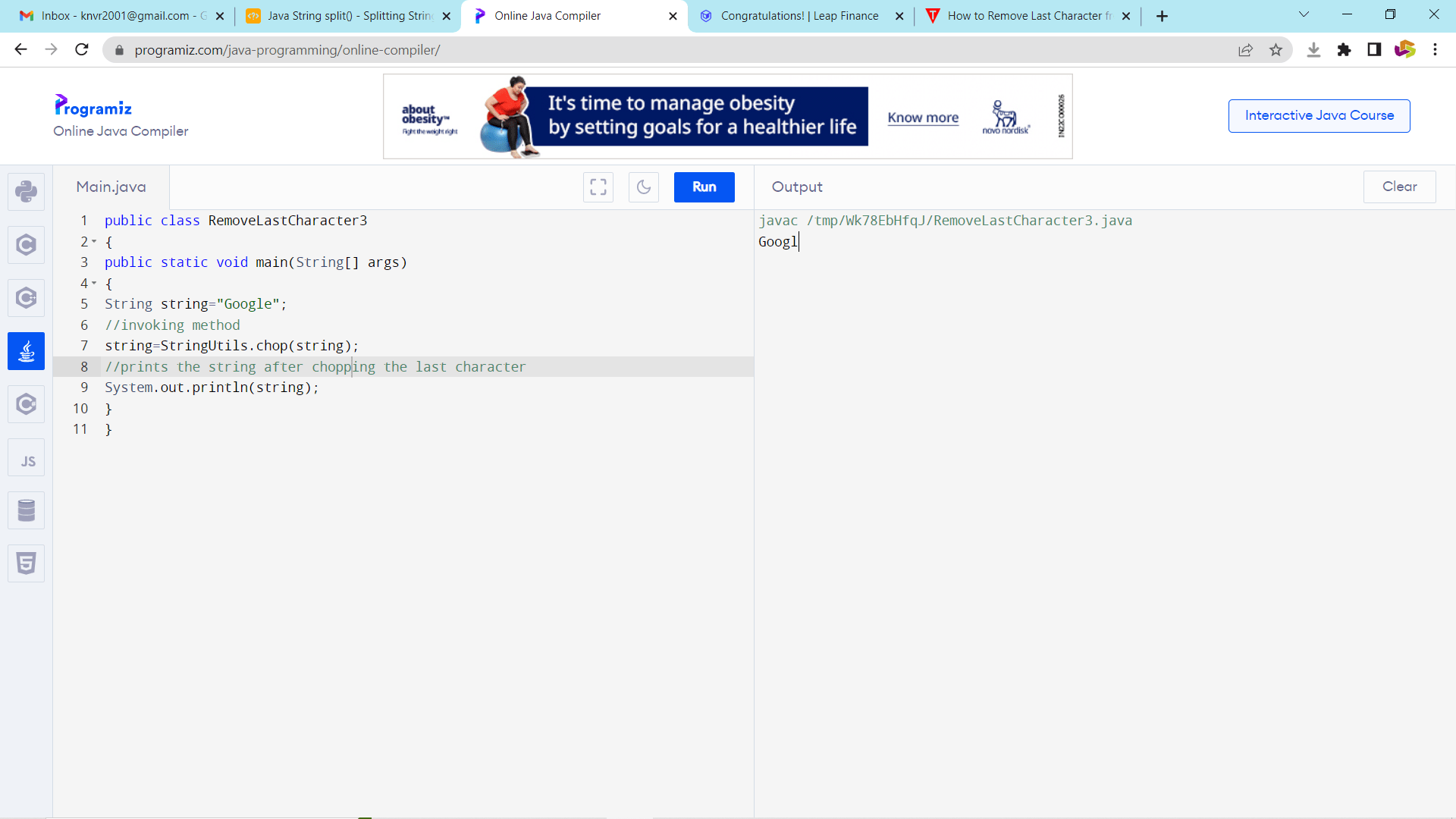
Regular Expression
A regular expression is a succession of characters that frames a hunting design. At the point when you look for information in a text, you can utilize this search example to portray what you are looking for. A regular expression can be a solitary person or a more convoluted design. a Regular expression can be utilized to play out a wide range of text search and text supplant tasks. Java doesn't have an underlying Regular Expression class, yet we can import the java.util.regex bundle to work with standard articulations. The bundle incorporates the accompanying classes:
- Pattern Class - Defines an example (to be utilized in a hunt)
- Matcher Class - Used to look for the example
- PatternSyntaxException Class - Indicates syntax error in a standard articulation design.
We can likewise utilize the regular expression to eliminate or erase the last person from the string. The String class gives the replaceAll() technique that parses two boundaries regex and substitution of type String. The strategy replaces the string with the predefined match.
