How to Create Different Packages for Different Classes in Java
Packages in Java
In Java, Packages are an assortment of classes, sub-packages, and connection points. i.e. A package addresses a word reference that contains a connected gathering of styles and points of interaction. Whenever we compose an assertion, be it as displayed underneath, we bring in every one of the classes of java.io.* package. Here, java is a catalogue name, and io is another sub-index inside it. What's more, the * addresses every one of the classes and connection points of that io subdirectory.
import java.io.*;
We have many such packages, for instance, java.lang, java. util, and there does exist a lot more inside which there are classes inside the package. Let’s take the most often utilised packages, the 'lang' package for java planning and the 'io' package for input-yield activities.
Allow us to talk about the upsides of packages in java that are as the following:
- Packages help organise related classes to interfaces into a gathering. This assembles every one of the classes and connection points, playing out a similar undertaking in the package. For instance, In Java, every one of the classes and connection points which perform info and result activities are put away in the java.io package.
- Packages conceal the classes and connection points in a different subdirectory.
- A package's classes and points of interaction are segregated from another package's classes and points of interaction.
There are two kinds of packages in java, as recorded:
- Built-in packages
- User-defined packages
Built-in packages:
Packages that accompany JDK or JRD you download are known as built-in packages. The built-in packages have come as Container documents. When we unfasten the Container records, we can undoubtedly see the packages in Container documents, for instance, lang, io, util, SQL, and so on. Java gives different built-in packages to model java.awt.
Examples of Built-in Packages:
- java.sql: Gives the classes to get to and handle information put away in a data set. Classes like Association, DriverManager, PreparedStatement, ResultSet, Explanation, and so forth are essential for this package.
- java.lang: Contains classes and points of interaction that are key to the plan of the Java programming language. Classes like String, StringBuffer, Framework, Math, Number, and so on are essential for this package.
Example:
import java.io.*;
import java.lang.*;
class JL {
public static void main(String args []) {
int p = 10, q = 20;
int s = Math.addExact(a,b);
int m = Math.max(a,b);
double pi = Math.PI;
System.out.printf("Sum = "+s+", Max = "+m+", PI = "+pi);
}
}
Output:
Sum = 30
Max = 20
PI = 3.1415
- java.util: Contains the assortments system, some internationalisation support classes, properties, and an arbitrary number of age classes. Classes like ArrayList, LinkedList, HashMap, Schedule, Date, Time Region, and so on are essential for this package.
Example:
import java.io.*;
import java.util.Arrays;
class JU {
public static void main(String args []) {
int[] Arr = {1, 3, 2, 5, 4};
Arrays.sort(Arr);
System.out.printf("The Sorted array is: %s", Arrays.toString(Arr));
}
}
Output:
The sorted array is: [1, 2, 3, 4, 5]
- java.net: Gives classes to execute organising applications. Classes like Authenticator, HTTP Treat, Attachment, URL, URLConnection, URLEncoder, URLDecoder, and so forth are essential for this package.
- java.io: Gives classes to framework input/yield activities. Classes like BufferedReader, BufferedWriter, Document, InputStream, OutputStream, PrintStream, Serializable, and so on are essential for this package.
Example:
import java.io.Console;
class JI {
public static void main(String args []) {
Console sc = System.console();
System.out.println("Please enter your name : ");
String na = sc.readLine();
System.out.println("Welcome : "+na);
}
}
Output:
Please enter your name:
Vijay
Welcome : Vijay
- java.awt: Contains classes for making UIs and for painting illustrations and pictures. Classes like Button, Variety, Occasion, Textual style, Illustrations, Pictures, and so forth are essential for this package.
Example:
//simple java program using the java awt package
import java.awt.Frame; //here, we are importing the java awt package
import java.awt.Label; //here, we are importing the java awt package
class JA {
//here, we are declaring the constructor
public JA() {
Frame f = new Frame(); //here, we are creating a frame
Label l = new Label(" Welcome to refresh java "); //here, we are creating a // label
f.add(l); //here, we are adding label to the frame
f.setSize(300, 200); //here, we are setting the frame size
f.setVisible(true); //here, we are setting the frame visibility as true
}
public static void main(String args []) {
JA at = new JA();
}
}
Output:
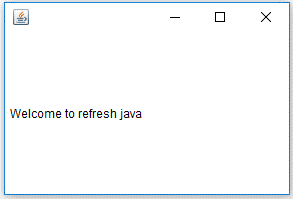
User-defined Packages:
User-defined packages are those packages that are planned or made by the designer to classify classes and packages. They are much like the underlying that java offers. It tends to be brought into different classes and involves equivalent to what we utilise worked in packages. However, If we discard the package explanation, the class names are placed into the default package with no name.
