Sum of digits in string in java
To find the sum of all digits in a string, you need to traverse through the string one by one character; if the character is an integer, you need to add it to the sum variable.
Example
Input: a23tr
Output: 5
Method 1
Algorithm
- Read String.
- Traverse the string till the end of the string using loops.
- Using the isDigit() method, we can check whether the character is a digit or not.
- If isDigit() will return true, then convert it into a number using parseInt().
- Add the digits to the sum variable.
Program
//Program to find the sum of digits in a string
import java. util.*;
import java.io.*;
public class Main
{ public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String s=sc. next();
int sum=0;
for(int i=0; i< s. length() ;i++)
{
char c=s. charAt(i);
if(Character. isDigit(c))
{
int a=Integer.parseInt(String. valueOf(c));
System.out.println("Numbers in the string are "+c);
sum=sum+a;
}//if
}// for loop
System.out.println("sum of digits in string is "+ sum);
}// main
}// class
Output
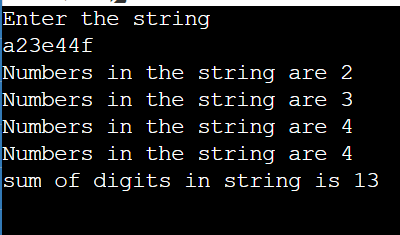
Explanation
This program needs to read the string input using the Scanner class. In order to use the Scanner class, we need to import the util package. After reading the input, we need to traverse through the string, and if there are any digits in the string, we will find them by the isDigit() method, which returns true if it is a number and false if it is not. Then, we will convert the character to a string and add it to the sum variable.
Method 2
Program
import java.util.*;
import java.io.*;
public class Main
{
public static void main(String[] args) {
Scanner sc=new Scanner(System.in);
System.out.println("Enter the string");
String s=sc.next();
int sum=0;
for (int i = 0; i < s.length(); i++) {
if(Character.isDigit(s.charAt(i)))
sum=sum+Character.getNumericValue(s.charAt(i));
}
System.out.println("Sum of all the digit present in String : "+sum);
}
}
Output
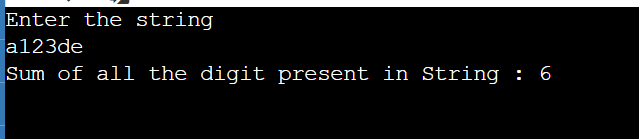
Explanation
In this method, we will use the getNumericValue() method to change the character to an integer. The integer value is added to the sum variable.
Method 3
Program
import java.util.*;
import java.io.*;
public class Main
{
public static void main(String[] args) {
Scanner sc=new Scanner(System.in);
System.out.println("Enter the string");
String s=sc.next();
int sum=0;
for(int i=0;i<s.length();i++)
{
if(s.charAt(i)>='0' && s.charAt(i)<='9')
{
sum+=(s.charAt(i)-'0');
}
}
System.out.println("Sum of all the digit present in String : "+sum);
}
}
Output:
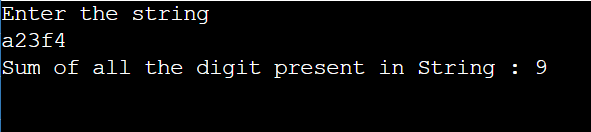
Explanation
In this method, we will subtract the character at the ith position with zero to type and convert it into an integer.