- Regex: It is the verbalization with which the string is arranged.
- Replacement: It is the exchange string or substitute string for each match.
It returns the subsequent substring.
Syntax:
public String replaceAll(String regex, String replacement)
Example
public class RemoveLastCharEx
{
public static void main(String[] args)
{
//creating an object of the class
RemoveLastCharEx R1=new RemoveLastCharEx ();
String str="Honesty is the best policy";
//method calling
str=R1.removeLastCharacter(string);
//prints the string
System.out.println(str);
}
public String removeLastChar(String str)
{
// the replaceAll() strategy eliminates the string and returns the string return (str == null) ? null : str.replaceAll(".!$", "");
}
}
Output:
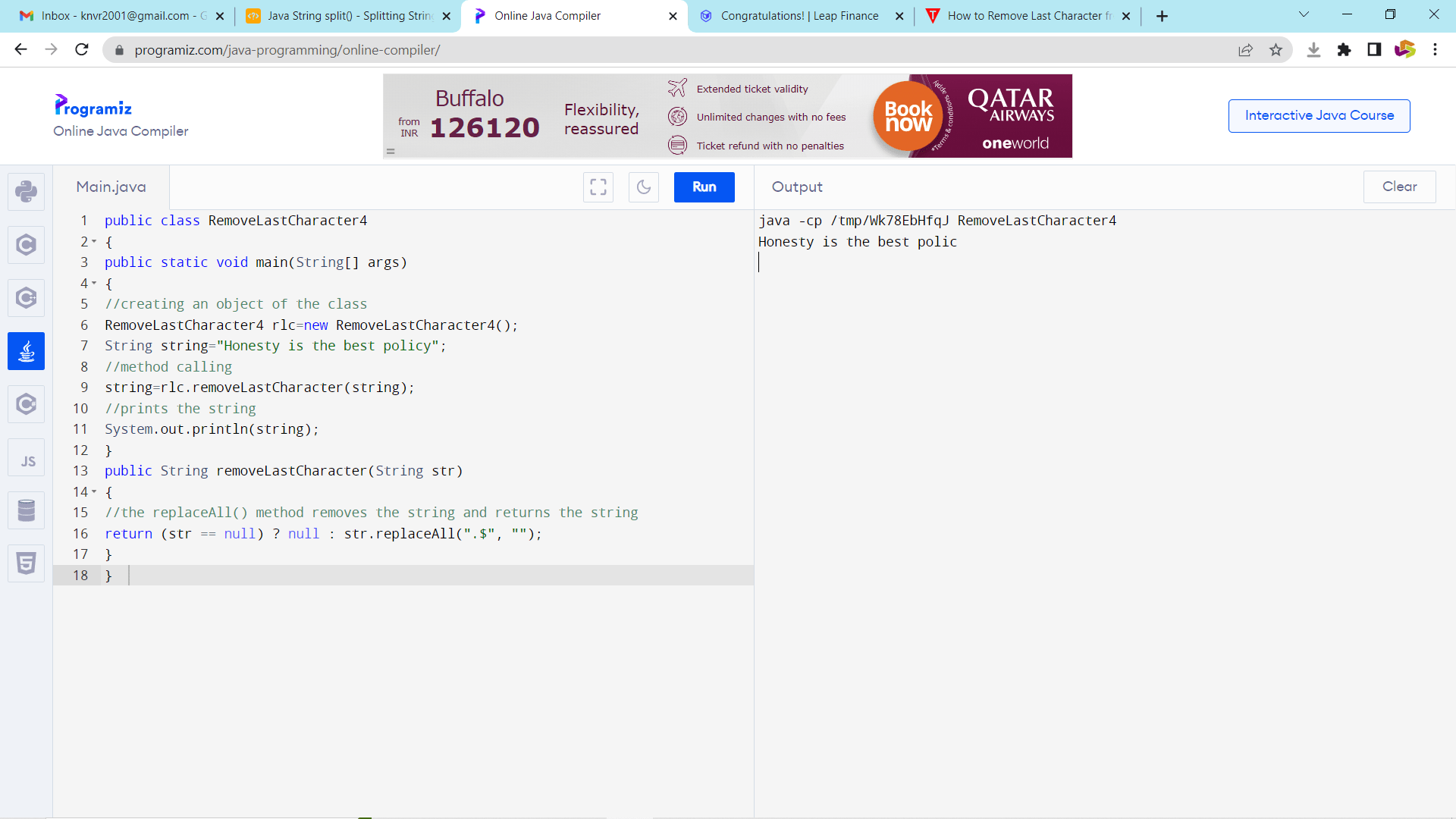