Trimorphic numbers in Java
Wondered what the Trimorphic number is!! In this article, you can learn about what a Trimorphic number is referred to as and how to find whether a number is trimorphic or not using java code.
Trimorphic Number :
A number is said to be a trimorphic number if it ends or terminates with itself when we raise it to a power of 3.
For simple understanding , if you take a number and multiply itself three times, if the resultant number ends with the digits of that number, then it is said to be a trimorphic number .
Consider the following example:
Let n = 75 ,
Raise 75 to the power of 3.
That is , 75 * 75 * 75 which is equal to 4,21,875 . The final resultant number ends with 75. Hence, it is a trimorphic number.
More simply, you can say that if the cube of a number ends with itself, then we can say that a number is a trimorphic number , else it is not a trimorphic number.
Step to find out whether the given number is trimorphic or not :
Step 1: Take a number and raise it to a power of 3 that is multiply itself three times.
Step 2 : Find the number of digits in the given input and store the value to the variable ( “ x ” ) for further use.
Step 3 : Find out the last “x” digits of the cube value , and compare them with the given input.
Step 4: If the given number and the last " x " digits of the computed value match each other, then you can fix that the given number is a trimorphic number .
Trimorph.java
public class TrimorpNum
{
public int findDigiCount(int X)
{
int cDigi = 0;
while(X != 0)
{
X = X / 10;
cDigi = cDigi + 1;
}
return cDigi;
}
public int finPow(int B, int E)
{
int answer = 1;
while(true)
{
if((E % 2) == 1)
{
answer = answer * B;
E = E - 1;
}
if(E == 0)
{
return answer;
}
B = B * B;
E = E / 2;
}
}
public boolean chekTrimorph(int T)
{
// step 1
int Y = T * T * T;
// second step process
int N = findDigiCount(T);
// third step process
int P = finPow(10, N);
// fourth step process
int R = Y - T;
// fifth step process
return (R % P) == 0;
}
public static void main (String args [ ])
{
// object creation for the class TrimorpNum
TrimorpNum object = new TrimorpNum();
for (int i = 1; i <= 10; i++)
{
boolean isTrimorph = object.chekTrimorph(i);
if(isTrimorph)
{
System.out.println(i + " is perfectly a Trimorphic Number.");
}
else
{
System.out.println(i + " is not a Trimorphic Number. Try another .");
}
}
}
}
Output :
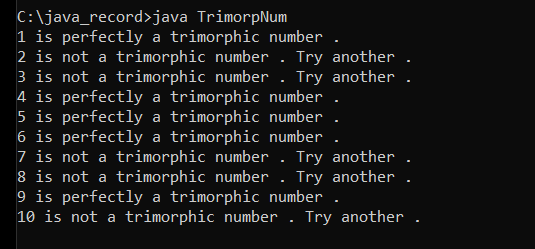
TrimorphNum.java
public class TrimorpNum
{
// a method that checks whether the number T is trimorphic or not
public boolean chekTrimorp (int Tri)
{
int Z = Tri * Tri * Tri ;
String Tri1 = String.valueOf (Tri) ;
String Z1 = String.valueOf (Z) ;
int si1 = Tri1.length ();
int si2 = Z1.length ();
for ( int i = si1 - 1, j = si2 - 1; i >= 0 && j >= 0;)
{
char a = Tri1.charAt(i);
char b = Z1.charAt(j);
if (a != b)
{
return false ;
}
i = i - 1 ;
j = j - 1 ;
}
return true;
}
// main method
public static void main (String args [ ])
{
// creating an object of the class TrimorpNum
TrimorpNum object = new TrimorpNum ();
for ( int i = 1; i <= 10; i++)
{
boolean isTrimorph = object.chekTrimorp (i);
if (isTrimorph)
{
System.out.println ( i + " is perfectly a trimorphic number .");
}
else
{
System.out.println ( i + " is not a trimorphic number . Try another .");
}
}
}
}
Output:
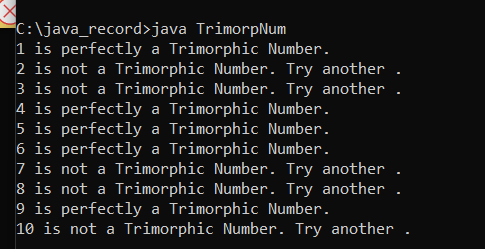