Java Console
If there is a character-based console device connected to the active Java virtual machine, it can be accessed using methods provided by the Java.io.Console class. JDK 6 adds the Console class to the java.io API.
To obtain console input, utilise the Java Console class. It offers ways to read text messages and passwords.
The user won't see the password if you read it using the Console class.
Internally, the system console is connected to the java.io.Console class. Since 1.5, the Console class has been available.
Here is a straightforward example of reading text from a console.
String text=System.console().readLine();
System.out.println("Text is: "+text);
Important Information
- If there is a console, it is used to read from and write to it.
- Because System.in and System.out provide access to the majority of Console's capabilities, it serves mostly as a convenience class. However, when reading strings from the console, its use can make some console interactions simpler.
- Constructors are not provided by Console. Instead, using System.console() returns a Console object, as demonstrated here:
static Console console( )
A reference to the console is returned if one is present. Null is returned if not. In some circumstances, a console won't be accessible. Therefore, no console I/O is possible if null is returned.
- It offers techniques for reading text and passwords. The user won't see the password if you read it using the Console class. Internally, the system console is connected to the java.io.Console class.
Java Console class declaration
Let's see the declaration for Java.io.Console class:
public final class Console extends Object implements Flushable
Java Console class methods
Method | Description |
Reader reader() | It is utilised to retrieve the console's related reader object. |
String readLine() | A single line of text from the console is read using this method. |
String readLine(String fmt, Object... args) | A formatted prompt is given before the console's single line of text is read. |
char[] readPassword() | It can read passwords that are not visible on the console. |
char[] readPassword(String fmt, Object... args) | The formatted prompt is given, and after that, the console reads the password that isn't being displayed. |
Console format(String fmt, Object... args) | A prepared string can be written with it. to the output stream on the terminal. |
Console printf(String format, Object... args) | It is employed to write a string to the output stream of the console. |
PrintWriter writer() | To retrieve the PrintWriter, use this. a piece connected to the console. |
void flush() | The console is flushed using it. |
How to obtain the console's object
The static function console() of the System class returns a single instance of the Console class.
public static Console console(){}
Let's see the code to get the instance of Console class.
Console c1= System .console();
Program for Java Console
import java . io . Console ;
class Read_string {
public static void main ( String args [ ] ) {
Console c1 = System . console ( ) ;
System . out . println ( " Enter your name: " ) ;
String name = c1 . readLine ( ) ;
System . out . println ( " Hello "+ name ) ;
}
}
Output
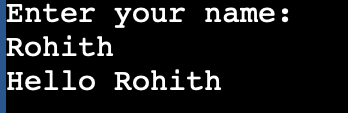
Program for Java Console for reading password
import java . io . Console ;
class {
public static void main ( String args [ ] ) {
Console c1 = System . console ( ) ;
System . out . println ( " Enter password: " ) ;
char [ ] character = c1 . readPassword ( ) ;
String password = String . valueOf ( character ) ;
System . out . println ( " Password is: "+ password ) ;
}
}
Output
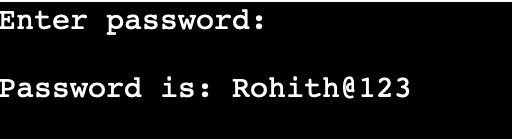