Java Solid Principles
Java implements the object-oriented SOLID principles for the design of software architecture.
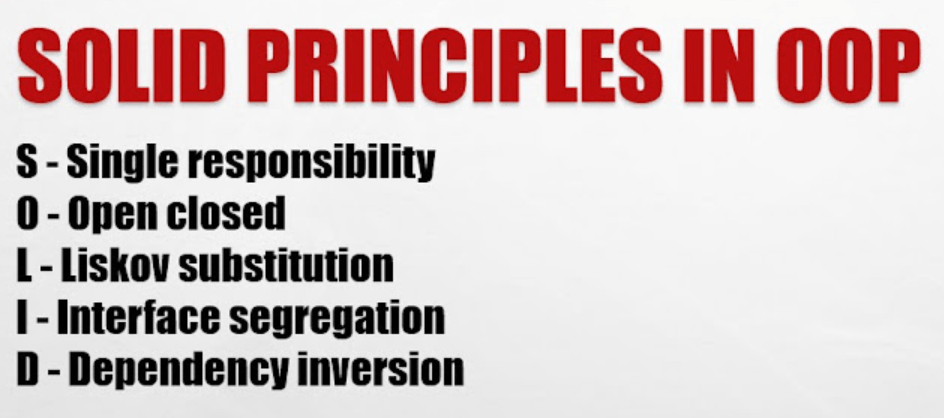
Solid Principles
Java implements the object-oriented SOLID principles for the design of software architecture. Five guiding principles transformed the discipline of object-oriented programming and the process of creating software. It ensures that the software is refactorable, debuggable, and modular.
S: Single responsibility principle
O: Open-closed principle
L: Liskov substitution principle
I: Interface segregation principle
D: Dependency inversion principle
Advantages of Solid Principles
- Code is standardized and easy
- Easy to maintain
- Code can be easily tested
- Code is independent and reusable
- There will be no redundancy in code
Single responsibility principle
The single responsibility principle says that a class should perform only one method or function. It does not imply that the class should not have more functions, but it is recommended to perform one function.
Example single responsibility principle
If you have a class with the name Film that contains three methods like shooting(), Editing() and Release().
Program without using the single responsibility principle
Film.java
class Film
{
public void Shooting()
{
System.out.println(“Shooting method”);
// shotting action
}
public void Editing()
{
// Editing action
System.out.println(“Editing method”);
}
public void Release()
{
// release
System.out.println(“Release method”);
}
Public static void main(String s[])
{
//Main function
}
}
Explanation
In the above sample code, there is a class Film with 3 actions that perform 3 different actions. Having all the functions in one class is making the code complex. So, according to the single responsibility principle, we will separate each function into separate classes.
Program using single responsibility principle
Film.java
class Film
{
public void Shooting()
{
// shotting action
}
public static void main(String s[])
{
// main method
}
}
Edit.java
class Main
{
public void Editing()
{
// Editing action
}
public static void main(String s[])
{
// main method
}
}
Release.java
class Release
{
public void Release()
{
// release function
}
public static void main(String s[])
{
// main method
}
}
Explanation
In the above sample code, all the three functions have 3 separate classes according to the single responsibility principle.
Advantages
- Easy to test and compile
- fewer dependencies
- Code is organized
Open Closed Principle
A class should be designed by this theory so that it completes its purpose successfully without the need for future modification. As a result, it should be feasible to expand the class but it should remain closed to modification.
Methods to do Open closed principle are
1. Using Inheritance
2. Extending class behavior
Program using the open-closed principle
MultiInherit.java
interface Father
{
float ht=6.2f;
void height();
}
interface Mother
{
float ht=5.8f; // public static final variable
void height(); //public abstract method
}
class Child implements Father, Mother
{
public void height()
{
//child got average height of parents
float ht=(father.ht+mother.ht)/2;
System.out.println("child's height: " +ht);
}
}
class MultiInherit
{
public static void main(String args[])
{
Child c1 = new Child();
c1.height();
}//main
}
Liskov Substitution Principle
The derived classes must be fully interchangeable with their source classes when it comes to inheritance. In other words, if class A is a subtype of class B, we should be able to substitute A for B without having the program behave differently.
Program for Liskov Substitution principle
public class Student
{
int height;
int weight;
public void setHeight(double h)
{
height = h;
}
public void setWeight(double w)
{
weight= w;
}
}
public class StudentBMI extends Student
{
public void setHeight(double h)
{
super.setHeight(h);
super.setWeight(w);
}
public void setWeight(double h)
{
super.setHeight(h);
super.setWeight(w);
}
}
Interface Segregation Principle
According to the idea, bigger interfaces break into smaller ones. because the implementation classes only employ the necessary methods. This principle is similar to the single popularity principle.
Example
Syntax:
public interface Vehicle
{
public void car();
public void bus();
public void train();
}
In the above, all three methods are stored in a single interface. By using the Interface Segregation principle we can split the interface methods.
Syntax:
public interface Converion1
{
public void car();
}
public interface Conversion2
{
public void bus();
}
public interface Conversion3
{
public void train();
}
Program for Interface segregation principle
public class Conversion implements IntToDouble,CharToString
{
public void intToDouble()
{
// method
// integer to double
}
public void charToString()
{
//conversion logic
// character to string function
}
}
Dependency inversion principle
It states that the code should depend on abstract details as a substitute for concrete details. We can design software in such a way that various packages or modules are separated from each other by using an abstract layer to bind them together. This principle can be used as classical use in the view of spring framework, All spring frameworks have separate components, these can be worked by injecting dependencies.
Here, Injection is nothing but a process of passing dependencies to a dependency object.
Dependency Injection:
It is a design pattern, that implements the inversion control of dependencies.
It allows programmers to remove Hard-coded dependencies so that the application is made extendable.
Example
Syntax
public class Employee
{
private Details details
public class Employee ()
{
details = new Details ();
}
}
In the above code, the Employee requires the Details object and it is useful to initialize the Details object. If the Details class is changed at any time, then we can also make changes in the Employee class. This makes a tight coupling between the Details class and the Employee class. This problem can be solved by the dependency inversion principle i.e, the Details object will be implemented independently and it is provided to the Employee class.