String Declaration in Java
A string is a group of characters. In Java, the string can be treated as both class and datatype. In Java programming, the String class have many advantages. Everything in java can be treated as a string.
Example for string: “ Tutorials”
The strings are enclosed with the double quotes. The String class contains many methods. Strings in Java are available in java.lang package. The declaration of string in Java can be done in two ways:
- By using the string literal
- By using the heap area, i.e. new keyword
1. String literal
In the literal string, the string is created with double quotes.
Example:
String name =" Hello";
In the above example, the string is enclosed with double quotes. Each time you create the string literal, The Java virtual machine(JVM) first checks the “string constant pool”. If the pool already has the string, then it will return the reference from the pool. If the string constant pool doesn’t contain the string, for the created string new instance will be treated and will store
the string. Can be understood with the below example:
String str1 = "Hello";
String str2 =" Hello."
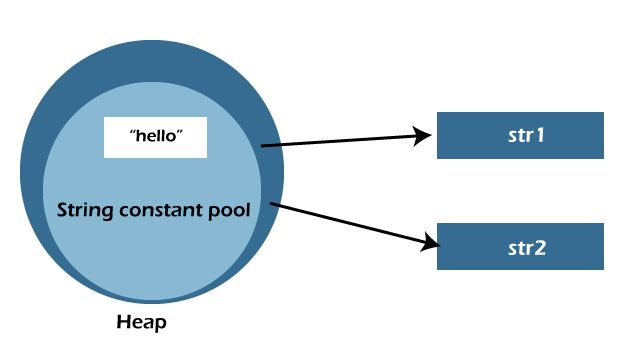
From the above example, the String str2 contains the same string which is declared in str1 will create one object. At the initial, the string object named “hello“ is not found in JVM so it will create an instance for that variable. After the new string named str2 is created with the same value as str1, it will not create a new instance for that variable. So the new instance is not created for the string str2.
An example program for string declaration using the string literal.
StringExample.java
class StringExample {
public static void main(String[] args) {
// create strings
String str1= "Programming";
String str2= "Online";
String str3= "competetions";
// printing the string
System.out.println(str1); //programming will prints
System.out.println(str2); // online will prints
System.out.println(str3); // competetions will prints
}
}
Output

2) By using a new keyword
A new keyword is used to allocate memory in the heap area.
Example:
String str = new String(“hello”);
JVM will create a new object in the heap memory area in this case. In the above example, the literal passed as parameter will store in the constant string pool, and the variable will point to the heap area.
Example 1
StringExampleNew.java
public class StringEx{
public static void main(String args[]){
String str1="Programming";// literal is created
char ch[]={'R','e','c','u','r','s','i','o','n'};
String str2=new String(ch);//character array is to string
String str3=new String("Demo");// Programming string is created by new keyword
System.out.println(str1);
System.out.println(str2);
System.out.println(str3);
}
}
Output:
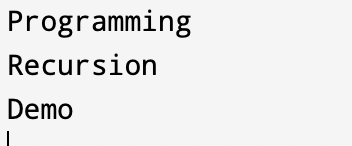
From the above program, the character array is converted to string, and str1,str2, and str3 display the result using the print( ) method.
StringExample.java
class StringExample{
public static void main(String args[])
{
char c[]={'g','o','o','d'};
String str1=new String (c); // new instance in heap area
String str2=new String (str1); // copies the same instance as in str1
System.out.println(str1); // prints str1
System.out.println(str2); // prints str2
}
}
Output

From the above two examples, we can understand how to declare a string using the heap memory area.