To make a package, we should utilise the package watchword.
Syntax:
package package-name;
Moves toward making User defined Packages.
Stage 1: Making a package in java class. The arrangement is fundamental and straightforward. Compose a package by following its name.
package example1;
Stage 2: Remember the class for the java package Yet recall that the class has one package announcement.
package example1;
class F1 {
public static void main(Strings[] args){
-------capability - - - - - - -
}
}
Stage 3: Presently, the user-defined package is effectively made; we can bring it into different packages and utilise its capabilities.
Syntax:
In creating a package, the package keyword is used
- To create a package, we use the Syntax
package package_name;
- If we want to create a subpackage within a package, we use
package package_name.spname;
- To compile the package, we use
C:\> javac -d . classname.java
- To run the package program
C:\> java packagename.classname
Example:
Stage 1: Characterize a class EmployeeData that holds the accompanying data of an employee:
ID: a string stores the remarkable ID of every employee
Name: It is a string showing the name of the employee.
Note that these fields ought to be proclaimed private.
Stage 2: Make one more class EmployeeDataExtended with a confidential characteristic named area. Fundamentally a string stores the area of the employee.
Stage 3: Presently, in this class, characterise a strategy addDetails() technique that stores the subtleties of understudies; and a strategy printDetails() strategy that yields the subtleties of employees in the arranged request of their id.
Creating the package1:
//simple Java Program that Illustrates the division of Classes into
//the Packages where the Class EmployeeData
// which creates the first package, i.e. pack1
//here, we are importing the package
package pack1;
//here, we are making the main class
public class EmployeeData {
private String id;
private String name;
//here, we are creating method 1
public void addEmployeeData(String id1, String nam)
{
// This keyword refers to the current instance itself
this.id1 = id;
this.nam = name;
}
//here, we are declaring the getter setters’ Methods
//which use the getter methods so that we can
// access the private members for the other packages
public String getId() { return id1; }
public String getName() { return nam; }
}
Creating the package2:
// Java Program that Illustrates the Dividion of Classes into
//the Packages where Class EmployeeDataExtended creates
//the Second package- pack2 and uses the first package
//here, we are importing the packages
package pack2;
//here, we are importing the required classes
// from the pre-defined packages
import java.io.*;
import java.lang.*;
import java.util.*;
import pack1.*;
//here, we are declaring the main class
class EmployeeDataExtended extends EmployeeData {
private String location;
public void addDetails(String id, String name, String location)
{
addEmployeeData(id, name);
// This keyword refers to the current object itself
this.location = location;
}
//here, we are implementing Method 1
public static void
printDetails(TreeMap<String, EmployeeDataExtended> map)
{
//here, we are iterating via for each loop
for (String a : map.keySet()) {
EmployeeDataExtended employee = map.get(a);
//here, we are going to print the ID and the name of the employee
System.out.println(employee.getId() + " " + employee.getName() + " "
+ employee.location);
}
}
//here, we are declaring Method 2
//here, we are declaring the Main driver method
public static void main(String[] args)
{
//here, we display a message for asking input from the user
System.out.print("Enter the number of employees : ");
//here, we are declaring the scanner class to read the user input
Scanner sc = new Scanner(System.in);
int a = sc.nextInt();
String b = sc.nextLine();
int c = 1;
//here, we are creating a TreeMap
TreeMap<String, EmployeeDataExtended> map = new TreeMap<>();
while (a != 0) {
System.out.println("Enter the details of employee " + c + " (id, name, location):");
c++;
String details = sc.nextLine();
String employeeInfo[] = details.split(" ");
EmployeeDE employee = new EmployeeDE();
employee.addDetails(employeeInfo[0], employeeInfo[1], employeeInfo[2]);
map.put(employeeInfo[0], employee);
a--;
}
//here, we are displaying the message
System.out.println("\nThe Employee Details are:");
//here, we are calling the above method 1 to
//this program prints details of the employees
printDetails(map);
}
}
Output:
Enter the number of Employees: 4
Enter the details of Employee 1 (id, name, location):
A101 Vijay Kumar Medak
Enter the details of Employee 2 (id, name, location):
A105 Aslam M.D Gajwel
Enter the details of Employee 3 (id, name, location):
A110 Ajay Kumar Hyderabad
Enter the details of Employee 4 (id, name, location):
A115 Gowtham Kumar Ranga Reddy
The Employee Details are:
A101 Vijay Kumar Medak
A105 Aslam M.D Gajwel
A110 Ajay Kumar Hyderabad
A115 Gowtham Kumar Ranga Reddy