Maximum length of string in java
In java, String can act as a data type and a class. The string can be defined as the collection of characters that are enclosed with double quotes(“ “). The string class consists of characters. String can’t be changed if it is created. The objects of the string can’t be changed because they are immutable.
Example :
String s=“Increase”;
String s can be converted into character array as
char ch[]={‘I’,’n’,’c’,’r’,’e’,’ a’,’s’,’e’};
String s =new String(ch);
In the above example, the String class has the length() method, which is used to find the length of the string. For finding the length of the string, there is the length() method.
The length () method returns the length of the string, which is an integer data type. This method counts the number of characters in the string.
Java uses UTF-16 for the representation of the character. Each character occupies two bytes.
The size of int is 4 bytes. The range of integers is -2^31 to 2^31-1. This means (-2147483648 to 2147483647) accepts this range; we can’t be able to store the 2147483647th character. Hence maximum length should be from 0 to 2147483647.
We can find the maximum length using the java program.
Example
import java.util.Arrays;
public class MaximumSize
{
public static void main(String args[])
{
for (int i = 0; i < 1000; i++)
{
try
{
//Integer.max value stores maximum value of the string
char[] array = new char[Integer.MAX_VALUE - i];
//specific value is arranged to each datatype
Arrays.fill(array, 'a');
//Constructor is created for String class ang passing array as an argument
String str = new String(array);
//prints the string length
System.out.println(str.length());
}
catch (Throwable e)
{
// throws a message
System.out.println(e.getMessage());
//returns the maximum value of string
System.out.println("Max: " + (Integer.MAX_VALUE - i));
System.out.println("Max: " + i);
}
}
}
}
Output
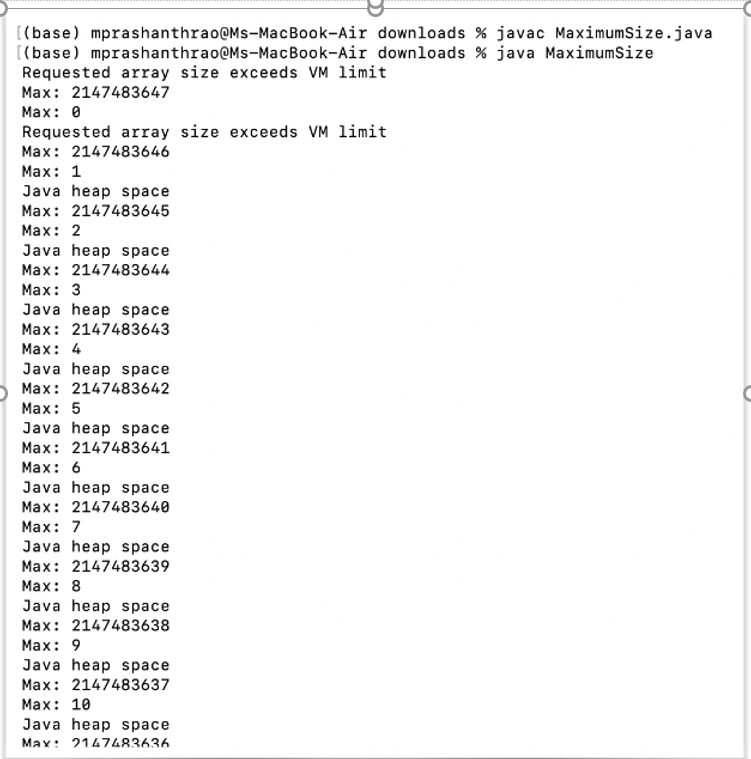
As the output, for example, users can run 1000 times. In the exception occurring block
An array of integers.MAX_VAlue-i. After that, we used the fill method to use the Array class.
The fill method assigns a particular data type value for each array element.
In the example, the program catch block is used to throw an exception caught in the try block. The getMessage() method in the catch block throws an exception.
Each character in Java stores two bytes of memory because strings can be stored as UTF-16 codes. We need twice the memory for storing old and new strings to add strings.
If we insert the strings more than their size, the memory will overflow, and we get a negative result. Consider an example for extending the string beyond the limit.
//Program for beyond the size
public class SizeBeyondLimit
{
public static void main(String[] arg)
{
try
{
System.out.println( "Trying to initialize" + " a n with value" + " Integer.MAX_VALUE + 1");
// to store maximum integer value
int n = Integer.MAX_VALUE + 1;
// preturn the result as negative num as size exceeded
System.out.println("n = " + n);
}
catch(Exception e) //used for handling the exception
{
System.out.println(e);
}
}
}
Output

From the output of the above program, we can see that it was printed as a negative integer.
Because of exceeding the actual size of the String.